Javascript Fundamentals #Values & Variables
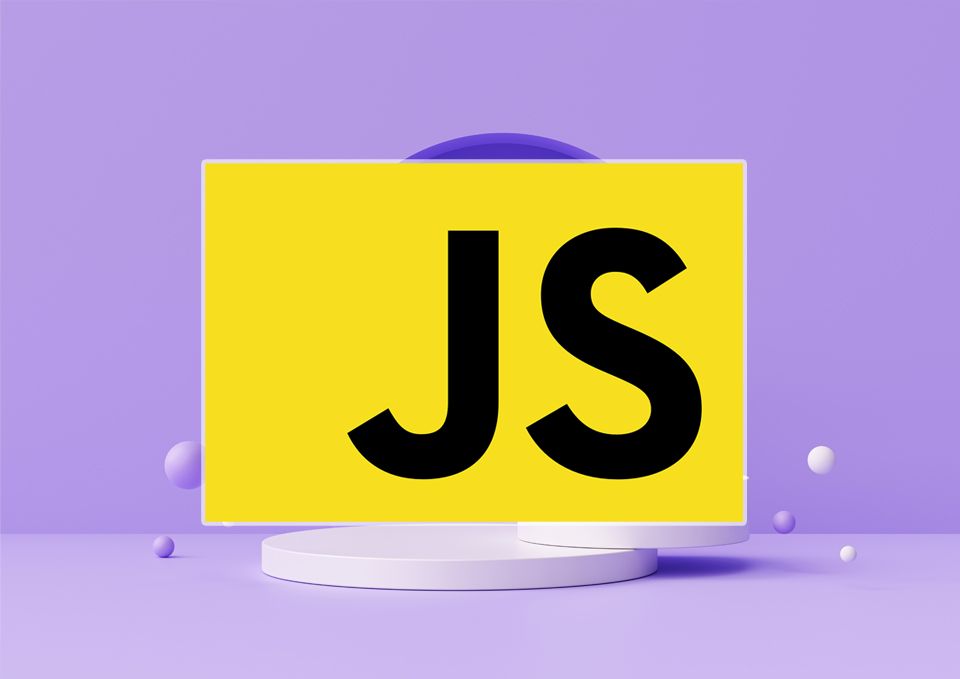
Are you interested in coding with JavaScript? Get started quickly by learning the basics of JavaScript values and variables. With a grasp of these concepts, you'll soon be able to create your own projects and bring your ideas to life.
Aspiring web developers must learn about the relationship between values and variables in JavaScript. Values, like numbers or strings, are data that can be stored and referenced with variables. A variable is a symbolic name for a value, allowing it to be conveniently referenced within a program. Get a better understanding of how values and variables interact with each other in JavaScript by reading this post.
Understanding the Basics of Value in JavaScript
In JavaScript, values are pieces of data that can be used in calculations and for various other functions. These values can come in the form of numbers, strings, Booleans, arrays, and objects. They are essential components of any JavaScript program.
Values are used to represent various types of data. These values can include numerical data (i.e., numbers such as 1, 2, and 3), text and characters (i.e., strings such as “Hello, World!"), true or false values (i.e., booleans such as true or false), groups of data (i.e., arrays such as), and collections of data and functions (i.e., objects such as {name: 'John', age: 25}).
Understanding the Basics of Variables in JavaScript
In JavaScript, variables are used to store and reference values. To declare a variable, you must use the keyword “var”, followed by the name of the variable. For example, if you wanted to declare a variable named “myName” , the code would look like this: "var myName". Once declared, that variable can be used throughout your program.
var myName;
Variable is like a container for holding data values. Variables are assigned values using the = operator; for example, you could assign the value "John" to a variable named myName like this: myName = "John". In addition to storing strings, variables can also hold numbers and Booleans (true or false).
myName = “John”;
Once a variable is assigned a value, it can be referenced by its name at any point in the code. Usage of variables is vital for writing dynamic programs, as they allow you to store different types of data that may change throughout the execution of the program. By simply replacing the variable name with its value, you can print or manipulate data as needed.
console.log(myName); // Outputs “John”
Data Type in Javascript
Using Variables and Values in JavaScript
Variables and values are used as tools in JavaScript to store data and manipulate it. Variables can be used for various uses, for example, storing user-inputted information like phone numbers or addresses. Additionally, variables can be used to store the result of a computation such as adding two numbers together or finding the average of a list of numbers.
Variables and values can be used in JavaScript programs to control the flow of a program. For instance, an if statement can be employed to evaluate if a given variable corresponds to a certain value, as seen in this example:
if (myName = “John”) {
// Do something
}
With JavaScript variables and values, you can create dynamic content. For instance, an array of strings can be used to generate a list of items. An example of this is represented below:
var items = [“Apple”, “Orange”, “Banana”];
for (var i = 0; i < items.length; i++) {
console.log(items[i]);
}
// Outputs “Apple”, “Orange”, “Banana”
Understanding the basics of values and variables in JavaScript is critical for any programmer. Variables are used to store data such as numbers, words, or objects, while values contain the actual data itself. Knowing how to properly declare, assign, and use both variables and values is essential for writing solid JavaScript programs.
Finish.