Operator Precedence: The Basics of the Javascript Fundamentals
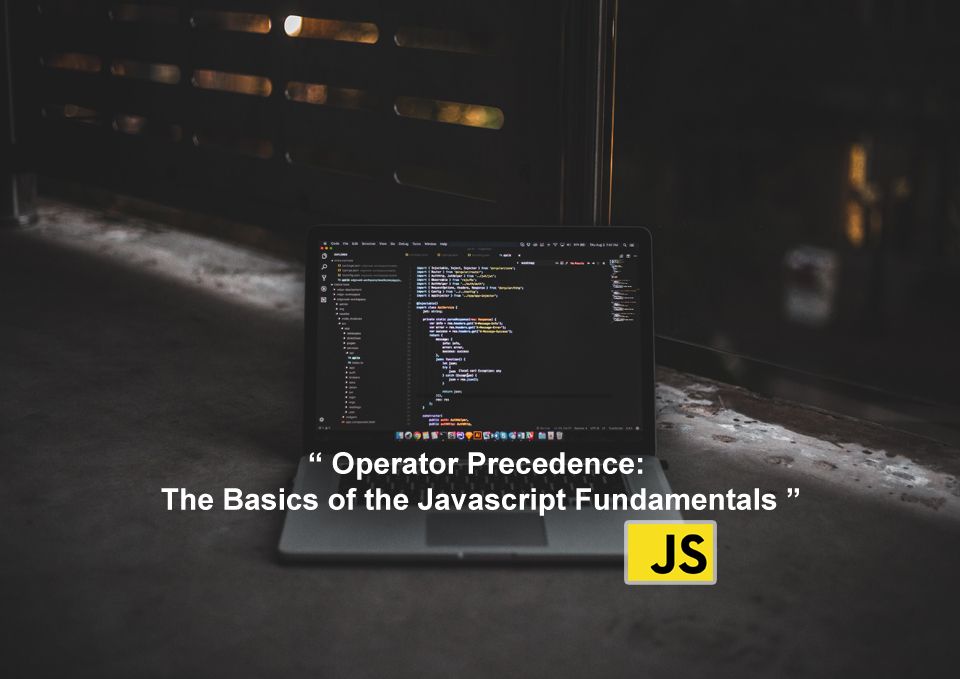
- Javascript Fundamentals #Data Type in Javascript
- Javascript Fundamentals #Values & Variables
- Javascript Fundamentals #JavaScript Data Types
- Javascript Fundamentals #when to use const vs let vs var
- Javascript Fundamentals #Basic Operators
- Operator Precedence: The Basics of the Javascript Fundamentals
Are you starting to learn JavaScript but feeling a bit overwhelmed? Not to worry – this blog post is here to help! In it, we’ll explore the basics of operator precedence and how it can help you understand the fundamentals of JavaScript. Keep reading to get started!
What is Operator Precedence?
Operator Precedence is a rule in JavaScript that determines the order in which operators should be executed when performing a certain mathematical operation. By setting a hierarchy of operators, it determines which operations have higher priority than others and therefore resolves the sequence of operations to be executed.
Operators with higher precedence will become the operands of operators with lower precedence. This means that the operator with higher precedence is evaluated first in an operation.
If you change a simple operator inside of an operation, it can affect the outcome. Without knowing operator precedence, it’s a mistake to try and create a complex operation.
Operator precedence, also known as “order of operations,” are rules widely used in both mathematics and computer programming. They are broken out into this order: Parentheses, Exponents, Multiplication/Division, and Addition/Subtraction. This means that parentheses are evaluated first come first, and addition/subtraction
The Basics of Operator Precedence
Operator precedence is a concept used in mathematics and computer programming to determine the order in which operations are performed. It is based on the "My Dear Aunt Sally" mantra (multiply, divide, add, subtract) for familiar arithmetic operations.
In JavaScript, operator precedence determines the order in which operations are evaluated in a complex expression. Operators are categorized by their tasks, such as arithmetic, comparison, or assignment. Operators with higher precedence are evaluated first.
For example :
1 + 2 * 3 is treated as 1 + (2 * 3), whereas 1 * 2 + 3 is treated as (1 * 2) + 3
the grouping operator has the highest precedence, while addition and subtraction have the same precedence. JavaScript developers generally use parentheses to control the order of the operation. In other words, operators with the same precedence are evaluated according to their position in the expression.
Knowing the operator precedence, helps developers understand the order in which operations are evaluated and avoid unexpected errors. The operator precedence is an important concept for any JavaScript developer to understand.
Left-to-right and Right-to-left Associativity
Left-to-right and Right-to-left Associativity are two ways of grouping operators in an expression. Left-to-right associativity means that the operands are parsed from left to right, while Right-to-left associativity means that the operands are parsed from right to left. This is important for operators with the same precedence because it determines the order in which the expression is evaluated.
For example, if you have two multiplication operators with the same precedence, the left-to-right associativity will parse them as (M1*M2) instead of (M2*M1). Similarly, if two additional operators have the same precedence, the left-to-right associativity will parse them as (A1 A2) instead of (A2 A1).
You can also use parentheses to override the default operator associativity rules. Most Java operators are left-to-right associative, although there are a few exceptions; for example, the unique exponentiation operator has right associativity.
10 + 20 + 30
// Left-to-right associativity would be:
(10 + 20) + 30
// then:
30 + 30
10 + 20 + 30
// Right-to-left associativity would be:
10 + (20 + 30)
// then:
30 + 30
Grouping
The Grouping operator in JavaScript, represented by parentheses (()), has the highest precedence of all operators. It is used to override the normal operator precedence, allowing developers to control the order of their operations.
For example, if a group of operators have different precedence, the operator with the highest precedence will be evaluated first. This ordering is important for ensuring accurate results in arithmetic calculations or expressions. Additionally, the grouping operator acts as a container for arbitrary expressions, making it a useful tool for JavaScript developers.
a && (b + c)
2 + 8 + 9 + (10 - 5)
//nesting parentheses.
(2 + 2) + ((9 - 5) - 2)
Logical Operators
In JavaScript, operator precedence determines the order in which operators are evaluated. Operators with higher precedence are evaluated first. The logical NOT operator, !, is always evaluated first. Following that, the arithmetic operators are evaluated, followed by the comparison operators. The logical operators && (AND) and || (OR) are evaluated last.
When evaluating operators with the same precedence, the expression is evaluated from left to right. For example, if a group of operators with the same precedence are used in a boolean comparison, the result will be a boolean value.
if (20 === number || 10 === number) return true;
Four logical operators
! — NOT
&& — AND
|| — OR
?? — Nullish Coalescing
Example
- logical NOT (!) :
const bool = true;
if (!bool) {
console.log("false!");
} else {
console.log("true!");
}
// 'true!'
const arr = ["operator", "precedence"];
if (!arr) {
console.log("false!");
} else {
console.log("true!");
}
// 'true!'
- logical AND (&&)
const bool = true;
const arr = ["operator", "precedence"];
if (arr && bool) {
console.log("true!");
} else {
console.log("false!");
}
// 'true!'
if (arr && bool && 1 > 2) {
console.log("true!");
} else {
console.log("false!");
}
// 'false!'
- logical OR (||)
const bool = true;
const arr = ["operator", "precedence"];
if (arr || 1 > 2) {
console.log("true!");
} else {
console.log("false!");
}
// 'true!'
if (1 > 2 || 2 > 3) {
console.log("true!");
} else {
console.log("false!");
}
// 'false!'
- nullish coalescing operator (??)
const bool = null ?? true;
console.log(bool);
// 'true'
const boool = 1 ?? true;
console.log(boool);
// '1'