Javascript Fundamentals #Data Types
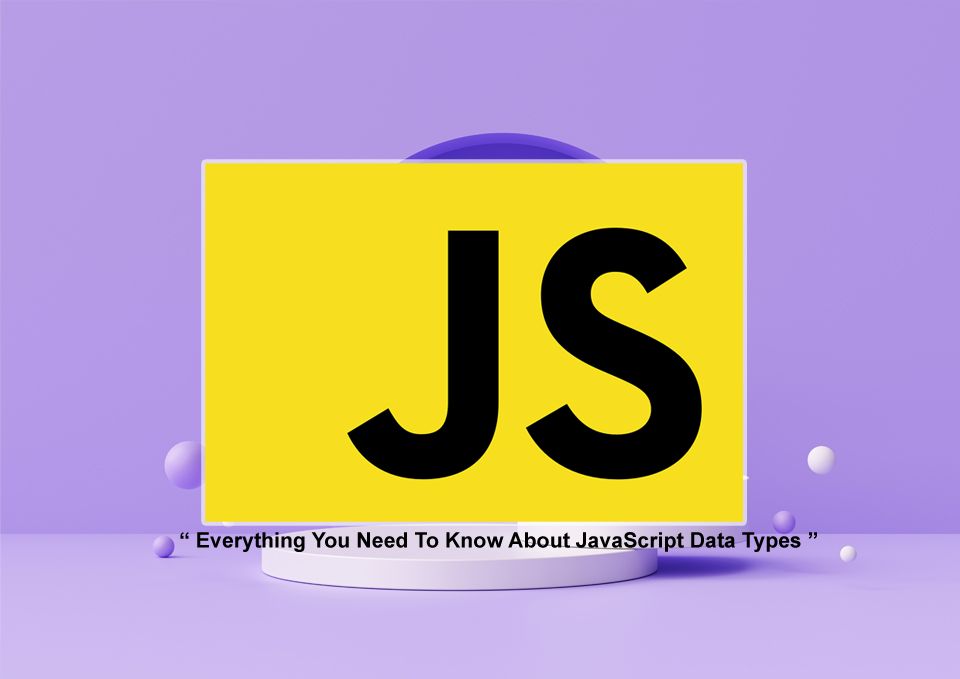
Knowing the different data types available in JavaScript can help you write more efficient and effective code. In this tutorial, we'll explore primitive data types such as numbers, strings, and booleans. We'll also take a look at objects, arrays, events, and validation in JavaScript.
Data Type in Javascript
Learning the Art of Coding with JavaScript Values & Variables
Javascript Data Types
In JavaScript, there are two types of data types: primitive and non-primitive (reference).
Primitive data types include numbers, strings, booleans, null, and undefined.
Non-primitive (reference) data types include arrays, functions, objects and dates.
Variables must be declared with the "var" keyword to indicate their type ; however, once declared the type cannot be changed as JavaScript is a dynamically typed language.
For example:
var x = 3; // x is a number
var x = "Aris" // x is a stringg
Primitives In JavaScript: Primitive Data Types Explained
JavaScript recognizes 5 primitive data types: strings, numbers, Boolean values, undefined values, and null.
- Strings are represented by a sequence of characters like "hello";
- numbers are numeric values such as 100;
- Boolean values can either be true or false;
- undefined represents an unassigned value;
- and null means there is no value.
Non Primitives In JavaScript: Non Primitive Data Types Explained
Non-primitive data types are an integral part of JavaScript. These include objects, arrays, and regular expressions.
- Objects can be used to access members and represent instances.
- array represents a collection of similar values.
- Regular expressions are very useful for manipulating strings according to complex patterns.
Example Code:
String
A JavaScript string is a sequence of characters surrounded by single, double, or backtick quotes.
//strings example
const name = 'ars';
const name1 = "dev";
const result = `The names are ${name} and ${name1}`;
Single quotes ('') and double quotes ("") can typically be used interchangeably, while backticks (
) wrap around variables or expressions, such as  ${variable or expression}. These strings provide a way to store and manipulate text-based data within programs.
Number
JavaScript numbers are numerical data values that can be either integers (whole numbers) or floats (decimals). Additionally, they can also be expressed using exponentials (e.g. 1.02e+3 would be equal to 1020). Examples of JavaScript numbers include whole numbers such as 345, decimal numbers such as 3.456 and exponential numbers like 1.02e+3.
const number1 = 3;
const number2 = 3.433;
const number3 = 3e5 // 3 * 10^5
const number1 = 3/0;
console.log(number1); // Infinity
const number2 = -3/0;
console.log(number2); // -Infinity
// strings can't be divided by numbers
const number3 = "abc"/3;
console.log(number3); // NaN
BigInt
Are you dealing with numbers larger than (253 - 1)? JavaScript's Number type might not be enough. Enter BigInt, a new breed of numeric data type introduced in ES2020 that can handle integer values of arbitrary length. With BigInt, you won't have to worry about the limits of the Number data type ever again!
// BigInt value
const value1 = 900719925124740998n;
// Adding two big integers
const result1 = value1 + 1n;
console.log(result1); // "900719925124740999n"
const value2 = 900719925124740998n;
// Error! BitInt and number cannot be added
const result2 = value2 + 1;
console.log(result2);
Boolean
Boolean, a data type in JavaScript, can only have two values: true or false. It is often used to represent logical entities and can be thought of as a yes/no switch. For example, a boolean can be used to check if an answer to a question is correct or incorrect.
const dataChecked = true;
const valueCounted = false;
undefined
JavaScript undefined is the data type given to a variable that has been declared but not assigned a value. For example, if you create a variable using the var keyword but do not assign it any value, its data type will be undefined.
let name;
console.log(name); // undefined
null
In JavaScript, null is a keyword which indicates the lack of any value or an empty value. It can be used to represent that a variable has no assigned value yet, or an object does not hold any valid data. For example, a variable declared but not initialized would have a null value.
const number = null;
Object
A JavaScript object is a powerful data type that allows users to store and manipulate collections of data. Objects enable a variety of functions, such as the ability to create and use variables, execute methods, assign values and much more. In simple terms, JavaScript objects provide an easy way for developers to group related pieces of information into one structure.
const student = {
firstName: 'ram',
lastName: null,
class: 10
};
Array
A JavaScript array is a special type of variable designed to store multiple values in a single place. It allows you to store and manipulate data in a structured way, making complex operations simpler. With an array, you can easily store and access information such as numbers, strings, objects, and more using indexes for each value stored.
const cars = [
"Saab",
"Volvo",
"BMW"
];
Regular expressions
JavaScript regular expressions are special objects used in pattern matching functions. A RegExp Object usually consists of characters that represent a pattern that can be used for searches and replacements on text strings. The use of Regular Expressions in JavaScript provides developers with powerful tools such as Properties and Methods to perform various text search operations.
const regex = new RegExp(/^a...s$/);
console.log(regex.test('alias')); // true
Data Type in Javascript
Learning the Art of Coding with JavaScript Values & Variables
Finish.