Understanding JavaScript Template Literals (Template Strings)
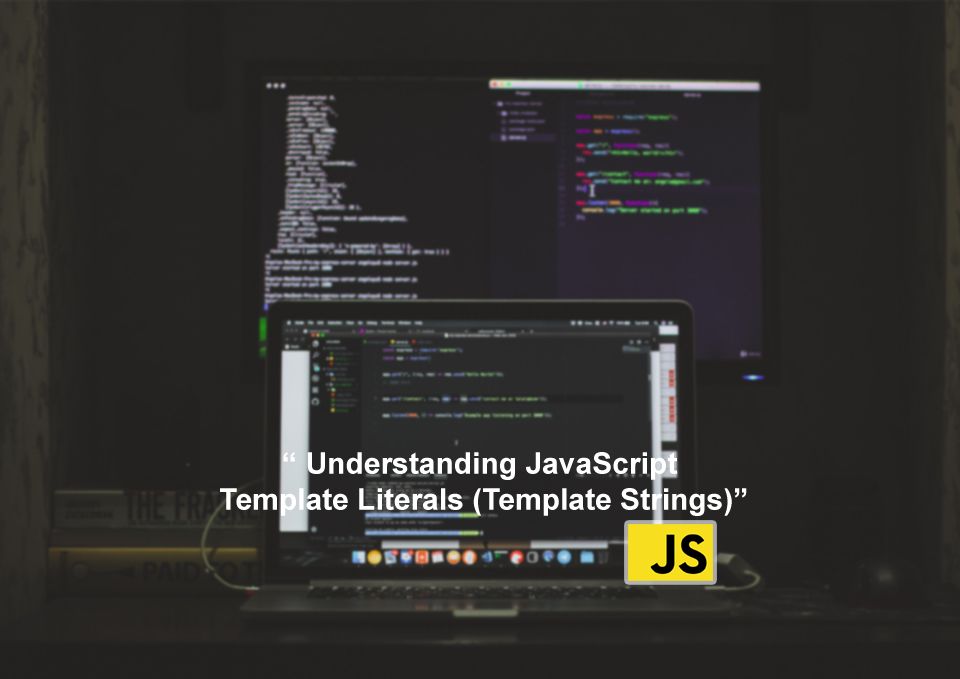
Are you a keen JavaScript programmer looking for an easier way to write your code? Are you tired of using multiple strings just to add a variable or two? Then template literals and string manipulation are the tools you need! In this blog post, we'll be discussing how to use strings and template literals in JavaScript and what advantages they offer.
Template literals (template strings) allow you to use strings or embedded expressions as strings. They are enclosed in backticks
(`).
const name = 'Jack';
console.log(`Hello ${name}!`); // Hello Jack!
Template Literals for Strings
In earlier versions of JavaScript, you would use single quotes or double quotes for strings.
const str1 = 'This is a string';
// cannot use the same quotes
const str2 = 'A "quote" inside a string'; // valid code
const str3 = 'A 'quote' inside a string'; // Error
const str4 = "Another 'quote' inside a string"; // valid code
const str5 = "Another "quote" inside a string"; // Error
To use the same quotes inside a string, you can use escape characters. And now you can use template literals instead of using escape characters.
const str1 = `This is a string`;
const str2 = `This is a string with a 'quote' in it`;
const str3 = `This is a string with a "double quote" in it`;
As you can see, template literals not only make it easy to include quotes, but also make our code look cleaner.
Multiline strings using template literals
Template literals also make it easy to write multiline strings.
// using the + operator
const message1 = 'This is a long message\n' +
'that spans across multiple lines\n' +
'in the code.'
console.log(message1)
Using template literals, you can replace with :
const message1 = `This is a long message
that spans across multiple lines
in the code.`
console.log(message1)
The output of these two programs will be the same.
Expression Interpolation
Before JavaScript ES6, you would use operators +
to concatenate variables and expressions in strings.
const name = 'Jack';
console.log('Hello ' + name); // Hello Jack
With template literals, it's a little easier to include variables and expressions in strings. For this, we use the ${...}
syntax.
const name = 'Jack';
console.log(`Hello ${name}`);
const result = 4 + 5;
// template literals used with expressions
console.log(`The sum of 4 + 5 is ${result}`);
console.log(`${result < 10 ? 'Too low': 'Very high'}`)
The process of assigning variables and expressions within template literals is called interpolation.
Tagged Templates
Normally you would use a function to pass arguments.
function tagExample(strings) {
return strings;
}
// passing argument
const result = tagExample('Hello Jack');
console.log(result);
However, you can create markup templates (which behave like functions) using template literals. You use tags that allow you to parse template literals with functions.
Markup templates are written like function definitions. However, parentheses ()
are not passed when calling the literal.
function tagExample(strings) {
return strings;
}
// creating tagged template
const result = tagExample`Hello Jack`;
console.log(result);
An array of string values ββis passed as the first argument to the marker function. You can also pass values ββand expressions as the remaining parameters.
const name = 'Jack';
const greet = true;
function tagExample(strings, nameValue) {
let str0 = strings[0]; // Hello
let str1 = strings[1]; // , How are you?
if(greet) {
return `${str0}${nameValue}${str1}`;
}
}
// creating tagged literal
// passing argument name
const result = tagExample`Hello ${name}, How are you?`;
console.log(result);
This way you can also pass multiple parameters in the tagged template.