Understanding If Else Statements in JavaScript Fundamentals
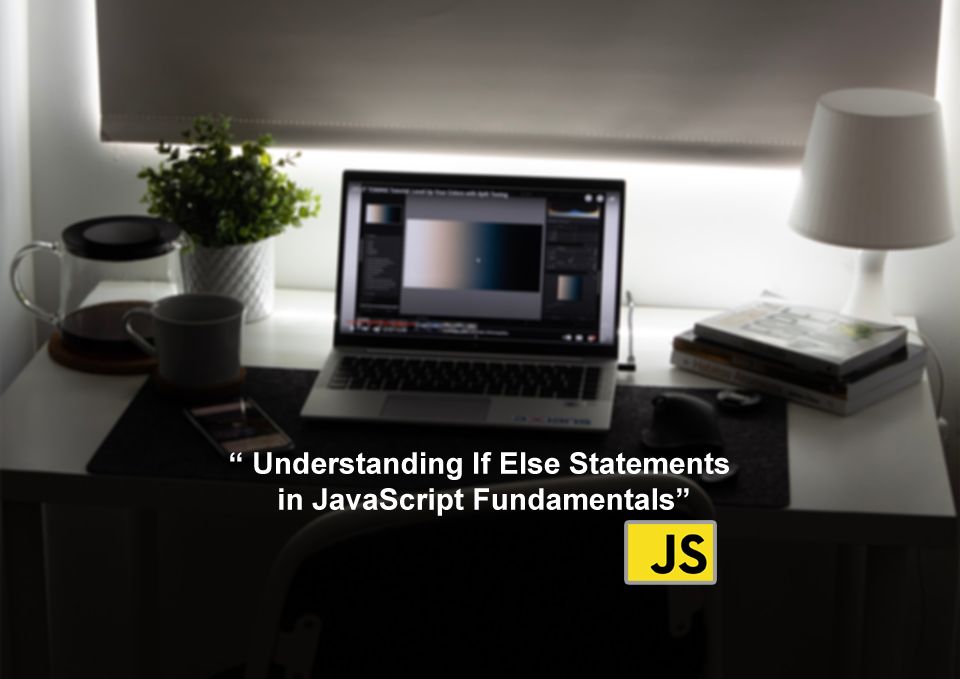
In computer programming, situations may arise where you have to run a piece of code among several alternatives. For example, assign grades A B or C based on the grades a student earns.
In this case, you can use JavaScript if...else statements to create programs that make decisions.
In JavaScript, there are three forms of if...else statements.
- if statement
- if else statement
- if else if else statement
JavaScript if Statement
The syntax of the if statement is:
if (condition) { // the body of if}
The if statement evaluates the conditions enclosed in parentheses ().
- The code inside the body of the if is executed if the condition evaluates to true.
- The code inside the body of the if is skipped if the condition evaluates to false.
Note: The code inside { } is the body of the if statement.
Example if Statement
// check if the number is positive
const number = prompt(Enter a number: );
// check if number is greater than 0
if (number > 0) {
// body of the if statement console.log(The number is positive);
}
console.log("The if statement is easy");
Suppose the user enters -1 . In this case, the condition number > 0 evaluates to false . So the body of the if statement is skipped.
Since console.log(if statement is simple); outside the body of the if statement, it is always executed.
Use comparison and logical operators in conditions. So, to learn more about comparison and logical operators, you can visit JavaScript Comparison and Logical Operators.
JavaScript if else statement
An if statement can have an optional else clause. The syntax for an if else statement is:
if (condition) {
// code block when the condition is true
} else {
// code block if condition is false
}
The if else statement evaluates the conditions inside the parentheses.
Example if else Statement
// check if the number is positive or negative/zeroconst
number = prompt(Enter a number: );
// check if number is greater than 0
if (number > 0) {
console.log(number is positive);
}
// if number is not greater than 0
else {
console.log(the number is negative or 0);
}
console.log (simple if...else statement);
Suppose the user enters -1 . In this case, the condition number > 0 evaluates to false . Therefore, the body of the else statement is executed, while the body of the if statement is skipped.
JavaScript if else if statement
The if else statement is used to execute a piece of code between two alternatives. However, if you need to choose between more than two alternatives, you can use if else if else.
if else The syntax of the if else statement is:
if (condition1) {
// code block 1
} else if (condition2){
// code block 2
} else {
// code block 3
}
- If condition1 evaluates to true, code block 1 is executed.
- If condition1 evaluates to false, condition2 is evaluated.
- If condition 2 is true, code block 2 is executed.
- If condition 2 is false, code block 3 is executed.
Example if else if Statement
// check if the number is positive or negative or zeroconst
number = prompt(Enter a number: );
// check if number is greater than 0
if (number > 0) {
console.log(number is positive);
}
// check if number is 0
else if (number == 0) {
console.log(number is 0);
}
// if the number is neither greater than 0 nor zero
else {
console.log(is a negative number);
}
console.log(if...else if...else statement is simple);
Assuming user input then the first test condition number > 0 evaluates to false . Then the second test condition number == 0 evaluates to true and executes its corresponding block.
Nested if else Statement
You can also use if else statements within if else statements. This is called nested if else statements.
Example Nested if else Statement
// check if the number is positive or negative or zeroconst
number = prompt(Enter a number: );
if (number >= 0) {
if (number == 0) {
console.log(you entered the number 0);
} else {
console.log(you entered a positive number);
}
} else {
console.log(you entered a negative number);
}
Suppose the user enters 5 . In this case, the condition number >= 0 evaluates to true and the control of the program enters the outer if statement.
The test condition number == 0 of the inner if statement is then evaluated. Since it is false, the else clause of the inner if statement is executed.
Note: As you can see, nested if else complicates our logic and we should avoid nested if else as much as possible.
The body of if else has only one statement
If the body of if else has only one statement, we can omit {} in the program. For example you can replace
const number = 2;if (number > 0) {
console.log(Number is positive.);
} else {
console.log(The number is negative or zero.);
}
with
const number = 2;if (number > 0)
console.log(Number is positive.);
else
console.log(Number is negative or zero.);