Understanding JavaScript Boolean Logic: A Beginner's Guide
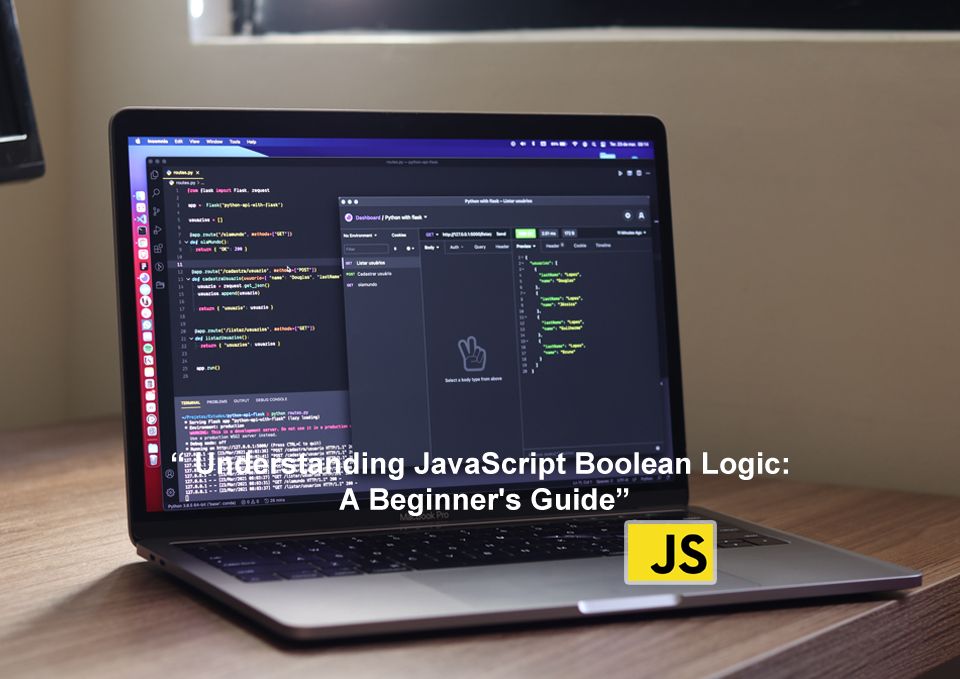
Boolean logic is a fundamental concept in programming that involves using true/false
values to make decisions in your code. It's the foundation of if/else
statements and other decision-making processes. In JavaScript, the two values used in Boolean logic are true
and false
.
Why Use Boolean Logic?
Boolean logic is crucial for making decisions in your code based on certain conditions. For example, you can use Boolean logic to check if a number is even or odd, if a user is logged in, or if a certain form field has been filled out correctly.
Using Boolean logic makes your code more dynamic and flexible. You can add multiple conditions and have different outcomes based on those conditions. This can save time and effort compared to hard-coding every possible outcome.
Sample Code and Implementation :
Here's a simple example of using Boolean logic in JavaScript to determine if a number is even or odd:
let num = 4;
let isEven = (num % 2 === 0);
if (isEven) {
console.log(num + " is even.");
} else {
console.log(num + " is odd.");
}
In this code, we first assign the value 4
to the variable num. Then, we use the module operator (%)
to find the remainder when dividing num by 2
. If the result is 0
, the number is even, and the value of isEven will be true. If the result is anything other than 0
, the number is odd, and the value of isEven will be false
.
Finally, we use an if/else
statement to check the value of isEven. If it's true, the code inside the if block will run and log 4 is even
. to the console. If it's false, the code inside the else block will run and log 4 is odd
. to the console.
Conclusion
Boolean logic is a simple but powerful tool for making decisions in your code. It allows you to add conditions and create dynamic outcomes based on those conditions.
It's a fundamental concept that every JavaScript developer should understand, and it's used in a wide range of applications, from simple scripts to complex web applications.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)