Looping Backwards and Loops in Loops (JS)
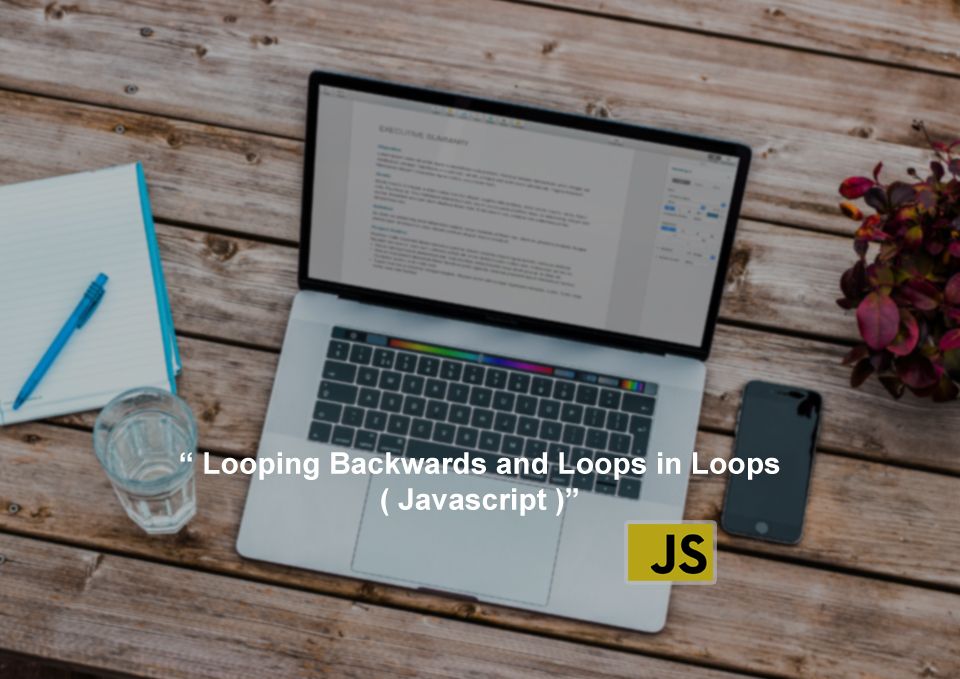
A popular programming language for developing web apps is JavaScript. Looping is one of the key ideas in JavaScript programming. Loops make it simpler to do repetitive tasks by allowing developers to execute a block of code repeatedly.
This article will show you how to utilize JavaScript loops in web development as well as how to make your code better by using backward looping and nested loops.
Backward looping
Allows developers to iterate over an array or string backward. This can be helpful in certain situations, such as when you need to process an array or string in reverse order.
Why loop backwards?
imagine you are at the end of a line of people, and you need to count how many people are in the line. You could start at the first person and count each person until you reach the end, or you could start at the end of the line and count backward to the beginning.
Can FOR loops go backwards?
Backward looping can be implemented using a for
loop, just like a regular loop. The only difference is that the loop variable starts at the end of the array or string and decrements instead of increments.
Example backward looping:
const array = ['A', 'B', 'C', 'D'];
for (let i = array.length - 1; i >= 0; i--) {
console.log(array[i]);
}
This loop will iterate over the array
backward, starting at the last element and ending at the first element.
Examples of Web App Features:
Here's an example of how backward looping can be used to display search results in reverse order:
const searchResults = ['Result 1', 'Result 2', 'Result 3'];
for (let i = searchResults.length - 1; i >= 0; i--) {
console.log(searchResults[i]);
}
Nested loops (Loops in Loops)
Contrarily, nested loops enable programmers to iterate over several arrays or objects concurrently, enabling the execution of intricate data operations.
Nested loops can be explained using the analogy of a Russian doll. Nesting loops have loops inside of them, just like a Russian doll has smaller dolls inside of it. Similar to how you can access the data inside each layer of a nested loop, you can access the smaller dolls by opening up each layer of the larger doll.
why nested loops ?
When you need to cycle through the same data numerous times, nested loops come in handy. In a nested loop, the inner loop executes its operations on each iteration of the outer loop because it runs inside the outer loop. Working with multidimensional arrays, tables, and other intricate data structures can benefit from this.
Scenario where nested loops are useful
You can use nested loops to display a table of data, which is a typical use case.
In this scenario, you would iterate through each row of the table using an outer loop and each column of the row using an inner loop. This enables you to present the facts in an ordered and organised manner.
Implementation of nested loops
For example, if you have an array of arrays, you could use a nested for loop to iterate through the outer array and then iterate through each element of the inner array:
let data = [ [1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
for (let i = 0; i < data.length; i++) {
for (let j = 0; j < data[i].length; j++) {
console.log(data[i][j]);
}
}
In this example, the outer loop iterates through the rows of the array, and the inner loop iterates through the columns of each row.
Examples of web app features
For example, you could use nested loops to display a calendar, where the outer loop iterates through each week of the month and the inner loop iterates through each day of the week. You could also use nested loops to perform complex calculations on data, such as analyzing sales data over time.
// Set the number of days in the month and the starting day of the week
let daysInMonth = 31;
let startingDayOfWeek = 1; // 0 = Sunday, 1 = Monday, 2 = Tuesday, etc.
// Initialize a two-dimensional array to hold the calendar data
let calendar = [];
// Create the outer loop to iterate through each week of the month
for (let week = 0; week < 6; week++) {
// Initialize a new array to hold the days of the week
let weekData = [];
// Create the inner loop to iterate through each day of the week
for (let dayOfWeek = 0; dayOfWeek < 7; dayOfWeek++) {
// Calculate the day of the month for the current cell
let dayOfMonth = (week * 7) + dayOfWeek + 1 - startingDayOfWeek;
// Check if the current cell is within the bounds of the month
if (dayOfMonth >= 1 && dayOfMonth <= daysInMonth) {
// Add the day of the month to the week's data array
weekData.push(dayOfMonth);
} else {
// Add a null value to represent an empty cell in the calendar
weekData.push(null);
}
}
// Add the week's data array to the calendar
calendar.push(weekData);
}
// Output the calendar to the console
console.log(calendar);
This code creates a monthly calendar by iterating through each week of the month using an outer loop, and then iterating through each day of the week using an inner loop. It calculates the day of the month for each cell and adds it to the corresponding week's data array, or adds a null value to represent an empty cell if the day is outside the bounds of the month.
Summary of key point
- Backward looping is used to iterate over an array or string in reverse order, and can be implemented using a for loop with a loop variable that decrements instead of increments.
- Backward looping can be useful in situations where processing data in reverse order is needed, such as displaying search results in reverse order.
- Nested loops allow developers to iterate over multiple arrays or objects simultaneously, enabling complex data operations.
- Nested loops can be used to display tables of data or perform calculations on multidimensional arrays or other complex data structures.
- Implementation of nested loops can be done by using a nested for loop to iterate through the outer array and then iterate through each element of the inner array.
- An example of a scenario where nested loops are useful is to display a calendar, where the outer loop iterates through each week of the month and the inner loop iterates through each day of the week.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Javascript array operations (Methods)
- Javascript Dot vs. Bracket Notation (comparison)
- Javascript: for loop (Example)
- Looping Backwards and Loops in Loops (JS)