JavaScript switch Statement (Beginner Guide)
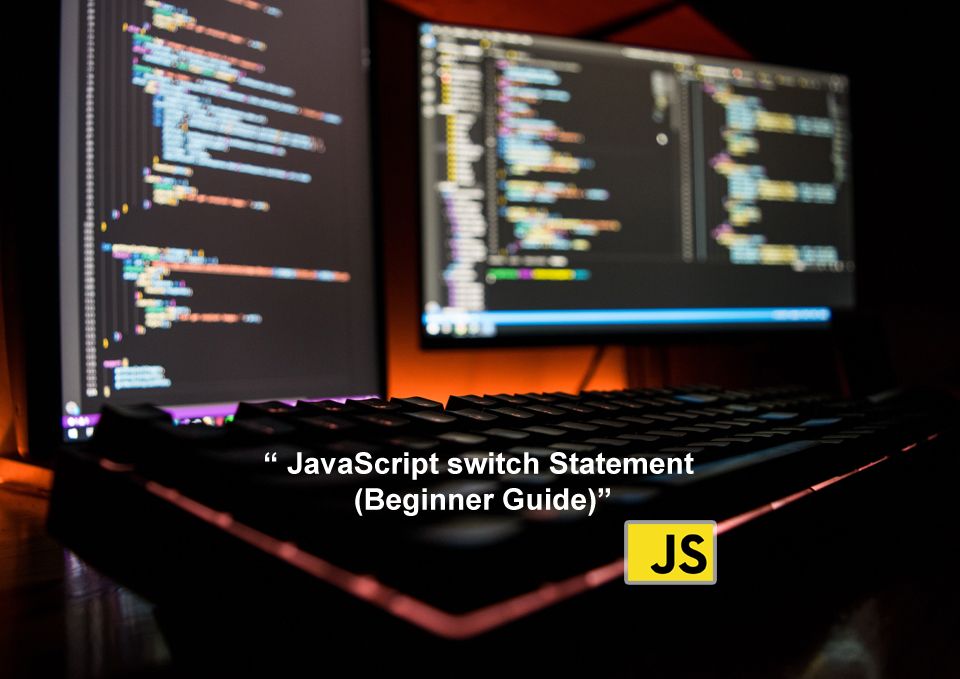
JavaScript switch statement is a control flow structure that is commonly used to perform different actions based on different conditions. If you're a beginner in JavaScript, it can be a bit confusing, but don't worry! This guide will help you understand the fundamentals of switch statements in a way that's easy to understand.
In JavaScript, the switch statement provides a way to test the value of an expression against multiple cases and execute different codes for each case. It's a great way to simplify complex if-else statements and make your code more readable and maintainable.
How to use a switch statement:
switch (expression) {
case value1:
// code to be executed if expression === value1;
break;
case value2:
// code to be executed if expression === value2;
break;
default:
// code to be executed if expression doesn't match any of the values;
}
In the example above, the expression
is evaluated and compared against each case
value. If a match is found, the corresponding code block is executed. If no match is found, the code in the default
block is executed.
It's important to include the break
statement after each case, as it stops the code from running into the next case. Without it, all the code after a matching case will be executed, even if it belongs to another case.
Implementation in web applications :
Here's a real-world example of how you can use a switch statement to determine the price of a product based on its size:
let size = "small";
switch (size) {
case "small":
console.log("The price of a small product is $10");
break;
case "medium":
console.log("The price of a medium product is $15");
break;
case "large":
console.log("The price of a large product is $20");
break;
default:
console.log("Invalid size. Please choose from small, medium or large.");
}
In this example, the value of size
is "small", so the code in the case "small"
block will be executed, and the output will be "The price of a small product is $10".
The benefit of using switch statements
The benefit of using switch statements is that you can use them to handle multiple cases with the same code.
Here's an example of how to do this:
let size = "small";
switch (size) {
case "small":
case "medium":
console.log("The price of a small or medium product is $15");
break;
case "large":
console.log("The price of a large product is $20");
break;
default:
console.log("Invalid size. Please choose from small, medium or large.");
}
In this example, both "small" and "medium" sizes will have the same code executed, and the output will be "The price of a small or medium product is $15".
limitations of switch statements
It's important to note that switch statements have some limitations. For example, you can only compare values to the cases using the strict equality operator (===)
, so you can't use comparison operators (>, <, >=, <=)
or perform type conversions.
In addition, switch statements are not the best option when you need to perform complex operations or when you need to test multiple conditions.
In those cases, you may want to use if-else statements or other control flow structures.
Another thing to keep in mind is that switch statements can become difficult to maintain when you have a large number of cases and complex logic. To avoid this, you should keep your switch statements as simple as possible and avoid duplicating code.
Summary
The article provides a beginner guide to JavaScript switch statements and explains why they should be used in web development.
The article explains how switch statements can be used to handle user input, display different content based on different conditions, and make decisions in code, making web pages more dynamic and user-friendly.
The article also highlights the limitations of switch statements, such as only being able to compare values using the strict equality operator and difficulty in maintaining code with a large number of cases and complex logic.
The article concludes by emphasizing the importance of using switch statements appropriately and following best practices to write efficient and maintainable code.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)