JavaScript Comparison and Logical Operators (Beginner's Guide)
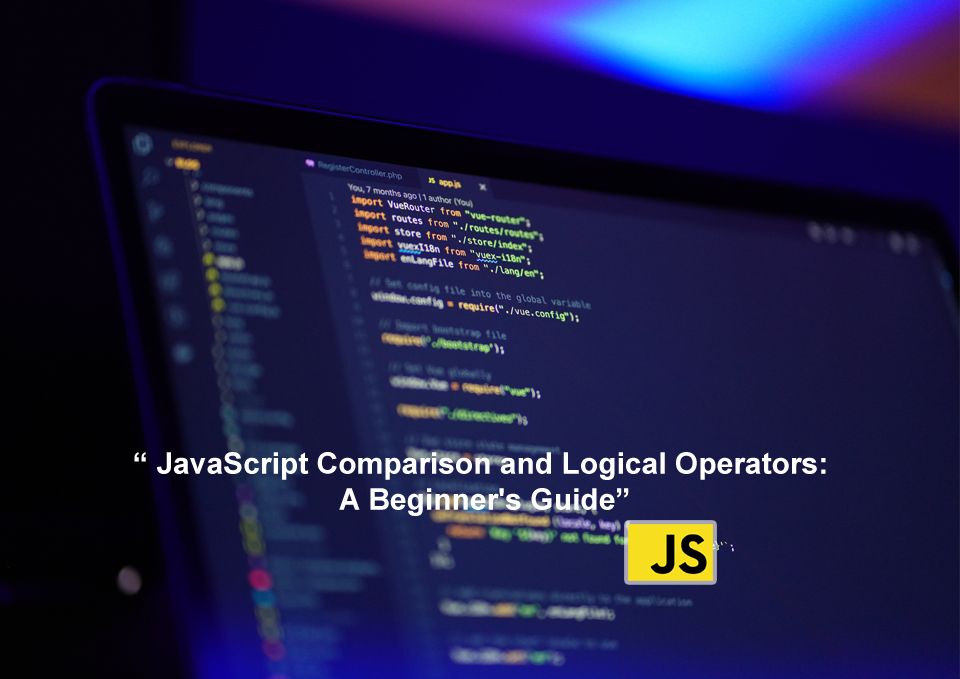
Javascript Logical Operators are an integral part of writing code in Javascript and are used to control the flow of a program. In this article, we will explore the basics of these operators and show how they can be applied in making a web app.
Main Logical Operators in Javascript
There are three main logical operators in Javascript: AND (&&)
, OR (||)
, and NOT (!)
.
- The AND operator returns true if both operands are true, and false if either operand is false.
- The OR operator returns true if either operand is true, and false if both operands are false.
- The NOT operator returns the opposite of a Boolean value, meaning if the value is true, it will return false, and vice versa.
Implementation in web applications :
First: AND (&&)
To demonstrate how these operators can be used in a web app, let's look at a simple example.
if (username !== "" && password !== "") {
// submit form
} else {
// display error message
}
The AND operator is used to check if both the username and password fields are not empty. If both are true, the form will be submitted, and if either one is false, an error message will be displayed.
Second: OR (||)
NOT operator in web applications is to check if a variable is falsy. For example, consider the following code:
Another example of where logical operators can be useful is in controlling the visibility of certain elements on a page.
For example:
if (loggedIn === true || condition === true) {
// show button
} else {
// hide button
}
In this example, the OR operator is used to check if either the user is logged in or the condition is true. If either is true, the button will be shown, and if both are false, it will be hidden.
Third : NOT (!)
NOT operator in web applications is to check if a variable is falsy.
For example, consider the following code:
let userName = "";
if (!userName) {
console.log("Please enter a username");
}
Combines all Operators
It is also worth noting that logical operators can be combined to create more complex expressions.
For example, consider the following code:
let x = 1;
let y = 0;
if ((x === 1 || y === 1) && x !== y) {
// code here
}
In this code, the expression inside the if statement uses both the OR and NOT operators. The OR operator checks if either x or y is equal to 1, while the NOT operator checks if x and y are not equal. Only if both of these conditions are met will the code inside the if statement be executed.
In addition, to control flow and decision-making, logical operators can also be used in bitwise operations. For example, the AND operator can be used to mask bits in a number, while the OR operator can be used to set bits. However, these operations are more advanced and outside the scope of this article.
Conclusion
In conclusion, it is clear that logical operators play a vital role in the Javascript programming language. They are used to make decisions, control the flow of code, and even perform bitwise operations. With their versatility, it is no wonder that logical operators are a fundamental tool for every Javascript developer.
By mastering the use of logical operators, developers can write clearer and more concise code. They can easily make decisions based on certain conditions and control the flow of their code in a way that makes it easier to understand. Additionally, the ability to perform short-circuit evaluation with logical operators can greatly improve the performance of code by skipping over expensive operations.
Summary
In summary, if you are just starting out with Javascript or if you are looking to improve your skills, understanding and mastering the use of logical operators should be one of your top priorities. With their versatility and power, logical operators are an essential tool for writing effective and efficient Javascript code.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)