JavaScript Arrow functions and traditional functions ( Comparison )
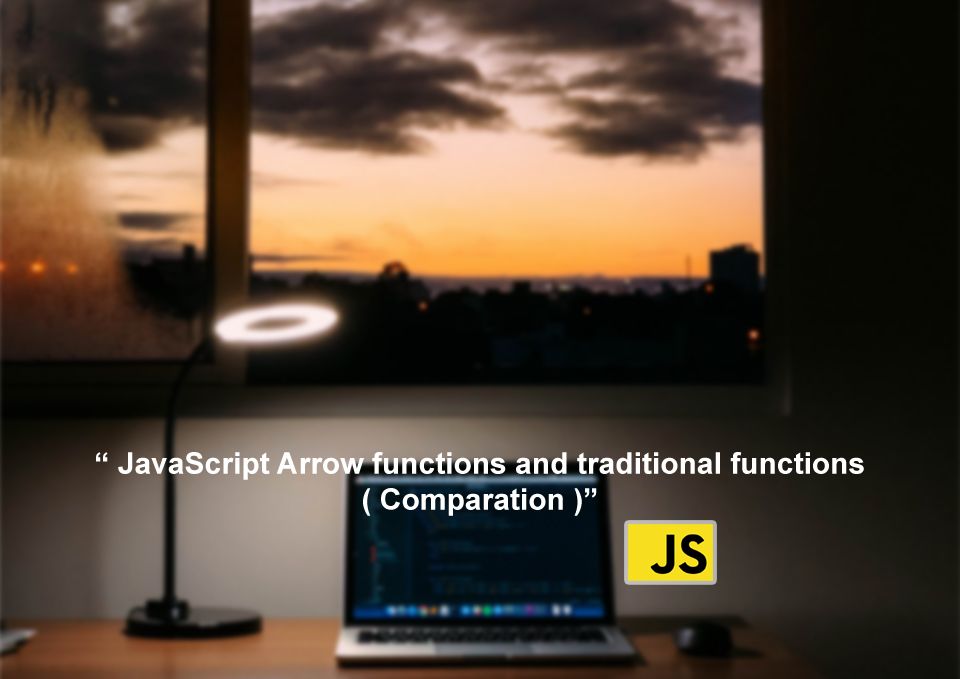
JavaScript arrow functions are a feature introduced in ES6 that allows you to create functions in a more concise way than traditional functions. They offer a clean syntax that makes code easier to read and maintain.
Syntax:
let myFunction = (arg1, arg2, ...argN) => {
statement(s)
}
or, if the function body has only one statement or expression:
let myFunction = (arg1, arg2, ...argN) => expression
Arrow Function Syntax
Arrow Function with No Arguments:
let greet = () => console.log('Hello');
greet(); // Hello
Arrow Function with One Argument:
let greet = x => console.log(x);
greet('Hello'); // Hello
Arrow Function as an Expression:
let age = 5;
let welcome = (age < 18) ?
() => console.log('Baby') :
() => console.log('Adult');
welcome(); // Baby
Multiline Arrow Functions:
let sum = (a, b) => {
let result = a + b;
return result;
}
let result1 = sum(5,7);
console.log(result1); // 12
Comparison Arrow functions and traditional functions
JavaScript code can be written using both arrow functions and conventional functions, but there are some significant distinctions between the two.
Arrow Functions:
- Possess a shorthand syntax, which can help make code shorter.
- Have a lexical this context, which means the value of this is decided by the surrounding code rather than being set by the caller;
- Implicitly return the result of an expression;
Traditional Functions:
- Offer more control and flexibility, but their syntax is more verbose.
- Require the
return
of a value with an explicit return statement. - Have a dynamic
this
context, which means that the caller chooses the value of this.
Example 1:
of an arrow function:
const add = (a, b) => a + b;
console.log(add(1, 2));
// Output: 3
of a traditional function:
function add(a, b) {
return a + b;
}
console.log(add(1, 2));
// Output: 3
When building a web app, both arrow functions and traditional functions can be used.
Example 2:
For example, in an event listener for a button click, you might use an arrow function to handle the click event:
const button = document.querySelector('button');
button.addEventListener('click', () => {
console.log('Button was clicked!');
});
On the other hand, you might use a traditional function to define a utility function that's used throughout your app:
function formatDate(date) {
return date.toLocaleDateString();
}
console.log(formatDate(new Date()));
// Output: "2/6/2023" (or similar, depending on the locale)
Transforming traditional functions into arrow functions
Here are three examples of transforming traditional functions into arrow functions:
Example 1:
// Traditional function
function multiplyByTwo(num) {
return num * 2;
}
// Arrow function equivalent
const multiplyByTwo = num => num * 2;
Example 2:
// Traditional function
function concatenateStrings(str1, str2) {
return str1 + str2;
}
// Arrow function equivalent
const concatenateStrings = (str1, str2) => str1 + str2;
Example 3:
// Traditional function
function sayHello(name) {
return "Hello " + name;
}
// Arrow function equivalent
const sayHello = name => "Hello " + name;
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- JavaScript Arrow functions and traditional functions