Javascript array operations (Methods)
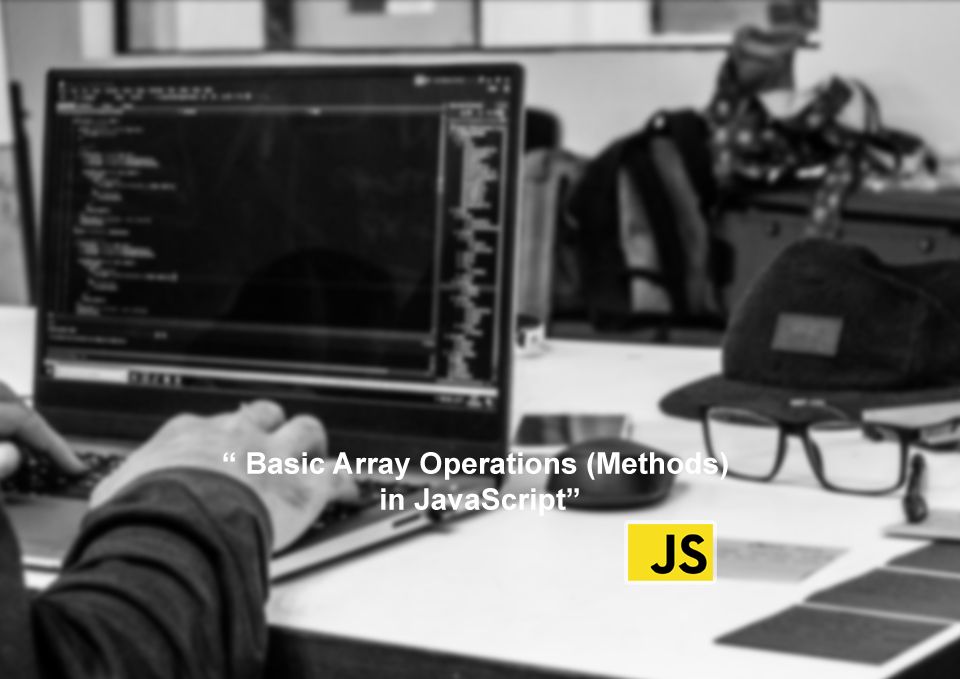
An array is simply a list of items. It could be a list of numbers, strings, objects, or any other data type. The items are stored in an ordered manner and can be easily accessed and manipulated. In JavaScript, arrays are created using square brackets [].
JavaScript has a set of useful array methods that can make data manipulation a breeze. From adding elements to an array to sorting and removing items, there is an operation for almost anything you might need to do with a JavaScript array. Whether it's checking if an element exists in the array or shuffling the items around, basic array operations will help you accomplish your tasks quickly and easily.
Basic Array Methods
push() - adding elements to the end of an array
To add one or more elements to the end of an array, use the push()
method.
It's like adding one extra candy bar to your candy jar; it makes your array sweeter.
let candies = ['gummies', 'lollipops', 'chocolate'];
candies.push('jelly beans');
console.log(candies);
// Output: ['gummies', 'lollipops', 'chocolate', 'jelly beans']
pop() - removing elements from the end of an array
We can take elements out of an array in the same way that we can add them.
Similar to plucking the last piece of candy out of your candy jar, the pop()
method removes the last element of an array.
candies.pop();
console.log(candies);
// Output: ['gummies', 'lollipops', 'chocolate']
shift() - removing elements from the beginning of an array
The shift()
method works similarly to the pop()
method, except it removes the first element of an array instead of the last.
candies.shift();
console.log(candies);
// Output: ['lollipops', 'chocolate']
unshift() - adding elements to the beginning of an array
The unshift()
method is used to add elements to the beginning of an array, similar to the push()
method but for the start of the array.
candies.unshift('gummies');
console.log(candies);
// Output: ['gummies', 'lollipops', 'chocolate']
indexOf() - finding the index of an element in an array
You might occasionally need to know the array element's index.
You can do that by using the indexOf()
method.
let index = candies.indexOf('chocolate');
console.log(index);
// Output: 2
slice() - extracting a portion of an array
By using the slice()
method, you can take a subset of an array and return it as a brand-new array. This is helpful if you wish to divide an array into sections for different uses.
let chocolates = candies.slice(2);
console.log(chocolates);
// Output: ['chocolate']
splice() - adding or removing elements from a specific index
The splice()
method is like a combination of push()
, pop()
, shift()
, and unshift()
. It allows you to add or remove elements from a specific index in an array.
candies.splice(1, 0, 'jelly beans');
console.log(candies); // Output: ['gummies', 'jelly beans', 'lollipops', 'chocolate'];
candies.splice(1, 1);
console.log(candies);
// Output: ['gummies', 'lollipops', 'chocolate']
Advanced Array Methods
map() - transforming each element in an array
This method allows you to "map out" a transformation for each element in an array. It's like having a GPS for your data, guiding it from point A
to point B
.
For example, let's say you have a list of numbers and you want to square each one. The map()
method makes this a piece of cake:
const numbers = [1, 2, 3, 4, 5];
const squaredNumbers = numbers.map(num => num * num);
console.log(squaredNumbers);
// Output: [1, 4, 9, 16, 25]
filter() - filtering elements based on a condition
This method is like a filter for your data, allowing you to select only the elements that meet certain conditions.
For example, let's say you have a list of temperatures and you only want to keep the ones that are above freezing. The filter()
method to the rescue!
const temperatures = [32, 45, 12, 67, -5];
const aboveFreezing = temperatures.filter(temp => temp > 32);
console.log(aboveFreezing);
// Output: [45, 67]
reduce() - reducing an array to a single value
This method reduces your array to a single value, like reducing a complex equation to a simplified answer.
For example, let's say you have a list of expenses and you want to find the total cost. The reduce()
method makes this a snap:
const expenses = [50, 100, 75, 200];
const totalCost = expenses.reduce((accumulator, expense) => accumulator + expense, 0);
console.log(totalCost);
// Output: 425
Conclusion
For organizing data in your web apps, JavaScript arrays can be a game-changer. The key to maximizing the use of arrays is to become an expert in basic array operations. Several important methods, including push, pop, shift, unshift, indexOf, slice, splice, map, filter, and reduce, were covered in this article.
These techniques can be used to add or remove elements from arrays, find the index of an element, extract a section of an array, transform each element in an array, conditionally filter elements, and reduce an array to a single value.
It is crucial to highlight that mastering these fundamental array methods in JavaScript can make managing data for your websites much easier. It's similar to having a toolbox full of useful tools at your disposal to handle any task that arises.
So don't be hesitant to delve into the world of arrays and begin learning about these techniques. By mastering them, you will become a more capable and effective developer in addition to making your coding journey easier.
As always, practice makes perfect. So, keep playing around with these methods and see what you can create. And remember, there is always more to learn, so never stop exploring and growing your skillset. "The sky's the limit when it comes to what you can do with arrays in JavaScript!".
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Javascript array operations (Methods)