Strict mode Vs Non Strict Mode in JavaScript (Comparation)
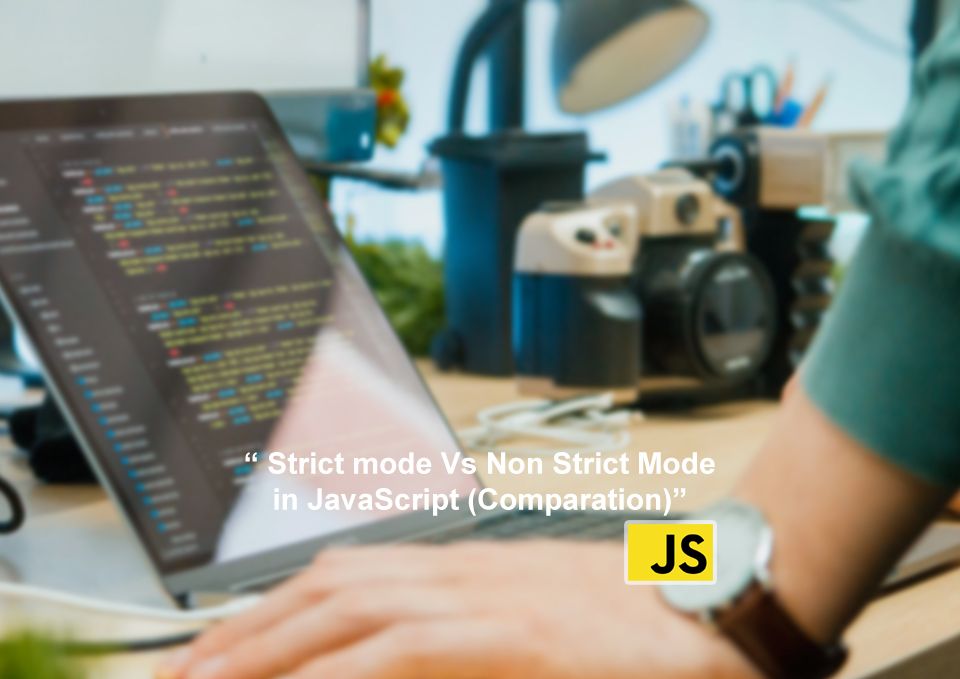
As a web developer, you want to ensure that your code is safe, efficient and error-free. This is where strict and non-strict modes come in. These two modes in JavaScript determine how the code runs and the level of security it provides. In this guide, we'll explore the differences Differences between strict and non-strict modes to help you understand when to use each mode to enhance your web applications.
Strict Mode
As the name suggests, strict mode enforces strict rules in your code, making it safer and less error-prone. It's like having a personal coding coach who can help you avoid common mistakes and make sure your code runs smoothly. For example in strict mode you cannot use undeclared variables This can cause unexpected results in your code. Strict mode will throw an error if you try to override important values ββin global objects to keep your code safe.
Non-Strict Mode
Non-strict mode, on the other hand, provides more flexibility to your code, allowing more relaxed syntax and rules. It's like having a laid-back coding friend who's always there to support you, even when you need to maintain backwards compatibility with older browsers. In non-strict mode you Undeclared variables can be used, and the JavaScript engine will create a global variable with the same name. This pattern makes it easier to write code that works across different platforms, but can also make it harder to debug your code.
Use Strict Mode for Better Code Quality
Consider the following code, which uses a variable that hasn't been declared:
x = 10;
console.log(x);
If you run this code in non-strict mode, it will log 10 to the console, but if you run it in strict mode, it will throw an error. By catching this bug early, you can avoid potential bugs and improve code quality.
Strict Mode for More Secure Code
Now consider the following code, which overwrites a value in the global object:
'use strict';
NaN = 'not a number';
console.log(NaN);
If you run this code in non-strict mode, it will log not a number
on the console, but if you run it in strict mode, it will throw an error. This helps you avoid unintended security risks and ensures your code remains secure.
Use Non-Strict Mode for Backward Compatibility
Suppose you have a codebase that has been around for a long time and you want to ensure that it continues to run seamlessly in modern JavaScript environments. In this case, you can use Non-Strict Mode to make sure that the code runs without any issues.
For example:
<script>
// The code will run in Non-Strict Mode
</script>
Non-Strict Mode for More Flexible Code
If you are writing code that needs to interact with different libraries or frameworks, Non-Strict Mode can provide you with more flexibility in terms of enforcing code rules.
For example:
<script>
"use strict";
function myFunction() {
// Your code here
}
// The code inside myFunction will run in Strict Mode
</script>
Comparison of using strict mode and non-strict mode
For Example :
// Non-Strict Mode
var person = {};
person.name = "John Doe";
// Strict Mode
"use strict";
var person = {};
Object.defineProperty(person, "name", {
value: "John Doe",
writable: true,
enumerable: true,
configurable: true
});
In the non-strict mode example, we just assigned the property name to the object person. However, in the strict mode example, we use the use strict directive and define the property name using the Object.defineProperty()
method. This ensures that properties can only be modified and deleted via this setting method and prevent any accidental changes to the object.
This example shows how strict mode
can enforce better, safer coding practices, which can ultimately improve the performance of web applications. It also highlights one of the main differences between strict
and non-strict
mode, which is to provide a higher level of control and security via strict mode.
When to Choose Strict or Non-Strict Mode
Let's first discuss the considerations for choosing stric
t mode. If you want your code to adhere to stricter standards, then strict
mode is for you. This mode enforces a stricter syntax, making it harder to write code that could cause bugs or security issues. Extra strict
mode will give you safer code because it will throw an error if you try to use some risky functionality (such as accessing global variables without declaring them).
Let's take a look at the precautions for choosing the Non-Strict
mode. If you're using an older browser, non-strict
mode may be a better choice. This is because Non-Strict
mode is more flexible and compatible with a wider range of browsers. Alternatively non-strict
mode can be This is beneficial if your code requires more flexibility, as it allows you to use certain features that would cause errors in strict mode.
So which mode should you choose?
The answer ultimately depends on your specific web application and its needs. If you're using an older browser, non-strict
mode is the way to go. However, if you are looking for safer and stricter code, strict
mode is a better choice.
Let's take a look at an example other comparison code to see the difference between Strict
and Non-Strict
mode in a real-life web app.
// Non-Strict Mode
function example() {
x = 10; // this will not throw an error
}
example();
console.log(x); // 10
// Strict Mode
function example() {
'use strict';
x = 10; // this will throw an error because x is not declared
}
example();
console.log(x); // Uncaught ReferenceError: x is not defined
In this example, you can see that Strict mode throws an error because x is not declared, while Non-Strict mode does not. This highlights the difference in syntax and functionality between the two modes.
Summary
In summary, understanding the difference between strict and non-strict mode in JavaScript is crucial to developing effective and efficient web applications. Strict mode is great for those looking for a more strict and secure codebase, while non-strict mode is great for those who need Compatibility with older browsers, or the need for more flexibility in the code.
At the end of the day, the choice between strict and non-strict mode is all about balance. Choosing the right pattern depends on the specific requirements of your web application and your goals for its development. It is important to remember that while strict mode can improve quality and Code Security It can also make it more challenging to use and may not be suitable for all projects.
Therefore, make an informed decision when choosing strict or non-strict mode. Consider the main differences between the two patterns, your web application's specific goals, and your project's unique needs. By doing this, you will be well able to create a web application that meets all your needs expect.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Strict mode Vs Non Strict Mode in JavaScript (Comparation)