Learn JavaScript Engine and Runtime
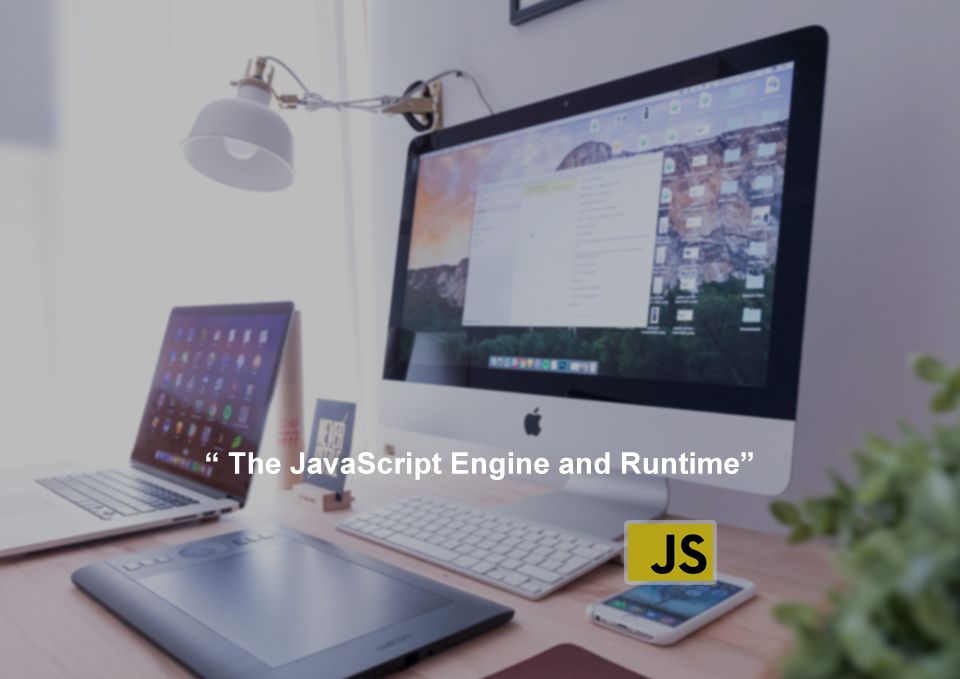
In this Articles, we discussed the definition and importance of JavaScript engines and runtimes. We defined a JavaScript engine as the component of a web browser that interprets and executes JavaScript code, while a JavaScript runtime is an environment that includes the JavaScript engine along with additional features and libraries.
Understanding how these components work is critical for web developers who want to optimize their code for better performance and write server-side JavaScript applications. We provided examples and analogies to help beginners understand these concepts and emphasized the importance of gaining practical experience in applying code to build web applications.
What is the difference between engine and runtime?
A JavaScript engine is the component of a web browser that interprets and executes JavaScript code. It reads JavaScript code, converts it into machine code, and executes it. The most popular JavaScript engines are V8, used in Google Chrome, and SpiderMonkey, used in Mozilla Firefox.
On the other hand, a JavaScript runtime is an environment that includes a JavaScript engine along with other features and libraries. It provides additional functionality to the JavaScript engine, such as the ability to interact with the file system, access databases, and make network requests.
Understanding JavaScript Engine and Runtime
Understanding how a JavaScript engine and runtime work is crucial for web developers because it helps them optimize their code for better performance. For example, knowing how the JavaScript engine handles memory allocation can help developers write more efficient code.
Additionally, knowledge of JavaScript runtime is essential for developers who want to write server-side JavaScript applications. It enables them to use the same language for both client-side and server-side programming, allowing for easier maintenance and better code reuse.
Components of a JavaScript Engine
If you're interested in web development, chances are you've heard of JavaScript. It's a popular programming language used for building interactive web applications. But have you ever wondered how JavaScript code is executed by your web browser? That's where the JavaScript engine comes in.
Parser
The first component of a JavaScript engine is the parser. Its job is to read your JavaScript code and convert it into an internal format that can be executed by the engine. The parser checks your code for syntax errors and creates an abstract syntax tree (AST) representation of your code.
Think of the parser as a translator that converts your code into a language the JavaScript engine can understand. Just like how a language translator needs to understand the grammar and syntax of the languages they are translating, the parser needs to understand the grammar and syntax of JavaScript to create an accurate AST.
Interpreter and Compiler
Once the parser has created the AST, the JavaScript engine needs to execute the code. This is done through a combination of an interpreter and a compiler.
The interpreter reads each statement in the AST and executes it. This is similar to how a musician reads sheet music and plays the notes in order. However, this approach can be slow, especially for larger applications. To improve performance, some JavaScript engines also use a compiler.
A compiler takes the AST and compiles it into optimized machine code that can be executed more quickly. This is like a musician who has memorized the sheet music and can play the piece more quickly because they don't have to keep referring to the sheet music.
Garbage Collector
The final component of a JavaScript engine is the garbage collector. As you execute JavaScript code, you create objects and variables that take up memory. However, if those objects and variables are no longer needed, they can be removed from memory to free up space.
The garbage collector is responsible for identifying and removing unused objects and variables from memory. This is like a janitor who cleans up a room after a party to make sure there's space for the next event.
How does a JavaScript Engine work?
1. Code Execution and Optimization
When you write JavaScript code, you may not realize that it's not actually being executed by your web browser directly. Instead, your code is first processed by the JavaScript engine, which is responsible for converting your code into machine code that your computer can execute.
To illustrate how this works, imagine you're a chef preparing a meal. Your recipe represents the JavaScript code, and your kitchen is the computer. Before you can cook the meal, you need to prepare the ingredients (parse the code), mix them together (execute the code), and put the dish in the oven (optimize the code). The finished dish is the machine code that the computer can execute.
Let's take a closer look at the process. First, the JavaScript engine parses your code, checking for syntax errors and creating an abstract syntax tree (AST). This is like reading the recipe and checking that you have all the necessary ingredients before you start cooking.
Once the AST is created, the engine then interprets the code, executing each statement one at a time. This is like following the recipe step by step and preparing the dish.
Finally, to improve performance, the engine may compile the code into optimized machine code. This is like prepping the ingredients in advance, so you can cook the meal more quickly.
2. ECMAScript and Browser Compatibility
In addition to executing your code, the JavaScript engine is also responsible for adhering to the ECMAScript specification, which defines the syntax and semantics of the language. ECMAScript is the standard that all modern web browsers use to implement JavaScript.
However, different web browsers may have different versions of the JavaScript engine, which can lead to compatibility issues. This is like different chefs using different ingredients or following different recipes, which can result in different outcomes.
To ensure that your code is compatible with different web browsers, it's important to write code that adheres to the ECMAScript standard and to test your code on different browsers.
For example, if you want to use the let
keyword to declare a variable, make sure to include the appropriate ECMAScript version in your code, as older versions of JavaScript may not support it. And if you want your web app to work on both Chrome and Firefox, make sure to test your code on both browsers to ensure compatibility.
Performance Optimization Techniques
1. Code Profiling and Debugging
As your web app grows in complexity, you may notice that it takes longer to load and respond to user interactions. This can be due to inefficient or poorly written code.
To improve the performance of your app, you can use code profiling and debugging tools to identify and fix bottlenecks in your code. Code profiling is like taking your car to a mechanic for a tune-up, where the mechanic uses diagnostic tools to identify any performance issues. Similarly, code profiling tools can analyze your code and provide insights into which parts of your code are taking the most time to execute.
Debugging is like fixing a leaky faucet in your home. You need to identify the source of the problem and fix it to prevent any further damage. Similarly, debugging tools can help you identify errors in your code and fix them.
2. Memory Management
Another factor that can affect the performance of your web app is memory management. JavaScript uses automatic memory management, which means that the engine automatically allocates and deallocates memory as needed. However, this can sometimes lead to memory leaks, where objects are not properly deallocated, causing the app to use more memory than necessary.
To prevent memory leaks, you can use techniques such as garbage collection and memory profiling. Garbage collection is like taking out the trash in your home - you need to get rid of any objects that are no longer needed to free up space. Similarly, garbage collection in the JavaScript engine automatically removes any objects that are no longer needed to free up memory.
Memory profiling is like checking your utility bill to see how much water or electricity you're using. Similarly, memory profiling tools can help you identify which parts of your app are using the most memory and optimize them for better performance.
3. Just-In-Time (JIT) Compilation
One technique that JavaScript engines use to improve performance is Just-In-Time (JIT) compilation. This is a process where the engine compiles frequently executed code into machine code in real-time, rather than interpreting it each time it's executed.
JIT compilation is like preheating your oven before putting the dish in - it allows the engine to prepare the code in advance for faster execution. This technique can significantly improve the performance of your web app, especially for code that's executed frequently.
Components of a JavaScript Runtime
Execution Context
The execution context is like the setting in which a play is performed. It contains information such as the value of "this," the current scope chain, and the variables and functions that are available. Every time a function is called, a new execution context is created for that function. The execution context keeps track of the state of the function, such as the values of its parameters and variables, and when the function is finished executing, the context is destroyed.
Call Stack
The call stack is like a stack of plates in your kitchen. Just like you add a new plate to the top of the stack when you're washing dishes, the JavaScript runtime adds a new execution context to the top of the call stack every time a function is called. When a function is finished executing, its execution context is removed from the call stack.
The call stack is an important part of the JavaScript runtime because it determines the order in which functions are executed. If the call stack becomes too large, it can cause a stack overflow error, which can crash your web app.
Event Loop
The event loop is like a DJ at a party. Just like a DJ listens for requests from partygoers and plays the next song, the event loop listens for events and executes the corresponding code. Events can include user interactions, network requests, and timer callbacks.
The event loop is an essential part of the JavaScript runtime because it allows your web app to handle multiple tasks simultaneously without blocking the main thread. If an event requires a long-running operation, such as a network request, the event loop can delegate the task to a separate thread or process and continue processing other events.
How does a JavaScript Runtime works ?
JavaScript is a single-threaded language, which means that it can only execute one piece of code at a time. However, it often needs to perform tasks that take a long time, such as network requests or reading large files. To handle these tasks without blocking the main thread, JavaScript uses an event-driven, non-blocking I/O model.
1. Handling Asynchronous Code
Asynchronous code is code that doesn't execute immediately, but instead waits for a signal or event to occur before executing. For example, when you make a network request in JavaScript, the response doesn't come back immediately. Instead, the browser sends the request and waits for a response from the server. Meanwhile, the browser can continue executing other code.
To handle asynchronous code, JavaScript uses callbacks, promises, and async/await. Callbacks are functions that are passed as arguments to other functions and are called when a task is complete. Promises are objects that represent a task that may or may not have been completed yet, and they allow you to chain multiple asynchronous tasks together. Async/await is a newer syntax for working with promises that makes code easier to read and write.
2. Callbacks, Promises, and Async/Await
Callbacks are like leaving a note for someone to call you back when they're free. When you pass a callback function to another function, you're saying "Hey, when you're done with your task, call this function with the results." Promises are like ordering takeout from a restaurant.
When you place an order, the restaurant gives you a promise that they'll have your food ready at a certain time. When the time comes, you can pick up your food and continue with your day. Async/await is like waiting in line at the DMV. You take a ticket and wait for your number to be called. Meanwhile, you can do other things, like read a book or check your email.
3. Event-Driven Programming
Event-driven programming is like throwing a party. You invite your guests and provide food and drinks, but you don't know exactly what your guests will do. Some may dance, some may talk, and some may sit quietly. As the host, you don't control every aspect of the party, but you're prepared to handle whatever happens.
Similarly, in an event-driven programming model, the JavaScript runtime listens for events and executes the corresponding code. These events can include user interactions, network requests, or timer callbacks.
Error Handling and Exception Handling Techniques
As with any programming language, it is important to handle errors and exceptions in JavaScript. Unexpected errors can occur during the execution of a JavaScript program, such as incorrect user input, network issues, or system errors. Exception handling in JavaScript can prevent the program from crashing and provide a better user experience.
Here are some of the techniques for error and exception handling in JavaScript:
Try-Catch Statements
A try-catch statement is a block of code that is used to handle exceptions. The try block contains the code that might throw an exception, and the catch block contains the code that handles the exception. If an exception is thrown in the try block, the catch block is executed.
For example, let's say you have a function that divides two numbers:
function divide(a, b) {
try {
return a / b;
} catch (e) {
console.log("Error: " + e.message);
return null;
}
}
If the division by zero occurs, the catch block is executed, and an error message is printed to the console.
Error Objects and Stack Traces
JavaScript has built-in error objects that provide information about the type of error that occurred. The error object contains a message property that describes the error. It also has a stack property that provides a trace of the function calls that led to the error.
For example, you can use the Error object to create a custom error message:
function divide(a, b) {
if (b === 0) {
throw new Error("Cannot divide by zero");
}
return a / b;
}
If the division by zero occurs, a custom error message is thrown.
Debugging and Testing Tools
JavaScript has several tools that can help with debugging and testing. The most common debugging tool is the console. You can use console.log()
to print messages to the console and debug the program.
Practical Examples
Setting up the Development Environment
Before you start writing any code, you need to set up your development environment. This involves installing the necessary tools and software to help you write and test your code.
Step 1: Install a Text Editor or Integrated Development Environment (IDE)
To write JavaScript code, you'll need a text editor or an Integrated Development Environment (IDE). A text editor is a lightweight tool that allows you to write and edit code. An IDE, on the other hand, is a more advanced tool that includes additional features such as debugging and version control.
Some popular text editors for JavaScript development include Sublime Text, Atom, and Visual Studio Code. IDEs like WebStorm and IntelliJ IDEA are also popular choices.
Step 2: Install Node.js
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. It allows you to run JavaScript code outside of the browser, which is useful for server-side applications. You can download Node.js from the official website at https://nodejs.org.
Step 3: Set up a Package Manager
A package manager is a tool that allows you to install and manage dependencies for your project. The two most popular package managers for JavaScript are npm and Yarn.
To install npm, simply run the following command in your terminal:
npm install npm@latest -g
To install Yarn, run the following command:
npm install -g yarn
Creating and Debugging JavaScript Code
Now that you have your development environment set up, it's time to start writing some code. Let's create a simple JavaScript function and learn how to debug it.
function add(a, b) {
return a + b;
}
console.log(add(2, 3));
In this example, we've defined a function called add
that takes two parameters (a
and b
) and returns their sum. We then call the function with the arguments 2
and 3
and log the result to the console.
Step 1: Use Console.log() to Debug Your Code
One of the easiest ways to debug your JavaScript code is by using console.log()
. This method allows you to print values to the console, so you can see what's going on behind the scenes.
In the example above, we used console.log()
to print the result of the add
function to the console. This helped us verify that the function was working as expected.
Step 2: Use Breakpoints to Debug Your Code
Another way to debug your code is by using breakpoints. A breakpoint is a specific line of code that you can pause execution at, so you can inspect the state of your code at that point in time.
To set a breakpoint in your code, simply click on the line number in your text editor or IDE. When you run your code in the debugger, it will pause execution at that line and allow you to inspect the state of your code.
Handling Asynchronous Code using Promises and Async/Await
JavaScript is a single-threaded language, which means that it can only do one thing at a time. However, many operations in JavaScript are asynchronous, which means that they don't block the main thread.
Asynchronous operations include things like making HTTP requests and reading and writing to a database. To handle asynchronous code in JavaScript, we use promises and async/await.
Step 1: Understanding Promises
A promise is an object that represents the eventual completion or failure of an asynchronous operation. It has three states: pending, fulfilled, and rejected.
Here's an example of a simple promise :
const promise = new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Hello, world!');
}, 1000);
});
promise.then((result) => {
console.log(result);
});
In this example, we've defined a new promise that resolves after one second with the value "Hello, world!". We then use the then
method to handle the fulfilled state of the promise and log the result to the console.
Step 2: Using Async/Await to Handle Promises
Async/await is a more modern way to handle promises in JavaScript. It allows you to write asynchronous code in a synchronous style, which can make it easier to read and write.
Here's an example of using async/await to handle a promise:
async function getPosts() {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const data = await response.json();
return data;
}
getPosts().then((posts) => {
console.log(posts);
});
In this example, we've defined an asynchronous function called getPosts
that uses the fetch
API to make an HTTP request to the JSONPlaceholder API. We then use the json
method to extract the data from the response.
The await
keyword is used to pause the execution of the function until the promise is fulfilled. This allows us to write asynchronous code in a synchronous style.
Creating a Web Application with JavaScript
Now that you know how to set up your development environment, write and debug JavaScript code, and handle asynchronous operations, it's time to put it all together and create a web application.
Here's an example of a simple web application that uses HTML, CSS, and JavaScript to display a list of blog posts:
<!DOCTYPE html>
<html>
<head>
<title>Blog Posts</title>
<style>
.post {
margin-bottom: 20px;
}
.post h2 {
font-size: 20px;
}
.post p {
font-size: 16px;
margin-top: 5px;
}
</style>
</head>
<body>
<div id="posts"></div>
<script>
async function getPosts() {
const response = await fetch('https://jsonplaceholder.typicode.com/posts');
const data = await response.json();
return data;
}
async function displayPosts() {
const posts = await getPosts();
const container = document.getElementById('posts');
posts.forEach((post) => {
const postElement = document.createElement('div');
postElement.className = 'post';
postElement.innerHTML = `
<h2>${post.title}</h2>
<p>${post.body}</p>
`;
container.appendChild(postElement);
});
}
displayPosts();
</script>
</body>
</html>
In this example, we've defined a web page that uses CSS to style the content and JavaScript to fetch and display the blog posts.
The getPosts
function uses the fetch
API to make an HTTP request to the JSONPlaceholder API and return the data as an array of blog posts.
The displayPosts
function uses the getPosts
function to fetch the blog posts, and then uses the createElement
and appendChild
methods to dynamically create and add HTML elements to the page for each blog post.
Finally, we call the displayPosts
function to populate the web page with the blog posts.
Optimizing Performance and Memory Usage
Identifying and Fixing Performance Bottlenecks
The first step in optimizing the performance of a web application is to identify and fix performance bottlenecks. Performance bottlenecks are sections of code or areas of the application that are causing slowdowns.
To identify performance bottlenecks, you can use performance profiling tools such as Chrome DevTools. These tools allow you to analyze the performance of your application and identify sections of code that are causing slowdowns.
Once you've identified the performance bottlenecks, you can then work on fixing them. This may involve refactoring code, optimizing algorithms, or using more efficient data structures.
Minimizing HTTP Requests and Optimizing Code Loading
One of the biggest factors that affects the performance of a web application is the number of HTTP requests it makes. Each HTTP request introduces latency and can slow down the application. To minimize HTTP requests, you can use techniques such as combining multiple files into a single file, using CSS sprites, and caching resources.
Another way to optimize the performance of a web application is to optimize the loading of the code. This involves using techniques such as lazy loading, which delays the loading of non-critical code until it is needed.
Caching and Memory Management Techniques
Caching and memory management techniques can also help to improve the performance and memory usage of a web application. Caching involves storing frequently accessed data in memory or on disk to reduce the need to recalculate it.
Memory management involves optimizing the allocation and deallocation of memory to minimize the amount of memory that is being used by the application. This can involve techniques such as garbage collection and object pooling.
Analogies in everyday life can help to understand these concepts better. Think of performance bottlenecks as a traffic jam on the highway. Just as a traffic jam slows down the flow of traffic, performance bottlenecks slow down the flow of the application. To fix a traffic jam, you might need to redirect traffic or add more lanes to the highway. Similarly, to fix a performance bottleneck, you might need to optimize the code or use more efficient algorithms.
Minimizing HTTP requests is like optimizing your grocery shopping list. Just as you can save time and effort by consolidating your shopping list into one trip to the store, you can save time and effort by consolidating HTTP requests into a single request. Lazy loading is like waiting to take out your winter clothes until it gets colder. You don't need them right now, so why take them out? Similarly, lazy loading delays the loading of non-critical code until it is needed.
Caching is like having a recipe book that you use frequently. Instead of having to look up the recipe every time you need it, you can store it in memory or on disk for quick access. Memory management is like managing the space in your closet. Just as you need to optimize the space in your closet to fit all of your clothes, you need to optimize the allocation and deallocation of memory to minimize the amount of memory being used by the application.