Next.js 14 Adding Another Route in the File System
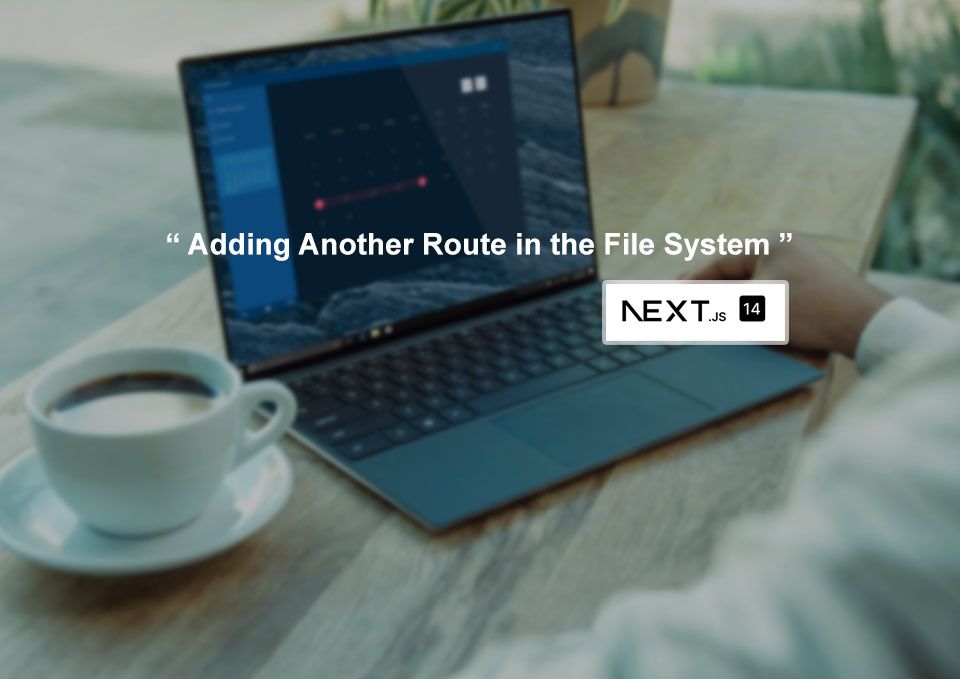
As a software developers, the way we organize our files and folders can make all the difference in the world. A well-structured file system is the cornerstone of maintainable, scalable, and productive code. When our codebase is a tangled web of disorganization, even the simplest tasks can become frustrating treasure hunts.
On the other hand, a logical and intuitive file system allows us to navigate our projects with ease, debug efficiently, and seamlessly add new features. It's a reflection of our collective vision, enabling smooth collaboration and a sense of pride in our work.
In this tutorial, we'll dive into the art of adding a new route to your file system. You'll learn the necessary steps and best practices to keep your codebase organized and your development workflow streamlined. By the end of this journey, you'll not only possess the technical skills to manage your file system, but also a deeper appreciation for its pivotal role in software success.
So, let's unlock the true power of a well-structured file system and elevate your coding prowess to new heights!
Prerequisites : Laying the Groundwork for File System Mastery
Before we dive into adding new routes, it's important to ensure you have a solid foundation in place. This means being familiar with the basic building blocks of file systems - directories, files, and paths. If you're new to these concepts, don't worry - there are plenty of online resources to get you up to speed.
Additionally, you'll want to have a good understanding of your development environment, including the operating system, text editor, and command line tools you'll be using. Mastering these tools will empower you to navigate and manage your file system with confidence.
Finally, a basic knowledge of web development concepts, particularly routing, will be crucial. After all, the new routes you add will serve as the pathways for your application's functionality. Understanding HTTP requests, responses, and common routing patterns will provide a strong foundation to build upon.
Don't worry if you're not an expert in all of these areas yet. We've curated a list of recommended resources to help fill any gaps and prepare you for the exciting journey ahead. With the right foundation in place, you'll be well on your way to file system mastery.
Setting Up the Environment
Let's imagine we're building a e-commerce platform called "Acme Online Store." Our goal is to create a well-structured file system that will support the growth and maintenance of this project over time.
Step 1: Establish the Project Directory First
let's create a new directory to house our Acme Online Store project. Open your terminal and navigate to the desired location on your local machine. Then, run the following command to create a new directory:
mkdir acme-online-store
Step 2: Set Up the Initial File System Structure
Within the "acme-online-store" directory, we'll create a basic file system structure to get us started. This will include a src
folder for our application code, a public
folder for static assets, and a tests
folder for our unit and integration tests.
cd acme-online-store
mkdir src public tests
Now, let's take a moment to consider how we might want to organize the files and folders within each of these top-level directories. For example, in the src
folder, we could have sub-folders for our main application components, such as pages
, components
, services
and utils
.
cd src
mkdir pages components services utils
This separation of concerns will help us keep our codebase clean, modular, and easy to navigate as the project grows.
Step 3: Establish Naming Conventions
Consistent and descriptive naming conventions are crucial for maintaining a well-structured file system. Let's agree on a set of guidelines for our Acme Online Store project:
- Use camelCase for file and folder names (e.g., "productPage.js", "shoppingCart")
- Capitalize the first letter of each major component (e.g., "HomePage.js", "CartService.js")
- Avoid using generic names like "utils" or "components" for individual files
- Ensure that file and folder names clearly reflect their purpose (e.g., "productDetails.js", "checkoutProcess")
By following these conventions, we'll create a file system that's intuitive and easy to navigate, even as the project grows in complexity.
Step 4: Leverage Visualization Tools
As our Acme Online Store file system expands, it will be helpful to have tools that can provide a visual representation of the directory structure. This will make it easier to identify any areas that need optimization or reorganization.
One tool we could use is the "tree" command in the terminal, which will generate a visual directory tree. Alternatively, we could explore file explorer applications or directory tree viewer software that can offer a more interactive view of our file system.
cd acme-online-store
tree
By following these steps, we've established a solid foundation for the Acme Online Store file system. As we continue to develop the application, we can further refine and optimize the structure, ensuring that our codebase remains a source of pride and joy for the entire development team.
Remember, a well-organized file system is not just a technical necessity - it's a reflection of your commitment to writing clean, maintainable, and enjoyable software. So, let's embrace the power of file system mastery and watch our Acme Online Store project thrive!
Adding a New Route to Your Web Application
Step-by-Step Guide: Adding the "Seller Dashboard" RouteAdding the "Seller Dashboard" Route.
Create the "Seller Dashboard" Route
Within the "src" directory of your Acme Marketplace file system, let's create a new folder called "seller-dashboard" to house the "Seller Dashboard" route.
cd src
mkdir seller-dashboard
Inside the "seller-dashboard" folder, we'll add a new file, "index.js," which will contain the code responsible for rendering the "Seller Dashboard" page.
cd seller-dashboard
touch index.js
In the "index.js" file, you'll need to define the route's URL path. For the "Seller Dashboard," we'll use the "/seller-dashboard" URL.
// index.js
export default function SellerDashboard() {
return (
<div>
<h1>Seller Dashboard</h1>
<p>
Manage your product listings, orders, and more in the Seller Dashboard.
</p>
{/* Add more content and functionality as needed */}
</div>
);
}
Integrate the New Route
With the "Seller Dashboard" route file in place, it's time to integrate it into the overall file system structure and routing configuration of your Acme Marketplace application.
Depending on the specific framework or routing library you're using (e.g., React Router, Vue Router), the integration process may vary. However, the general steps typically involve:
- Updating a central routing configuration file to include the new "/seller-dashboard" route and associate it with the "SellerDashboard" component.
- Ensuring that the new route is properly linked or referenced in other parts of your application, such as the navigation menu or breadcrumbs.
- Testing the new route to verify that it's functioning as expected and seamlessly integrating with the rest of your website.
// routing-config.js
import SellerDashboard from './seller-dashboard/index.js';
const routes = [
// Other routes...
{
path: '/seller-dashboard',
component: SellerDashboard,
},
];
Discuss Common Patterns and Conventions
As you continue to expand the Acme Marketplace file system, it's important to consider common patterns and conventions for organizing routes. This will help maintain a clean, scalable, and intuitive structure.
Some best practices to keep in mind:
- Separate routes by feature or functionality (e.g., "products," "orders," "customers")
- Use nested directories for related routes (e.g., "products/list," "products/create")
- Employ consistent naming conventions for files and directories (e.g., camelCase, Pascal case)
- Consider creating a dedicated "routes" or "pages" directory to centralize route management
By following these steps and best practices, you'll have successfully added the "Seller Dashboard" route to the Acme Marketplace file system, creating a cohesive and organized structure that will serve as a solid foundation for your platform's continued growth and development.