Learn Javascript: for loop (Example)
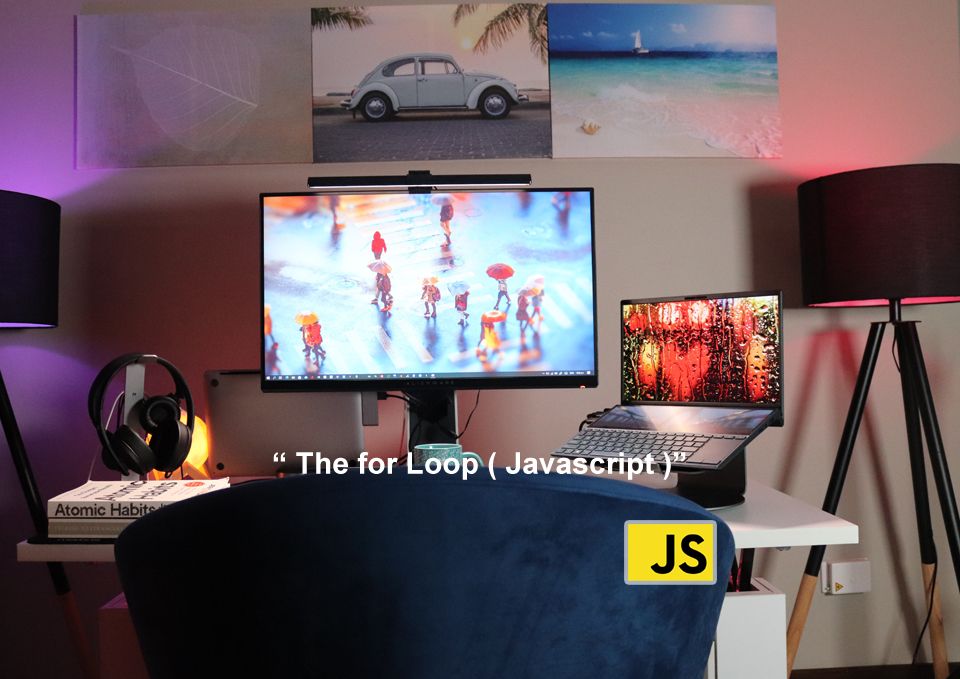
Imagine being able to repeat a task multiple times without manually doing it again and again. That's exactly what a for loop does for you in JavaScript. It's like having a tiny robot working for you, executing a set of instructions over and over until a certain condition is met.
Think of building a website and wanting to display a list of items. Instead of writing the same code for each item, you can use a for loop to generate the code for each item automatically. The for loop makes it possible to repeat a block of code a specified number of times or until a certain condition is met.
So, whether you're a beginner or a seasoned developer, the for loop is a must-have tool in your JavaScript toolbox. It makes your code more organized, efficient, and less prone to errors. Trust us, once you start using it, you'll wonder how you ever lived without it! Get ready to simplify your life with the magical world of JavaScript for loops.
JavaScript for loop syntax
"Loops, loops, and more loops!" That's the mantra of every JavaScript developer. Why? Because loops are the backbone of efficient and scalable code. And, among all the loops in JavaScript, the for loop is the king.
Here's the basic syntax of a for loop in JavaScript:
for (let i = 0; i < 10; i++) {
console.log(i);
}
Let's break down the syntax:
- The
for
keyword is followed by a set of parentheses. - Within the parentheses, we have three parts separated by semicolons:
- The first part,
let i = 0
, declares a variablei
and sets its value to 0. This variable is called the loop counter and is used to keep track of the number of iterations. - The second part,
i < 10
, is the condition that must be met for the loop to continue. In this case, the loop will continue as long asi
is less than 10. - The third part,
i++
, is the increment or decrement of the loop counter after each iteration. In this case,i
is incremented by 1 after each iteration.
- Within the curly braces, we have the code block that will be executed repeatedly as long as the condition is met.
Sample case
Consider making a straightforward to-do list application. Each job should have a checkbox that may be ticked to indicate that it has been completed when the list of tasks is displayed.
Here's how to create the code for each task using the for loop:
const tasks = ['Buy milk', 'Finish project', 'Go for a walk'];
for (let i = 0; i < tasks.length; i++) {
let task = tasks[i];
let taskItem = `<li>
<input type="checkbox">
${task}
</li>`;
document.querySelector('.tasks-list').innerHTML += taskItem;
}
4 Example Cases
Example 1: Fetching and Displaying Data from an API.
In this example, you are using an API to fetch data and display it on a website. You can use the for loop to iterate over the data and generate the HTML code for each item.
For example:
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json())
.then(data => {
for (let i = 0; i < data.length; i++) {
let post = data[i];
let postHTML = `<li>
<h3>${post.title}</h3>
<p>${post.body}</p>
</li>`;
document.querySelector('.posts-list').innerHTML += postHTML;
}
});
Example 2: Generating Dynamic Forms.
In this example, you want to generate a dynamic form based on user input. You can use the for loop to generate the HTML code for each form field.
For example:
const fields = [
{ type: 'text', label: 'Full Name' },
{ type: 'email', label: 'Email' },
{ type: 'password', label: 'Password' }
];
for (let i = 0; i < fields.length; i++) {
let field = fields[i];
let fieldHTML = `<div>
<label>${field.label}</label>
<input type="${field.type}" name="${field.label}">
</div>`;
document.querySelector('.form-fields').innerHTML += fieldHTML;
}
Example 3: Generating a Calendar.
In this example, you want to generate a calendar for a specific month and year. You can use the for loop to generate the HTML code for each day of the month.
For example:
const month = 'February';
const year = 2023;
const daysInMonth = 28;
for (let i = 1; i <= daysInMonth; i++) {
let dayHTML = `<div class="day">${i}</div>`;
document.querySelector('.calendar').innerHTML += dayHTML;
}
Example 4: Animating Elements.
In this example, you want to animate elements on a website. You can use the for loop to generate the animation code for each element.
For example:
const elements = document.querySelectorAll('.element');
for (let i = 0; i < elements.length; i) {
let element = elements[i];
element.style.animation = animate ${i + 1}s ease-in-out forwards;
}
Conclusion
To sum up, the for loop is a very strong JavaScript technique that may dramatically streamline and simplify your code. As opposed to writing out each action manually, a for loop allows you to repeat a collection of tasks repeatedly while saving you time and effort.
The for loop is a flexible tool that is certain to be very useful in your web development projects, whether you're iterating over an array of components, computing the sum of integers, or performing some other function.
Therefore, to make your life a little bit easier the next time you find yourself creating repetitious code, think about employing a for loop!
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Javascript array operations (Methods)
- Javascript Dot vs. Bracket Notation (comparison)
- Javascript: for loop (Example)