Functions Calling Other Functions in JavaScript
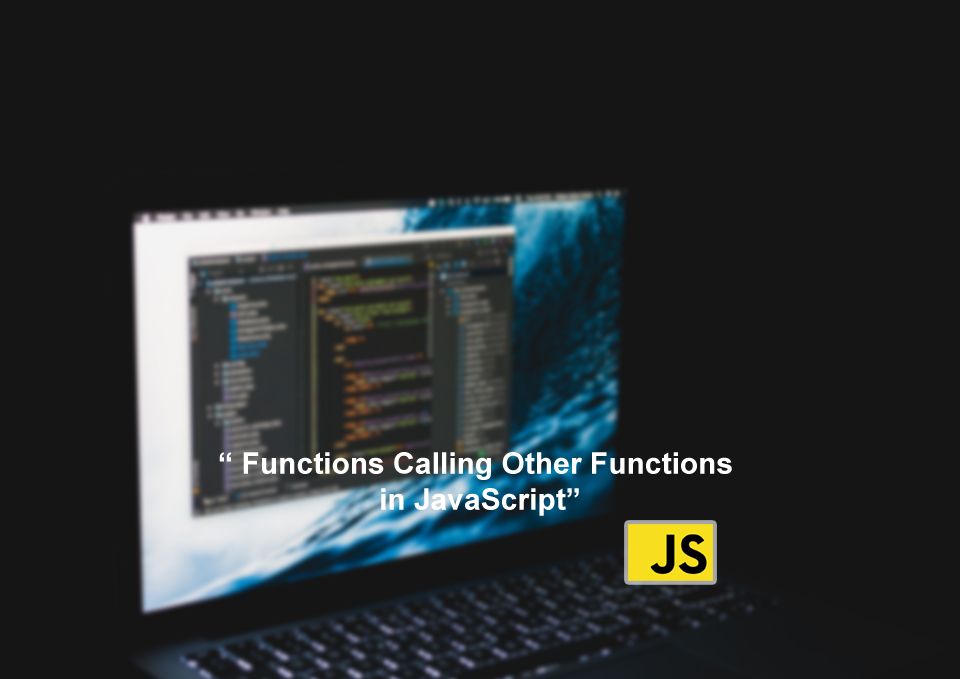
When we execute a function by utilizing its name end closed in parenthesis, this is known as function calling
. For instance, we can use greet()
it to execute a function with the name greet
that outputs a message.
A function can also accept arguments, which are values that it can utilize to carry out its function. A function that adds two numbers, for instance, may accept two parameters and output the sum.
Calling, passing, and returning
Functions in JavaScript are the backbone of any successful web application.
How to call a function
In JavaScript, calling a function is straightforward. Simply place the function's name where you want it to be executed in your code by knowing its name.
For example:
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet("John");
The function "greet" has been declared in the example above, and it accepts the parameter "name". We simply provide the function's name (in this case, "greet") and the necessary parameter to call the function ("John"). The greeting will then be recorded on the console when the function runs.
Passing parameters to a function
Functions can take in parameters, which are values that are passed to the function when it is called. These parameters can be used within the function to perform specific tasks.
For example:
function greet(name) {
console.log(`Hello, ${name}!`);
}
greet("Jane");
greet("John");
greet("Jim");
In this example, we are calling the "greet" function three times, each time passing in a different name as a parameter. The function will then run and log the appropriate greeting to the console for each name.
Returning values from a function
Values may also be returned by functions. To specify the value that a function should return, use the return statement.
function square(num) {
return num * num;
}
const result = square(2);
console.log(result);
In this example, we've declared a function called square
that accepts the input num
. Using the return
statement, the function computes the square of the inputted value and then returns the result. The result is then logged to the console and saved in a variable called result.
Function calling another function in a simple web app
For example we're developing a web application standard:
- To determine the total cost of the products in a shopping cart.
- Each item's price is determined by one function, while the total is determined by another.
- We can call the function that determines the price of each item rather than writing for each item the function that determines the total.
function calculateItemPrice(item, quantity) {
return item.price * quantity;
}
function calculateTotalPrice(cart) {
let total = 0;
for (let i = 0; i < cart.length; i++) {
let item = cart[i];
total += calculateItemPrice(item, item.quantity);
}
return total;
}
Function calling to create more complex web applications
Let's take the example of building a web application that creates reports depending on user data. The ability to develop functions for data retrieval, data processing, and report generation is available. You may create a complicated application that is simple to maintain and update by invoking these methods in the right order.
function retrieveData(userId) {
// code to retrieve data from a database
return data;
}
function processData(data) {
// code to process data and prepare it for the report
return processedData;
}
function generateReport(processedData) {
// code to generate the report
return report;
}
function generateReportForUser(userId) {
let data = retrieveData(userId);
let processedData = processData(data);
let report = generateReport(processedData);
return report;
}
Conclusion
In conclusion, function calling is a fundamental technique in web app development that allows developers to create reusable and maintainable code. By breaking down complex tasks into smaller functions and calling them in the right order, developers can simplify their code and build more complex applications. So the next time you start a new web project, remember the power of function calling and how it can make your life easier!
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Functions Calling Other Functions in JavaScript