Learn Javascript looping arrays
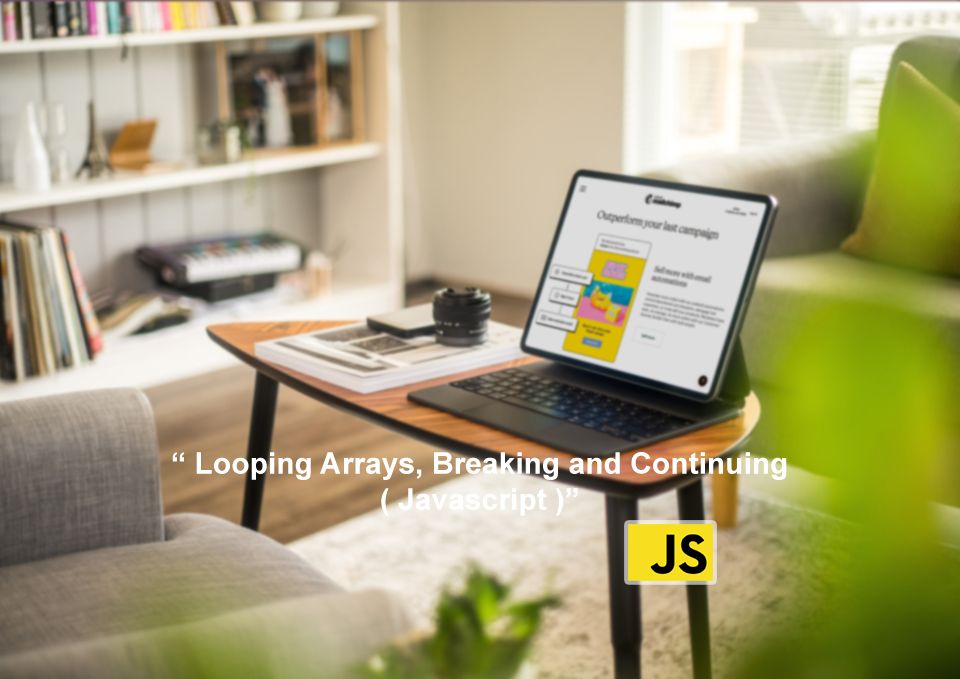
Looping arrays are one of the most important concepts to understand when working with Javascript. This article will provide a clear and concise explanation of what looping arrays are, why they are important in web development, and what breaking and continuing in looping arrays means.
Imagine you have a task that you need to perform multiple times, such as making a sandwich. Doing this task manually, one sandwich at a time, can be time-consuming and monotonous. This is where looping arrays in Javascript come in. Looping arrays allow you to repeat a block of code multiple times, just like making multiple sandwiches quickly and efficiently.
Can you use break and continue in for loops?
You may manage the flow of your loops using the principles of breaking and continuing in looping arrays. Think of it like making a sandwich. If you accidentally drop the bread on the floor, you would break the loop by discarding that piece of bread and starting the process over again. If you find that you're out of a certain ingredient, you would continue the loop by skipping that step and moving on to the next one.
Types of Looping Arrays
1. For Loop:
A for loop is a control structure that allows you to repeat a block of code a specified number of times. The basic syntax of a for loop is:
for (let i = 0; i < array.length; i++) {
// code to be executed
}
Consider it similar to making a sandwich. You have the necessary components, and you want to keep adding them to the bread until you get the required number. The for loop functions as a recipe that specifies how many times to repeat an action.
2. For...of Loop:
The for...of loop is used to iterate over the elements of an array. The basic syntax is:
for (let element of array) {
// code to be executed
}
Think of it as going through your closet to find a specific outfit. You want to look at each piece of clothing one by one until you find the outfit you're looking for. The for...of loop is like the process you follow to find the outfit.
3. For...in Loop:
The for...in loop is used to iterate over the properties of an object. The basic syntax is:
for (let property in object) {
// code to be executed
}
Consider it like organizing your bookcase. You have a lot of books, and you want to make sure each one is put away properly. The method you use to organize your books is comparable to the for...in loop.
4. While Loop:
The while loop is a control structure that allows you to repeat a block of code as long as a specified condition is true. The basic syntax is:
while (condition) {
// code to be executed
}
Think of it as playing a game. You play until you either win or lose. The while loop is like the rules of the game that determine when you win or lose.
5. Do...While Loop:
The do...while loop is similar to the while loop, except that it guarantees that the code inside the loop will be executed at least once. The basic syntax is:
do {
// code to be executed
} while (condition);
Imagine it like a trip to the grocery. You visit the store, make your purchase, and then determine if you require anything else. The do...while loop is comparable to the procedure you use to check your shopping list before you head to the store.
Advantages of Using Looping Arrays in JavaScript
- Iterating Over Elements: The looping arrays feature of JavaScript makes it easy to iterate through and perform operations on each individual element of an array.
- Repeated Code Executions: Because JavaScript's looping arrays allow for multiple code executions, it is the ideal solution for repetitive tasks.
- Dynamic output: Looping arrays in JavaScript produces dynamic output, enabling you to modify the output of your code according to the elements in the array.
Imagine you have an array of integers and you want to calculate the sum of the array's numbers. You might traverse through the array's elements using a for loop and add each one to a sum variable. The outcome would be the array's total number of elements. This is just one illustration of how JavaScript's looping arrays can be used to generate dynamic output.
How do you break and continue a loop?
Breaking and continuing in looping arrays refer to the ability to control the flow of your loops. Breaking a loop means exiting the loop early, while continuing a loop means skipping the current iteration and moving on to the next one.
Syntax of Breaking a Loop:
Breaking a loop in JavaScript is done using the "break" keyword. The basic syntax is:
for (let i = 0; i < array.length; i++) {
if (condition) {
break;
}
}
Imagine yourself engaging in a game. When you reach a specific level in the game, you wish to cease participating and exit. When you use the break keyword, the game will end.
Using Break to Exit a Loop Early:
Breaking a loop in JavaScript is useful when you want to exit the loop early based on a certain condition.
For example : let's say you have an array of numbers and you want to find the first number that is greater than 10
. You could use a for loop to iterate over the elements in the array and use the break keyword to exit the loop as soon as you find the first number that is greater than 10.
Syntax of Continuing a Loop:
Continuing a loop in JavaScript is done using the "continue" keyword. The basic syntax is:
for (let i = 0; i < array.length; i++) {
if (condition) {
continue;
}
}
Think of it as playing a game. If you reach a certain level and don't want to continue playing that level, you want to skip to the next level. The continue keyword is like skipping to the next level.
Skipping an Iteration in a Loop:
Continuing a loop in JavaScript is useful when you want to skip the current iteration of the loop based on a certain condition.
For example, let's say you have an array of numbers and you only want to sum the even numbers. You could use a for loop to iterate over the elements in the array and use the continue keyword to skip the current iteration if the current element is not even.
Applying Looping Arrays, Breaking and Continuing in a Web Application
Example 1: Displaying an Array of Objects
In this example, we will create an array of objects, loop over the elements in the array using a for loop, and break the loop if a certain condition is met.
Creating an Array of Objects:
To create an array of objects in JavaScript, we can use the following code:
let objectsArray = [ { name: "John", age: 28 }, { name: "Jane", age: 32 }, { name: "Jim", age: 26 } ];
Looping Over the Array Using a For Loop:
To loop over the elements in the array and display the information, we can use a for loop. Here is an example:
for (let i = 0; i < objectsArray.length; i++) {
console.log("Name: " + objectsArray[i].name + ", Age: " + objectsArray[i].age);
}
The name and age of every individual in the console will be displayed as this for loop iterates through the elements in the array.
Breaking the Loop if a Condition is Met:
For example, if we only want to display the information of the person who is 32 years old, we can use the following code:
for (let i = 0; i < objectsArray.length; i++) {
if (objectsArray[i].age === 32) {
console.log("Name: " + objectsArray[i].name + ", Age: " + objectsArray[i].age);
break;
}
}
When it encounters an object with the age of 32, this for loop will run over the objects in the array and terminate. As a result, only one person's information—"Name: Jane, Age: 32"—will be shown in the console.
Example 2: Creating a Dynamic List
In this example, we will use a for...of loop to display a list of elements, use the "continue" statement to skip an iteration, and implement a search functionality.
Using a For...of Loop to Display a List of Elements:
To display a list of elements, we can use a for...of loop. Here is an example:
let list = ["apple", "banana", "cherry", "date", "elderberry"];
for (const element of list) {
console.log(element);
}
This for...of loop will traverse through the list's elements, displaying each one on the console.
Using Continue to Skip an Iteration:
To skip an iteration in a loop, we can use the "continue" statement.
For example, if we want to skip the iteration for the "banana" element in the list, we can use the following code:
let list = ["apple", "banana", "cherry", "date", "elderberry"];
for (const element of list) {
if (element === "banana") {
continue;
}
console.log(element);
}
This is for...of loop
will iterate through the elements in the list and skip the iteration for the "banana"
element, resulting in the display of the other parts in console: "apple", "cherry", "date", and "elderberry"
.
Example 3: Creating a Responsive Navigation Bar
A navigation bar is an essential component of any website since it allows users to swiftly and easily navigate the various areas of the site. In this example, we'll use a while loop in JavaScript to create a responsive menu bar. The navigation bar will be able to generate links dynamically, interrupt the loop if a condition is fulfilled, and improve the user experience.
Using a While Loop to Generate Navigation Links
Here is an example of how we can use a while loop to generate navigation links in a responsive navigation bar:
let links = ['Home', 'About', 'Services', 'Contact'];
let i = 0;
while (i < links.length) {
console.log(`<a href="#">${links[i]}</a>`);
i++;
}
We begin by creating an array of links with the values "Home," "About," "Services," and "Contact"
in this code. A while loop is then used to iterate over the array and construct navigation links for each item. The I variable serves as the loop's counter and is incremented by one each time the loop executes.
Breaking the Loop if a Condition is Met
If a certain condition is met, we may need to break the loop.
For example, we might only want a few links on the navigation bar. We can use the break statement to leave the loop early in this scenario.
let links = ['Home', 'About', 'Services', 'Contact'];
let i = 0;
while (i < links.length) {
console.log(`<a href="#">${links[i]}</a>`);
i++;
if (i === 2) {
break;
}
}
We added a conditional statement to this code to see if I equals 2. If this condition is met, the loop will terminate prematurely and only the first two links will be displayed.
Summary of Key Points
This article provides an explanation of looping arrays in Javascript. Looping arrays allow repeating a block of code multiple times, making it efficient for repetitive tasks. There are 5 types of looping arrays in Javascript: For Loop, For...of Loop, For...in Loop, While Loop, and Do...While Loop. Each type has its own syntax and usage. Breaking and continuing in looping arrays refer to the ability to control the flow of loops. Breaking a loop means exiting the loop early using the "break" keyword, while continuing a loop means skipping the current iteration and moving on to the next one using the "continue" keyword. Advantages of using looping arrays include iterating over elements, repeated code execution, and dynamic output.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Javascript array operations (Methods)
- Javascript Dot vs. Bracket Notation (comparison)
- Javascript: for loop (Example)
- javascript looping arrays