Next.js 14: File-based Routing and React Server Components
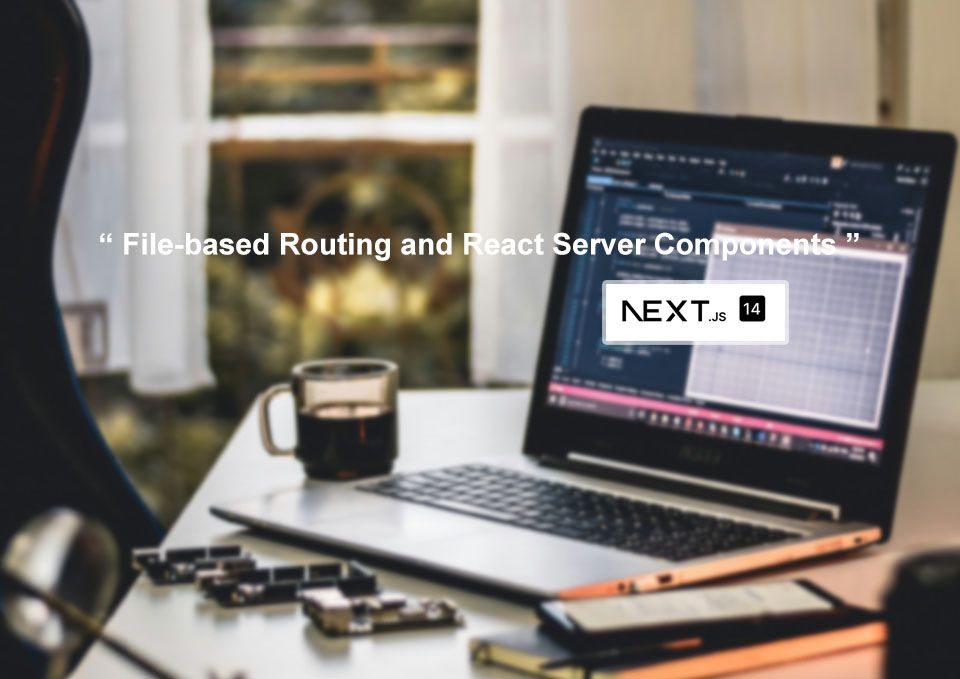
The world of web development is constantly evolving, and the release of Next.js 14 has brought about two significant features that are poised to transform the way we build modern web applications: file-based routing and React Server Components.
File-based Routing: Simplifying the Path to Scalable Applications
One of the key improvements in Next.js 14 is the introduction of file-based routing. This feature allows developers to define their application's structure and navigation by simply creating files and folders within the
directory of their project. This intuitive approach eliminates the need for complex, manually-configured routing setups, making it easier to manage and scale applications as they grow in complexity.pages/
With file-based routing, developers can quickly create new pages, nested routes, and dynamic routes without having to worry about the underlying routing logic. This streamlined approach not only saves time and effort, but also promotes better code organization, making it easier for other developers to understand and maintain the codebase.
React Server Components: Enhancing Performance and User Experience
Another exciting feature introduced in Next.js 14 is React Server Components. This technology allows developers to leverage the server-side rendering capabilities of React, resulting in improved performance and a more responsive user experience.
With React Server Components, the initial rendering of a component is done on the server, which can significantly reduce the initial load times for your web application. This is particularly beneficial for pages that require a large amount of data or complex rendering logic, as the server can handle these tasks more efficiently than the client.
Once the initial page load is complete, React Server Components can then seamlessly transition to client-side rendering, providing a smooth and interactive experience for users. This hybrid approach combines the benefits of server-side and client-side rendering, delivering the best of both worlds.
Understanding File-based Routing
At its core, file-based routing in Next.js 14 allows you to define your application's pages and routes by simply creating files and folders within the pages/
directory of your project. This intuitive approach eliminates the need for complex, manually-configured routing setups, making it easier to manage and scale your application as it grows in complexity.
The way it works is straightforward: each file you create in the pages/
directory corresponds to a unique route in your application. For example, if you create a file named about.js
, it will automatically be accessible at the /about
route. This simplicity extends to nested routes as well, where you can create subfolders to represent nested pages.
Exploring Different Route Types
With file-based routing in Next.js 14, you can create a variety of route types to suit your application's needs:
1. Pages: These are the basic building blocks of your application, representing individual pages or views.
2. Nested Routes: You can create subdirectories within the pages/
folder to represent nested routes, allowing for a more organized and hierarchical application structure.
3. Dynamic Routes: Next.js 14 also supports dynamic routes, where the route path can contain parameters. This is particularly useful for creating pages that display content based on user input or unique identifiers.
By leveraging these different route types, you can easily build complex and dynamic web applications without the need for manual routing configurations.
Benefits of File-based Routing
The introduction of file-based routing in Next.js 14 brings several benefits to the table:
1. Simplified Application Structure: The intuitive file-based approach to routing makes it easier to understand and navigate the codebase, especially as your application grows in complexity.
2. Improved Scalability: As your application expands, adding new pages and routes becomes a straightforward process of creating new files and folders, without the need to maintain a centralized routing configuration.
3. Better Code Organization: File-based routing encourages a modular and organized codebase, as each page or route is self-contained within its own file or directory.
4. Reduced Boilerplate: By eliminating the need for manual routing setup, file-based routing in Next.js 14 saves you time and effort, allowing you to focus more on building the core functionality of your application.
Implementing File-based Routing in Next.js 14
To demonstrate how to set up file-based routing in a Next.js 14 project, let's consider a simple example:
// pages/index.js
export default function Home() {
return (
<div>
<h1>Welcome to the Home Page</h1>
<p>This is the main page of our application.</p>
</div>
);
}
In this example, we have three files:
1. index.js
represents the home page at the root URL (/
).
2. about.js
represents the about page at the /about
route.
3. [id].js
within the products/
directory represents a dynamic route for individual product pages, where the id
parameter is extracted from the URL.
This file-based structure automatically sets up the necessary routes for your Next.js 14 application, making it easy to add new pages, nested routes, and dynamic routes as your project evolves.
// pages/about.js
export default function About() {
return (
<div>
<h1>About Us</h1>
<p>This is the About page of our application.</p>
</div>
);
}
// pages/products/[id].js
import { useRouter } from 'next/router';
export default function ProductPage() {
const router = useRouter();
const { id } = router.query;
return (
<div>
<h1>Product {id}</h1>
<p>This is the page for a specific product.</p>
</div>
);
}
Understanding React Server Components
At the heart of RSC is a fundamental shift in how React components are rendered and delivered to the client. In traditional client-side rendering, the entire component tree is processed and sent to the browser, where it is then hydrated and rendered on the user's device.
React Server Components, on the other hand, offer a more efficient approach. With RSC, the initial rendering of a component is performed on the server, leveraging the server's computational resources to handle the more complex and data-intensive tasks. This server-side rendering process results in a leaner, more optimized HTML payload that is then sent to the client.
The Hybrid Approach in Next.js 14
Next.js 14 takes advantage of this hybrid approach, combining the power of Server Components and traditional Client Components to deliver the best possible user experience.
When a user first visits your Next.js 14 application, the initial page load is handled by the server, where the Server Components are rendered and the resulting HTML is sent to the client. This server-side rendering process can significantly improve the perceived performance of your application, as users can start interacting with the page much faster.
Once the initial page load is complete, the client-side runtime takes over, allowing for a seamless transition to client-side rendering for subsequent interactions. This hybrid approach ensures that your application maintains its responsiveness and interactivity, providing a smooth and engaging user experience.
Benefits of React Server Components
The introduction of React Server Components in Next.js 14 brings several key benefits to the table:
1. Improved Performance: By offloading the initial rendering process to the server, RSC can significantly reduce the initial load times of your web application, leading to a more responsive and engaging user experience.
2. Better Data Management: Server Components are better equipped to handle data-intensive operations, such as API calls and complex computations. This allows you to optimize the data flow and reduce the amount of data that needs to be transferred to the client.
3. Automatic Code Splitting: Next.js 14 with RSC automatically handles code splitting, ensuring that only the necessary code is loaded for each page or component, further enhancing the performance of your application.
4. Improved Developer Experience: RSC encourages a more modular and scalable codebase, as developers can better separate concerns between server-side and client-side logic. This promotes better code organization and maintainability.
Implementing React Server Components in Next.js 14
To demonstrate the implementation of React Server Components in a Next.js 14 project, let's consider a simple example:
// pages/api/data.js (Server Component)
export default async function handler(req, res) {
const data = await fetchDataFromAPI();
res.status(200).json(data);
}
// components/DataDisplay.js (Server Component)
import { use } from 'react';
export default function DataDisplay() {
const data = use(fetchData());
return (
<div>
<h2>Data Display</h2>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
</div>
);
}
// pages/index.js (Client Component)
import DataDisplay from '../components/DataDisplay';
export default function Home() {
return (
<div>
<h1>Welcome to the Home Page</h1>
<DataDisplay />
</div>
);
}
Conclusion
If you're a web developer seeking to stay ahead of the curve, it's time to explore the possibilities of Next.js 14. By embracing the power of file-based routing and React Server Components, you can unlock new levels of efficiency, performance, and creativity in your web development projects.
Dive in, experiment, and discover how Next.js 14 can help you build the web applications of the future. The future is here, and it's powered by the latest advancements in Next.js.