Conditional (Ternary) Operator in javascript
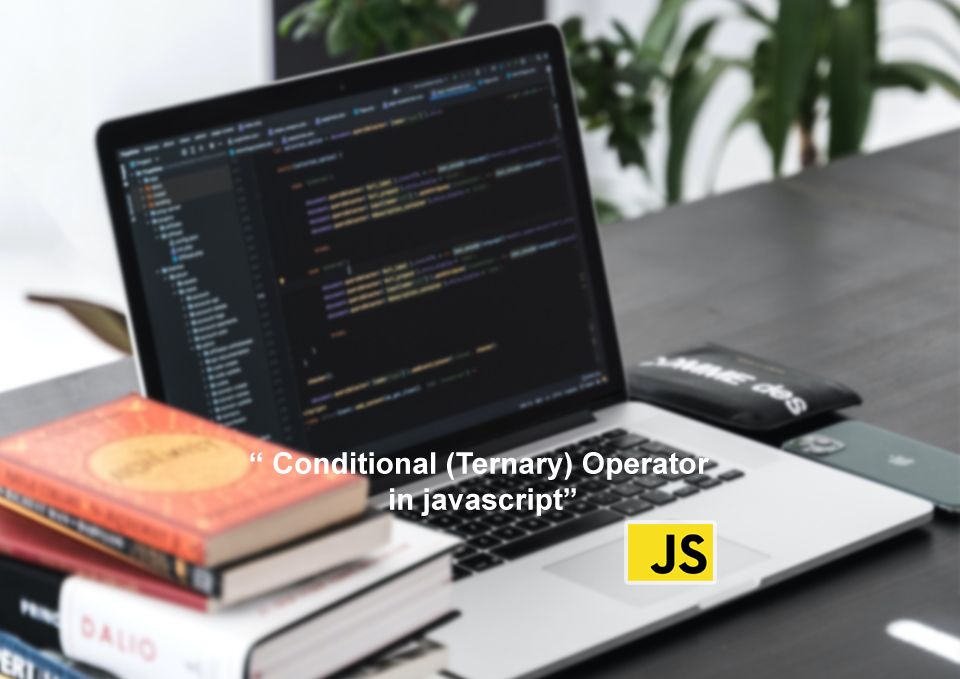
The conditional (ternary) operator in JavaScript is a compact and elegant way of doing "if-else" operations. It's like having a mini decision machine in your palm! Instead of writing lengthy if-else statements, you can express the same logic in one line Ternary operator. This is especially useful for making quick and easy decisions in your code, giving you more room to breathe and simplify your code.
What is the conditional (ternary) operator and why would you use it?
Have you ever been in a situation when writing code where you had to make a decision based on a certain condition and then return a value based on whether that condition was true or false? Well, my friend, you are in luck! satisfies the conditional (ternary) operator a compact and elegant solution for all your Decision requirements in JavaScript.
But what exactly is the ternary operator? Simply put, it's a shorthand for an if-else
statement that allows you to express the same logic in one line. The syntax is easy to remember and use: condition? value1:value2
. Returns value1 if the condition is true, if false Returns a value of 2.
So why use the ternary operator instead of a traditional if-else statement? First, it's cleaner and saves space, which is especially useful when you just need to make a quick decision and don't want the code to look cluttered. Second, it adds a bit of individuality and elegance of your code make it stand out from the usual if-else
statements.
How does the conditional (ternary) operator work in JavaScript?
Let's dig a little deeper into an example and see how the ternary operator works. Suppose you're building a web application that displays a message to the user based on the time of day. Using the ternary operator, you can write the following:
let time = 12;
let message = time >= 12 ? "Good afternoon!" : "Good morning!";
console.log(message);
It's important to note that the ternary operator only works on one line of code. If you need to perform multiple actions based on certain conditions, it is better to use if-else statements instead.
Comparison of conditional (ternary) operators with if-else statements.
Decision-making in programming can be a real headache. With so many options to choose from, which one is best for your code? Conditional (ternary)
operators and if-else
statements are two of the most commonly used decision-making tools in code, but which one should you use? That's what we're going to explore today.
Let's start with the ternary operator. This operator is a shorthand way of writing simple if-else
statements. It is written like this:
condition ? value1 : value2
Evaluates the condition and returns value1 if true, and value2 if false. It's perfect when you need to make a quick decision and return a value.
Now let's look at the if-else
statement. This statement allows you to write multiple lines of code based on certain conditions. It is written like this:
if (condition) {
// code to run when the condition is true
} else {
// code to run when the condition is false
}
if-else statements are great for situations where you need to perform multiple actions based on certain conditions. It also applies to more complex decision-making processes.
So which one should you use? It depends on the actual situation. If you need to make a simple decision and return a value, the ternary operator is the way to go. However, if you need to perform multiple actions or have a more complex decision-making process, an if-else
statement is a better choice.
Best practices and common mistakes to avoid when using the conditional (ternary) operator.
Best Practices:
- Keep it simple: The
ternary
operator is great for simple decisions, so try to keep your conditions and return values ​​simple and straightforward. If your conditions become too complex, it's better to useif-else
statements instead. - Make it readable: When using the ternary operator, make sure your code is easy to read and understand. Add comments with proper indentation if needed, and consider breaking complex expressions into separate variables.
- Use parentheses: Adding parentheses around your conditions can help improve readability and avoid confusion. It also helps prevent errors like type coercion.
Common Mistakes to Avoid:
- Does not return a value: The ternary operator is used to return a value, so make sure that both branches of the expression return a value.
- Use unnecessary conditions: Do not add unnecessary conditions to the ternary operator. This can clutter the code and make it harder to maintain in the future.
- No parentheses: If your value1 or value2 expressions span multiple lines, make sure to enclose them in parentheses.
Let's look at an example to see these best practices and mistakes in action:
// Good example
let age = 25;
let message = (age >= 21) ? 'Welcome to the party!' : 'Sorry, you are not old enough.';
// Bad example
let score = 80;
(score > 60) ? console.log('Passed!') : console.log('Failed!');
// This is a mistake because console.log doesn't return a value
// Good example
let score = 80;
let result = (score > 60) ? 'Passed!' : 'Failed!';
console.log(result);
// Bad example
let num = 10;
let output = (num === 10) ? 'Ten' : (num === 20) ? 'Twenty' : 'Neither';
// This is a mistake because it's too complex and hard to read
// Good example
let num = 10;
let output;
if (num === 10) {
output = 'Ten';
} else if (num === 20) {
output = 'Twenty';
} else {
output = 'Neither';
}
Practical application of the conditional (ternary) operator.
If you're a JavaScript developer, you've probably come across the conditional (ternary) operator. This is a compact and efficient way to execute simple conditional statements. But have you ever wondered how this little operator can simplify your code and save you time in real-world applications?
Let's dive into some everyday use cases where the ternary operator can simplify your code.
Toggling CSS Classes:
Say you're building a weather app and want to display different icons based on temperature. Instead of using if-else statements, you can use the ternary operator to toggle CSS classes with a single line of code.
const temperature = 72;
const weatherClass = temperature >= 70 ? 'sunny' : 'cloudy'; document.body.classList.add(weatherClass);
Shortening return statements:
Suppose you have a function that returns a value based on a certain condition. You can use the ternary operator to shorten return statements and make them easier to read.
function getDiscount(isPremium) {
return isPremium ? 0.15 : 0;
}
const discount = getDiscount(true); console.log(`Discount: ${discount}`);
Setting default values:
You can set a default value for a variable using the ternary operator. This is especially useful when working with data that may not always be available.
const username = userData.username || 'Guest';
console.log(`Welcome ${username}!`);
Conditional Rendering:
In React, you can conditionally render elements using the ternary operator. This saves you time and makes your code more readable.
const isLoading = true;
return (
<div>
{isLoading ? <p>Loading...</p> : <p>Data loaded successfully!</p>} </div>
);
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Understanding JavaScript Template Literals (Template Strings)
- Exploring Falsy & Truthy Values: A Comprehensive Guide
- Equality Operators: == vs. ===
- Understanding JavaScript Boolean Logic: A Beginner's Guide
- JavaScript Comparison and Logical Operators (Beginner's Guide)
- JavaScript switch Statement (Beginner Guide)
- JavaScript Expressions and Statements (Beginner Guide)
- Conditional (Ternary) Operator in javascript