Javascript Dot vs. Bracket Notation (comparison)
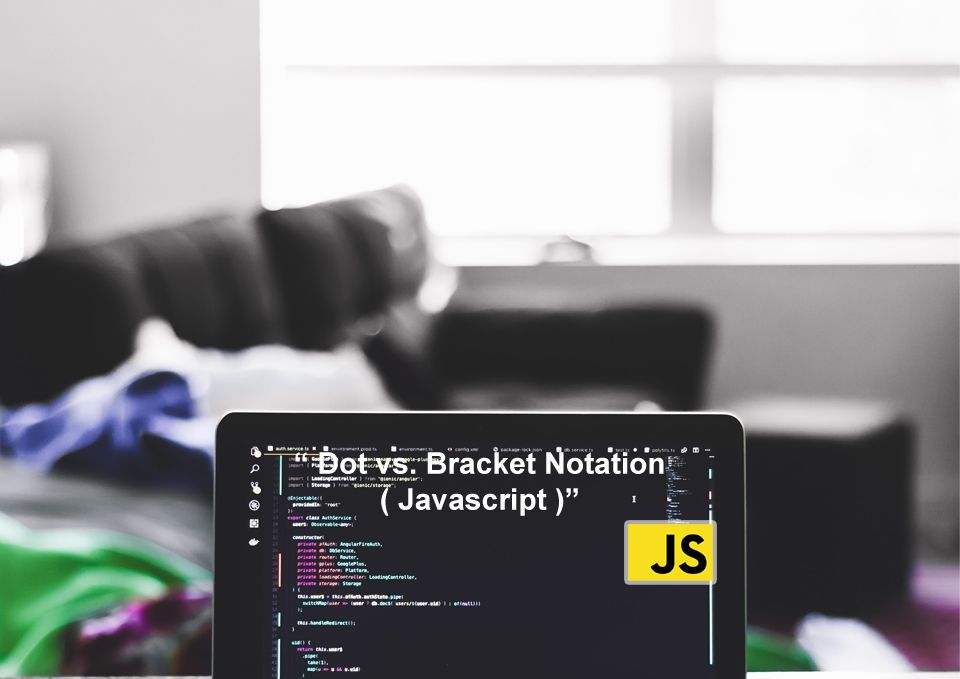
Have you ever written a code that just doesn't seem to work, only to find out later that it was a simple typo in the property name? That's why choosing the right notation in accessing object properties is crucial in JavaScript. In this article, we'll be talking about Dot vs Bracket Notation and comparison, two common ways of accessing object properties in JavaScript.
Dot Notation
How Dot Notation works in JavaScript
Suppose we have an object called "person" with properties such as "name" and "age". To access the "name" property using dot notation, we simply write: person.name
. That's it! It's that simple.
Benefits of using Dot Notation in JavaScript
- Readability: Dot Notation is easy to read and write, making your code clear and understandable.
- Less prone to errors: Since Dot Notation is straightforward, it's less likely that you'll make a typo or mistake in accessing properties.
- Ease of use: Dot Notation is the simplest way to access properties in an object, making it a great choice for beginners and experts alike.
Example of using Dot Notation in a web application
Accessing an Object Property with Dot Notation
With Dot Notation, getting to object properties is simple. Retrieving a property's value is as simple as using a period (.) before the property name.
Check out an illustration. Assume we have a "user" object that has data on users, such as their name, email address, and age. We can just write:
console.log(user.name);
Modifying an Object Property with Dot Notation
Modifying object properties with Dot Notation is just as easy as accessing them. To modify a property, you simply need to assign a new value to it.
For example, if we want to change the user's email address, we can write:
user.email = "newemail@example.com";
Let's see how we can use Dot Notation in a real-world web application
Suppose we're building a web app that displays information about a user. The user's information is stored in an object called "userInfo" with properties such as "name", "age", and "email".
We can use Dot Notation to access the user's name and display it in a header on the page like this:
const header = document.getElementById("header");
header.textContent = "Welcome, " + userInfo.name;
And if we want to allow the user to update their information, we can create a form that updates the userInfo object using Dot Notation:
const form = document.getElementById("update-form");
form.addEventListener("submit", function(event) {
event.preventDefault();
userInfo.name = form.elements.name.value;
userInfo.age = form.elements.age.value;
userInfo.email = form.elements.email.value;
});
Bracket Notation
What is Bracket Notation?
Bracket Notation is a way of accessing object properties by using square brackets ([])
instead of a period (.)
. The property you want to access is placed within the brackets, and it can either be a string literal or a variable that holds the property name.
How Bracket Notation Works in JavaScript
The beauty of bracket notation is that it enables dynamic object property access, which means you can access various properties depending on specific circumstances.
Assume, for instance, that we have an object called "user" that contains details on a user, such as their name, email address, and age. We can type the following to gain access to the user's email:
const propertyName = "email";
console.log(user[propertyName]);
This code will access the "email" property of the "user" object, even though we don't know what the property name is until runtime.
Example of Using Bracket Notation in a Web Application
- Accessing Properties with Spaces or Special Characters: Let's say you have an object with a property name that has spaces, for example:
let person = {
"full name": "John Doe"
};
To access the "full name" property, you can use bracket notation like this:
let name = person["full name"];
console.log(name); // Output: "John Doe"
- Dynamic Property Access using Variables: Another advantage of bracket notation is that you can use variables to dynamically access properties. For example:
let person = {
name: "John Doe",
age: 30
};
let key = "age";
let age = person[key];
console.log(age); // Output: 30
In this example, we stored the property name "age" in the variable key
and used it in bracket notation to access the property value.
Comparison of Dot and Bracket Notation
Pros of Dot Notation:
- Easy to read and understand: Dot notation is straightforward and easy to understand, especially for beginners. The syntax is simple and easy to remember.
- Faster to type: Because dot notation is a shorter and more concise syntax, it's faster to type compared to bracket notation.
- Error handling: If you try to access a property that doesn't exist using dot notation, JavaScript will throw an error. This can be helpful in debugging as it allows you to quickly identify what's wrong with your code.
Cons of Dot Notation:
- Limited property access: With dot notation, you can only access properties that are valid JavaScript identifier names. If you try to access a property with spaces or special characters, you'll have to use bracket notation instead.
- Inflexible: Once you declare a property using dot notation, you can't change the name of that property without modifying your code. This can be a drawback if you need to access properties dynamically based on user input or other variables.
Pros of Bracket Notation:
- Dynamic property access: Bracket notation allows you to access properties dynamically, meaning you can use a variable or expression to specify which property you want to access.
- Flexible: Bracket notation is more flexible than dot notation as it allows you to access properties with spaces or special characters.
Cons of Bracket Notation:
- More complex syntax: Bracket notation is more complex compared to dot notation, and it can be harder to read and understand, especially for beginners.
- Slower to type: Bracket notation is longer and more verbose than dot notation, making it slower to type.
So, when should you use dot notation and when should you use bracket notation? If you're accessing properties that are simple, straightforward, and don't have spaces or special characters, dot notation is the way to go. It's easier to read, faster to type, and helps with error handling. On the other hand, if you need to access properties dynamically based on user input or other variables, or if you need to access properties with spaces or special characters, bracket notation is the way to go.
Here's an example of accessing an object property
// Using dot notation
let user = {
name: "John Doe",
age: 30
};
console.log(user.name); // Output: "John Doe"
// Using bracket notation
let property = "name";
console.log(user[property]); // Output: "John Doe"
In this example, we're accessing the name property of the user object using both dot notation and bracket notation. With dot notation, we simply use the . operator to access the property. With bracket notation, we use the square bracket []
syntax, which allows us to access properties dynamically using a variable.
Conclusion
This article examined the variations between JavaScript's bracket and dot notations. We talked about what they are, how they operate, and what each one offers. We also considered instances of each notation's use and application in web applications.
Choosing the right notation for your web application is important for clarity and efficiency. Dot notation is the simpler and more straightforward option, making it a great choice for most applications. However, bracket notation is the way to go when you need to access properties with spaces or special characters, or when you want to dynamically access properties using variables.
It's essential to understand both notations and to know when to use each one. Keep in mind that both dot and bracket notation are powerful tools in your JavaScript toolkit, and using the right one can make all the difference in the success of your web application.
In conclusion, don't be afraid to experiment with both notations and see which one works best for you and your project. Whether you're a seasoned programmer or just starting out, understanding dot and bracket notation is a must-have skill in the world of web development.
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Javascript array operations (Methods)
- Javascript Dot vs. Bracket Notation (comparison)