Equality Operators: == vs. ===
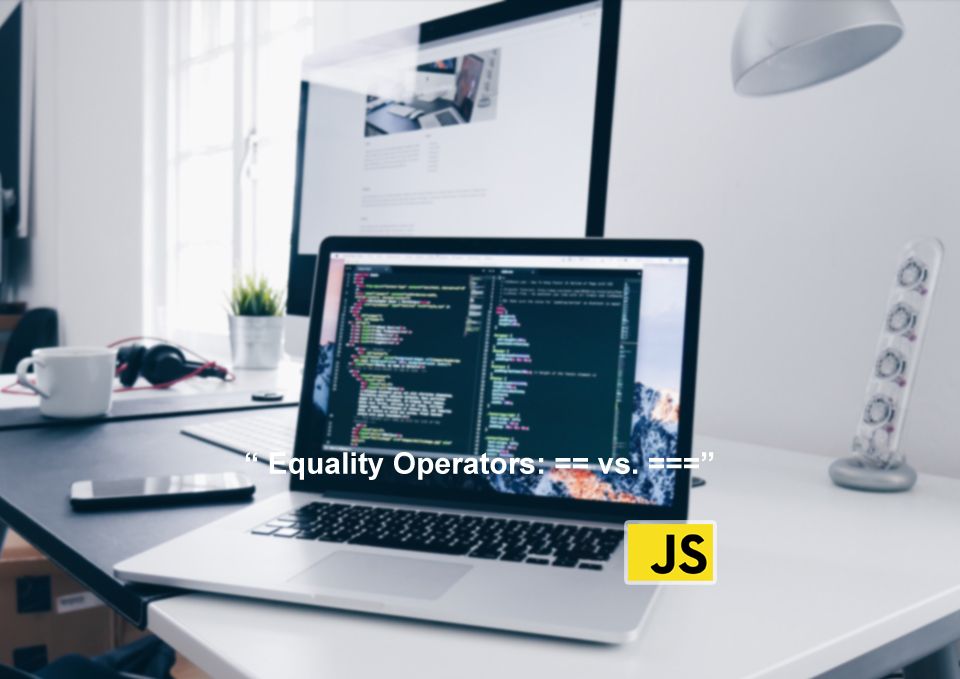
The equality operator in javascript is used to compare two values for equality. Comparisons are performed by ==
and ===
operators in javascript. The main difference between ==
and ===
operators in JavaScript is that the ==
operator performs a type conversion on the operand before comparison, while the ===
operator compares the values and data types of the operands.
Introduction
In our daily life, we come across many situations where we need to compare two things. For example, imagine logging into your Instagram. After visiting the site, you'll see a login page asking for your username and password. Once you submit your details, the site goes through its database and Compare the provided details with the available details. If the details match, it allows you to log in; otherwise it doesn't.
This is one of many instances where two objects are compared and further actions are decided based on the result.
In Javascript, we use comparison operators to compare two values. There is a special case of comparison, where two values are compared and it is determined whether the values are equal (or not). In this case we can use the following two operators in javascript:
==
operator.===
operator.
=
with an exclamation point !
. So the !=
and !==
operators are used to check the inequality of two values respectively.Before understanding the difference between ==
and ===
in Javascript Let us see what the ==
and !==
operators are in javascript.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
What are ==
and !=
in JavaScript?
The ==
and !=
operators in JavaScript are used to compare two values. The ==
operator checks whether two values are equal. The !=
operator checks whether two values are not equal. It is also known as the loose equality operator because it checks for abstract equality, i.e. it tends to convert The data type of the operands for comparison when the data types of the two operands are different.
For equality:
x == y
For inequality:
x != y
where x
and y
are operands and the middle part is the operator. In case of equality comparison, we use ==
operator and in case of inequality comparison we use !=
operator.
Return type: boolean
It returns true
or false
.
The ==
operation if the two operands are of the same data type and have the same value, or if the two operands are of different data types but one of them is convertible to the data type of the other operand and have the same value character returns true. returns false if the two operands have different values .
The !=
operator returns true if the two operands have the same data type and different values, or if they have different data types and neither of them can be compared with the type of the other operand. Returns false if both operands have the same data type and have the same value, or both different data types, but any of them can be converted to the data type of the other operand and have the same value.
==
or !=
operator performs a type conversion on elements before comparing them.What is type conversion?
The ==
and !=
operators loosely compare two operands, i.e. when comparing two operands, if the data types of the two operands are different, the operator tends to convert either of them to the other operation types of numbers, and compare their values.
This is one of the most important differences between ==
and ===
in Javascript
Example:
If we are comparing two values, a string, and another number, then it converts the string value to a number type and then compares the values.
let a = 10;
let b = '10';
console.log(a == b);
//output: true
In the above example, b is converted to a numeric type by the operator and then compared with a. Since a and b have the same numeric value, it outputs true.
Behavior of the ==
and !=
operators when both operands are of the same type:
When the two operands being compared are of the same type, the ==
and !=
operators behave as follows:
- If both are numeric data types, the
==
operator returns true if both have the same value; false otherwise. The!=
operator will do the opposite.
let a = 10;
let b = 10;
let c = -10;
console.log(a==b);
//output: true
console.log(a==c);
//output: false
console.log(b!=c);
//output: true
console.log(a!=b);
//output: false
In the example above, the first output is true because a and b have the same value, and the second output is false because a and c have different signs. Similarly, the third output is true because b and c are different, and the fourth output is true because a and b are the same.
- The
==
operator still outputs false if a number is compared to NaN. - If both operands are of type string, the
==
operator returns true only if every element of the first operand matches every element of the second operand. The!=
operator will do the opposite.
Example:
let str1 = 'Javascript';
let str2 = 'Javascript';
let str3 = 'JavaScript';
console.log(str1 == str2);
//output: true
console.log(str1 == str3);
//output: false
console.log(str1 != str3);
// output: true
In the example above, the first output is true because str1 and str2 are identical, but the second output is false because str1 and str3 are not identical. The third output is true because str1 and str3 are not the same.
So we can conclude that the comparison is also case-sensitive.
- If both operands are of boolean type, the
==
operator returns true only if both operands are true, otherwise, it returns false. The!=
operator will do the opposite.
Example:
bool a = false;
bool b = true;
bool c = true;
bool d = false;
console.log(b == c);
//output: true
console.log(b == a);
//output: false
console.log(a == d);
//output: true
console.log(b != d);
//output: true
console.log(b != c);
//output: false
Behavior of the ==
and !=
operators when the types of the two operands are different:
The ==
and !=
operators use the abstract equality comparison algorithm to compare two operands when the operands are of different types. The algorithm relaxes the check and tries to modify the operands to be of the same type before performing any operations.
Here is the gist of ==
and !=
behavior when the operand types are different:
- If we are comparing strings and numbers, convert the strings to numbers before comparing.
- If we compare a boolean with a number (0 or 1), then it will treat 0 as false and 1 as true.
- If we compare two objects, then the operator will check if both refer to the same object. If yes, the
==
operator will return true and!=
will return false, otherwise==
will return false and!=
will return true. - If we compare null and undefined then
==
operator will return true and!=
operator will return false.
Compare strings
and numbers
10 == '10' //true
10 == '99' //false
10 != '99' //true
10 != '10' //true
Explanation:
In this example, the first operand is of numeric type and the second operand is of string
type. The ==
operator converts a string type to a number.
The first output is true because 10 and 10 are equal, so it is true for the ==
operator, and the second output is false because 10 and 99 are not equal.
The third output is true because 10 and 99 are not equal, so the output of the !=
operator is true and the fourth output is false because 10 and 10 are equal.
Comparing booleans
and numbers
true == 1 //true
true == 0 //false
false != 1 //true
false != 0 //false
Explanation:
In this example, the first operand is of boolean type and the second operand is of numeric type (0 or 1). The ==
operator converts a numeric type to a Boolean value.
The first output is true because true and 1 are equal (because 1 is considered true and 0 is considered false) so the output of the ==
operator is true, and the second output is true because true and 0 are not equal.
Comparing null
and undefined
let a = null;
let b;
console.log(a == b);
//output: true
Explanation:
For the ==
operator, it outputs true, which compares true for null
and undefined
.
comparing objects
let car1 = {
name: "Maruti"
}
let car2 = {
name: "Maruti"
}
console.log(car1 == car1);
//output: true
console.log(car1 == car2);
//output: false
console.log(car1 != car1);
//output: false
console.log(car1 != car2);
//output: true
Explanation:
In the last example, the first output is true
because car1 and car1 refer to the same instance, while the second output is false
because car1 and car2 refer to different instances.
==
operators.This only works if it's the same object. Different objects with the same value are not equal. Thus
==
operator returns false when we compare two different objects with the same value.What is ===
and !==
in JavaScript?
The ===
operator is called the strict equality operator (and !==
is called the strict inequality operator). The === operator follows the strict equality comparison algorithm, that is, it does not perform type conversion of the operands before comparing their values, even if the data types are The operands are different.
For equality:
x === y
For inequality:
x !== y
where x and y are operands and the middle part is the operator. In case of equality comparison we use === operator and in case of inequality comparison we use !== operator.
Return type: boolean
It returns either true
or false
.
The ===
operator compares operands and returns true if both operands have the same data type and have a certain value, otherwise returns false.
The !==
operator compares operands and returns true if the two operands have different data types or have the same data type but different values. Returns false if both operands are of the same data type and have the same value.
==
operator, ===
does not perform type conversions.Example:
let a = 10;
let b = '10';
let c = 10;
console.log(a===b);
//output: false;
console.log(a===c);
//output: true;
Here the first output is false
because a is a number
type and b is a string
type; the second output is true
because a and c have the same data type and value.
The ===
and !==
operators follow the strict equality comparison algorithm to compare two operands.
Here are some highlights of the strict equality comparison algorithm:
- If the operands we compare are of different data types, then it returns false.
- If either of the two operands we compare is NaN then it will return false.
- If the operand we compare is null or undefined then it will return true.
- If the operands we are comparing are objects, then it will return true if both refer to the same object, otherwise, it will return false.
When the data types of the two operands are different
34 === '34' //false
34 !== '34' //true
Explanation:
Here the first operand is a number data type and the second operand is a string data type, so ===
operator returns false and !==
operator returns true.
When either operand is NaN
let a = NaN;
let b = 10;
a === b //false
a !== b //true
Explanation:
Since the first operand is of the NaN
form, the ===
operator returns false and the !==
operator returns true.
When both operands are null
or undefined
null === null //true
undefined === undefined //true
null === undefined //false
Explanation:
Here the first two comparisons output true because both operands are of the same type and have the same value, but the last outputs false because one operand is null and the other is undefined.
When both operands are object
let car1 = {
name: "Audi"
}
let car2 = {
name: "Audi"
}
console.log(car1 === car1);
//output: true
console.log(car1 === car2);
//output: false
console.log(car1 !== car1);
//output: false
console.log(car1 !== car2);
//output: true
Explanation:
In this example, the first output is true because car1 and car1 refer to the same instance, while the second output is false because car1 and car2 refer to different instances.
Using == and === operands in JavaScript
Both ==
and ===
operators are used to comparing operands in Differences Between ==
and ===
in Javascript.
The ==
and ===
operands are used to compare two values for equality.
The ==
operand loosely compares two values, so it can be used in cases where the data types of the operands are unimportant. For example, imagine a table entry where you ask students for their roll numbers. Probably some will enter it as a string and some as a number. In this case Data can be validated without a database using the ==
operator.
The ===
operand strictly compares two values, so it is used where the data type of the operands matters. Imagine an online coding competition where the answers are numbers in string format. In this case, we will use the ===
operator to compare and validate the answers.
Comparison and difference between ==
and ===
in Javascript
No | == | === |
---|---|---|
1 | Compares two operands | Compares two operands |
2 | returns true if operands have the same data type and same value, returns false if the values differ. | returns true only if operands are of same data type and same value, otherwise returns false |
3 | In case both operands are of different data types, it performs type conversion of one operand in order to make the data types of the operands the same. | In case both operands are of different data type, it doesn't perform type conversion of the operands. |
4 | Also known as loose equality | Also known as strict equality |
5 | Follows abstract equality comparison algorithm | Follows strict equality comparison algorithm |
Examples
- Here are some examples of the behavior of the == operator:
console.log(2 == 2);
//output: true, because 2 and 2 are the same.
console.log(2 == '2');
//output: true, because the string '2' gets converted into a number before comparison.
console.log(false == false);
//output: true, as the operands are the same.
console.log( false == 0 );
//output: true, as the == operator does the type conversion, and then the '0' entity is treated as false.
let student1 = {
name: 'John',
class: 10
}
let student2 = {
name: 'John',
class: 10
}
let student3 = {
name: 'Peter',
class: 10
}
console.log(student1 == student1);
//output: true, as both the operands refer to the same object.
console.log(student1 == student2);
//output: false, as student1 and student2 refer to different objects.
console.log(student1 == student3);
//output: false, as student1 and student3 refer to different objects.
- Here are some examples of the behavior of the === operator:
console.log(2 === 2);
//output: true, because 2 and 2 have the same data type and value
console.log(2 === '2');
//output: false, because the === operator doesn't do the type conversion and the data types of both operands are different.
console.log(false === false);
//output: true, as the operands have the same data type and value.
console.log( false === 0 );
//output: false, as the === operator doesn't do the type conversion and the data types of both operands are different.
let student1 = {
name: 'John',
class: 10
}
let student2 = {
name: 'John',
class: 10
}
let student3 = {
name: 'Peter',
class: 10
}
console.log(student1 === student1);
//output: true, as both the operands refer to the same object.
console.log(student1 === student2);
//output: false, as student1 and student2 refer to different objects.
console.log(student1 === student3);
//output: false, as student1 and student3 refer to different objects.
Conclusion
- The
==
and===
operators are used to check the equality of two operands. - The
!=
and!==
operators are used to check the inequality of two operands. ==
and!=
are loose equality operators, that is, they perform type conversion on the operands before comparison.===
and!==
are strict equality operators, i.e. they compare the operands without any type conversion and return false (in the case of the===
operator), even if the data types are different.- The
==
and!=
operators are used in cases where the data types of the operands are not a major factor in the comparison and can be twisted to allow comparison of the two operands. For example the==
operator can be used to validate a student's enrollment number (obtained through the form and available in String or Number type) to store the admission number (Number data type) in the database. - The
===
and!==
operators are used in cases where the data types of the operands are important to the comparison and cannot be changed for comparison. For example in a coding competition, answers can be in the form of numbers or strings, but according to the rules, points will be awarded Answers for string types only. In this case, we'll use the===
operator to compare the user's answer with the answer stored in our database.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===