How To Use Object Methods in JavaScript (Example)
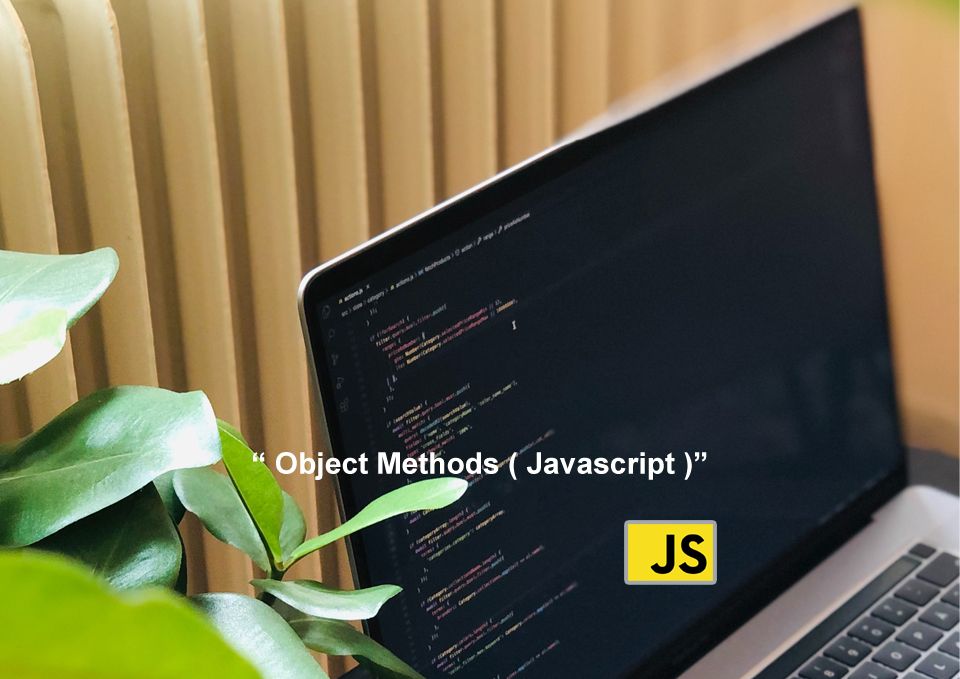
In JavaScript, objects are made up of key/value pairs. These values can be properties and methods, and can contain all types of JavaScript data, like strings, numbers, and booleans. All objects in JavaScript come from the Object constructor, which has many useful built-in methods.
This tutorial will cover the main built-in object methods in JavaScript and give examples of how to use them.
Object.create()
First, let's look at the Object.create()
method.
The prototype of an object, which you can create using this method, inherits from another object. Creating multiple objects that share the same properties and methods is a great way to build reusable objects.
Let's take the example of wanting to create an object for a person with the properties of name and age. These properties can be used to create a prototype object, which you can then use the function Object.create()
to create additional objects that descended from it:
let personProto = {
name: "",
age: 0
};
let person1 = Object.create(personProto);
person1.name = "John";
person1.age = 30;
let person2 = Object.create(personProto);
person2.name = "Jane";
person2.age = 25;
Object.keys(), Object.values(), and Object.entries()
You can examine an object's properties using the methods Object.keys()
, Object.values()
, and Object.entries()
.
Object.entries()
returns an array of key-value pairs.Object.keys()
returns an array of the object's keys.Object.values()
returns an array of the object's values.
For example:
let person = {
name: "John",
age: 30
};
console.log(Object.keys(person)); // Output: [ "name", "age" ]
console.log(Object.values(person)); // Output: [ "John", 30 ]
console.log(Object.entries(person)); // Output: [ [ "name", "John" ], [ "age", 30 ] ]
Object.assign()
Merging two or more objects into one new object is possible with the Object.assign()
function. This is a fantastic method for adding properties from several sources to an object.
For example:
let person = {
name: "John",
age: 30
};
let job = {
title: "Developer",
salary: 100000
};
let combined = Object.assign({}, person, job);
console.log(combined); // Output: { name: "John", age: 30, title: "Developer", salary: 100000 }
Object.freeze() and Object.seal()
The Object.freeze()
and Object.seal()
methods allow you to manage an object's behavior.
Object.freeze()
makes an object immutable, which means that its attributes and methods cannot be altered.Object.seal()
the method prevents new properties from being added to an object while allowing current properties to be updated.
As an example:
let person = {
name: "John",
age: 30
};
Object.freeze(person);
person.age = 31; // This will have no effect
let sealedPerson = Object.seal(person);
sealedPerson.address = "123 Main St"; // This will have no effect
sealedPerson.age = 31; // This will change the value of `age`
Conclusion
Finally, these JavaScript Object methods give us powerful capabilities for manipulating and working with objects in online applications.
These methods enable us to accomplish everything from creating a new object to accessing its attributes and values, assigning new properties to it, and even preventing modifications from being done.
It's vital to remember that each of these methods has its own distinct use case, and the one we employ will be determined by the needs of the project we're working on.
For example, Object.freeze
would be used to construct an object that cannot be changed. However, we would use Object.create
to create a new object that inherits properties from an existing object ().
In summary, these methods enable us to interact with objects in a variety of ways and are an essential component of the JavaScript language that every web developer should be familiar with. Therefore, let's get started using them in our projects!
"It's all about unlocking the power of objects and giving them the control they deserve!"
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Javascript array operations (Methods)
- Javascript Dot vs. Bracket Notation (comparison)
- How To Use Object Methods in JavaScript (Example)