Next.js 14 Navigating Between Pages - Wrong & Right Solution
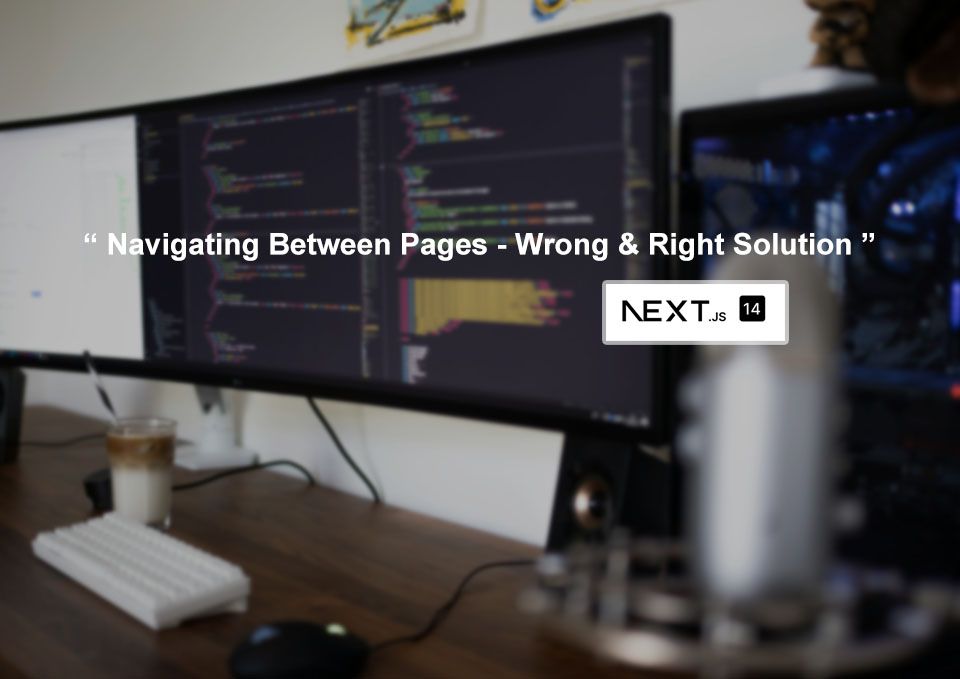
In the world of modern web development, providing a seamless and efficient experience for users as they navigate between pages is crucial. Traditional approaches often involved reloading the entire page, which can lead to performance issues and poor user experiences. However, with the advent of Single Page Applications (SPAs), developers now have a powerful solution for delivering smooth and responsive page navigation.
The Wrong Solution: Reloading the Entire Page
The traditional approach to page navigation involved reloading the entire page whenever a user clicked a link or performed an action that required a new page. This method worked well in the early days of the web when pages were primarily static and contained minimal interactivity. However, as web applications became more complex and interactive, the limitations of this approach became increasingly apparent.
When the entire page is reloaded, several things happen behind the scenes:
- Server Request: The browser sends a request to the server for the new page.
- Server Processing: The server processes the request and generates the new page content.
- Data Transfer: The server sends the complete HTML, CSS, and JavaScript files for the new page to the browser.
- Page Rendering: The browser renders the new page, discarding the previous page's state and resources.
This process can be inefficient and lead to several issues:
- Increased Server Load: Every page reload requires the server to process a new request, which can strain server resources, especially during periods of high traffic.
- Increased Bandwidth Usage: Transferring the complete page content for every navigation can consume significant bandwidth, particularly for users with slower internet connections.
- Slower Page Load Times: Users must wait for the entire page to load, even if only a small portion of the content has changed, leading to a frustrating experience.
- Loss of Application State: Any client-side state or data maintained by the previous page is lost during the reload, requiring additional effort to manage and persist this information.
While this approach may still be suitable for simple, static websites, it quickly becomes problematic for modern, dynamic web applications that prioritize performance and user experience.
The Right Solution: Single Page Applications (SPAs)
To address the limitations of the traditional page reload approach, developers have embraced the concept of Single Page Applications (SPAs). An SPA is a web application that loads completely on the initial page load and subsequent navigation updates only the necessary parts of the page, rather than reloading the entire page.
Here's how SPAs work:
- Initial Page Load: The browser fetches the complete application code (HTML, CSS, and JavaScript) from the server.
- Application Bootstrapping: The SPA framework (e.g., React, Angular, Vue) initializes and renders the initial view.
- Navigation Events: When a user interacts with the application (e.g., clicks a link or button), the SPA framework intercepts the navigation event.
- Partial Updates: Instead of reloading the entire page, the SPA framework updates only the necessary parts of the page, typically by rendering new components or views while preserving the application state.
This approach offers several advantages:
- Improved Performance: By avoiding full page reloads, SPAs minimize the amount of data transferred between the client and server, resulting in faster load times and a more responsive user experience.
- Efficient Use of Resources: With fewer server requests and reduced data transfers, SPAs place less strain on server resources and bandwidth.
- Seamless User Experience: Users experience smooth transitions between views, without disruptive page reloads or flickering, contributing to a more polished and engaging experience.
- Maintained Application State: Client-side state and data are preserved during navigation, reducing the need for additional logic to manage and persist this information.
To implement an SPA, developers typically rely on client-side routing and state management libraries provided by popular front-end frameworks like React
, Angular
, and Vue
.
Core Components of SPAs
Client-side Routing
Client-side routing is a fundamental aspect of SPAs, enabling the application to handle navigation and URL changes without triggering a full page reload. Instead of relying on traditional server-side routing, client-side routing libraries handle URL changes and update the application's view accordingly.
Popular routing libraries include:
- React Router (for React applications)
- Angular Router (for Angular applications)
- Vue Router (for Vue.js applications)
Here's an example of how client-side routing works in a React application using React Router:
import { BrowserRouter as Router, Switch, Route, Link } from 'react-router-dom';
function App() {
return (
<Router>
<div>
<nav>
<ul>
<li>
<Link to="/">Home</Link>
</li>
<li>
<Link to="/about">About</Link>
</li>
<li>
<Link to="/contact">Contact</Link>
</li>
</ul>
</nav>
{/* A <Switch> looks through its children <Route>s and
renders the first one that matches the current URL */}
<Switch>
<Route path="/about">
<About />
</Route>
<Route path="/contact">
<Contact />
</Route>
<Route path="/">
<Home />
</Route>
</Switch>
</div>
</Router>
);
}
In this example, the Router
component from React Router provides the routing functionality. The Link
components render navigation links, and the Switch
and Route
components determine which component (e.g., Home
, About
, Contact
) to render based on the current URL.
State Management
As applications grow in complexity, managing and sharing data between components becomes a critical concern. State management libraries provide a structured approach to managing and updating the application's state, ensuring that components have access to the data they need and updates are propagated efficiently.
Popular state management libraries include:
- Redux (for React applications)
- MobX (for React applications)
- Vuex (for Vue.js applications)
Here's an example of how state management works in a React application using Redux:
// Redux store
import { createStore } from 'redux';
// Define the initial state
const initialState = {
count: 0
};
// Define the reducer
function counterReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
// Create the Redux store
const store = createStore(counterReducer);
// React component
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
function Counter() {
const count = useSelector(state => state.count);
const dispatch = useDispatch();
return (
<div>
<h1>{count}</h1>
<button onClick={() => dispatch({ type: 'INCREMENT' })}>Increment</button>
<button onClick={() => dispatch({ type: 'DECREMENT' })}>Decrement</button>
</div>
);
}
In this example, Redux manages the state of a simple counter application. The createStore
function creates a Redux store with the counterReducer
function, which updates the state based on the dispatched actions ( INCREMENT
or DECREMENT
). The Counter
component uses the useSelector
hook to access the current state and the useDispatch
hook to dispatch actions that update the state.
Best Practices for Implementing SPAs
Code Splitting and Lazy Loading
As web applications grow in size and complexity, the initial bundle size can become substantial, leading to longer load times and poor performance. Code splitting and lazy loading techniques help mitigate this issue by breaking the application code into smaller chunks and loading them on-demand as needed.
Code splitting involves separating the application code into multiple bundles, each containing the code for a specific feature or route. When a user navigates to a particular route, only the necessary code bundles are loaded, reducing the initial load time and improving performance.
Lazy loading takes code splitting a step further by deferring the loading of non-critical code until it is actually required. This approach can significantly reduce the initial load time and improve the overall user experience.
Most modern front-end frameworks and bundlers (e.g., webpack, Rollup) provide built-in support for code splitting and lazy loading, making it easier for developers to implement these techniques.
Handling Browser History and URLs
In traditional server-rendered applications, the browser's history and URL are managed by the server, ensuring that bookmarking and sharing links work as expected. However, in SPAs, where navigation is handled client-side, additional steps are needed to manage the browser history and URLs correctly.
Failing to handle browser history and URLs properly can lead to issues such as:
- Broken Bookmarks and Shared Links: If the browser's history and URLs are not updated correctly, bookmarked or shared links may not load the intended application state or view.
- Inability to Refresh or Navigate Back/Forward: Users may encounter errors or unexpected behavior when refreshing the page or using the browser's back and forward buttons.
- Poor SEO Performance: Search engines may have difficulty indexing and crawling SPA content if URLs are not handled correctly.
To address these concerns, SPA frameworks provide utilities and libraries for managing browser history and URLs.
For example, React Router provides the useHistory
hook and the history
object for managing browser history, while Angular Router and Vue Router offer similar functionality.
Here's an example of how to manage browser history in a React application using React Router:
import { useHistory } from 'react-router-dom';
function NavigationComponent() {
const history = useHistory();
const handleNavigate = () => {
// Navigate to a new route
history.push('/new-route');
};
const handleGoBack = () => {
// Go back to the previous route
history.goBack();
};
return (
<div>
<button onClick={handleNavigate}>Navigate to New Route</button>
<button onClick={handleGoBack}>Go Back</button>
</div>
);
}
In this example, the useHistory
hook from React Router provides access to the browser history object. The history.push
method is used to navigate to a new route, while history.goBack
allows the application to go back to the previous route.
Additionally, libraries like history
(from the history
package) provide advanced functionality for managing browser history, such as handling hash-based URLs (example.com/#/route) or HTML5 pushState
URLs (example.com/route).
Optimizing Initial Load Time
While SPAs offer improved performance for subsequent navigation, the initial load time can be longer compared to traditional server-rendered applications. This is because the entire application code needs to be downloaded and parsed before the initial view can be rendered.
To mitigate this issue, developers can employ various techniques:
- Code Splitting and Lazy Loading: As discussed earlier, code splitting and lazy loading help reduce the initial bundle size by loading only the necessary code upfront.
- Minification and Compression: Minifying (removing unnecessary whitespace and comments) and compressing (gzipping) the application code can significantly reduce the file size and improve load times.
- Server-side Rendering (SSR): Server-side rendering involves pre-rendering the initial application state on the server and sending the rendered HTML to the client. This approach provides a faster initial load time but requires additional server resources and complexity.
- Pre-rendering: Pre-rendering involves generating static HTML files for each page or route during the build process. These pre-rendered files can be served directly to the client, providing a fast initial load time without the need for server-side rendering during runtime.
The choice of optimization techniques will depend on the specific requirements and constraints of the application, such as performance goals, server capabilities, and team expertise.
Considerations and Drawbacks of SPAs
While SPAs offer significant advantages in terms of performance and user experience, there are some considerations and potential drawbacks to keep in mind:
Initial Load Time
As mentioned earlier, the initial load time for SPAs can be longer compared to traditional server-rendered applications due to the need to download and parse the entire application code. While techniques like code splitting, lazy loading, and pre-rendering can help mitigate this issue, developers should still be mindful of the potential impact on user experience, especially for users with slower internet connections or limited device capabilities.
SEO Challenges
Search engine optimization (SEO) can be more challenging with SPAs because search engine crawlers may have difficulty indexing and rendering the dynamic content generated by client-side JavaScript. However, there are several strategies to address this:
- Server-side Rendering (SSR): By pre-rendering the initial application state on the server, search engines can crawl and index the rendered HTML content.
- Pre-rendering: Similar to SSR, pre-rendering generates static HTML files for each page or route, which can be easily indexed by search engines.
- Dynamic Rendering: Services like Prerender.io or Puppeteer can be used to dynamically render and serve pre-rendered content to search engine crawlers.
- Metadata and Structured Data: Providing relevant metadata and structured data within the HTML can improve the discoverability and ranking of SPA content.
While these strategies can help improve SEO for SPAs, it's important to continuously monitor and adapt to best practices as search engine algorithms and guidelines evolve.
Conclusion
Navigating between pages efficiently is a critical aspect of modern web development, and the choice between reloading the entire page or adopting a Single Page Application (SPA) approach can significantly impact performance and user experience.
The traditional approach of reloading the entire page for each navigation can lead to increased server load, higher bandwidth usage, slower load times, and loss of application state. In contrast, SPAs offer a more efficient and responsive solution by updating only the necessary parts of the page during navigation, resulting in improved performance, efficient resource utilization, and a seamless user experience.
To implement SPAs effectively, developers must leverage client-side routing and state management libraries provided by popular front-end frameworks like React, Angular, and Vue. Additionally, best practices such as code splitting, lazy loading, proper handling of browser history and URLs, and optimizing initial load times should be followed to ensure optimal performance and user experience.
While SPAs introduce some challenges, such as potential longer initial load times and SEO considerations, various techniques and strategies can be employed to mitigate these issues. By adopting modern web development practices and leveraging the power of SPAs, developers can build performant, engaging, and user-friendly web applications that deliver a superior navigation experience.