Python Functions: What is a return statement
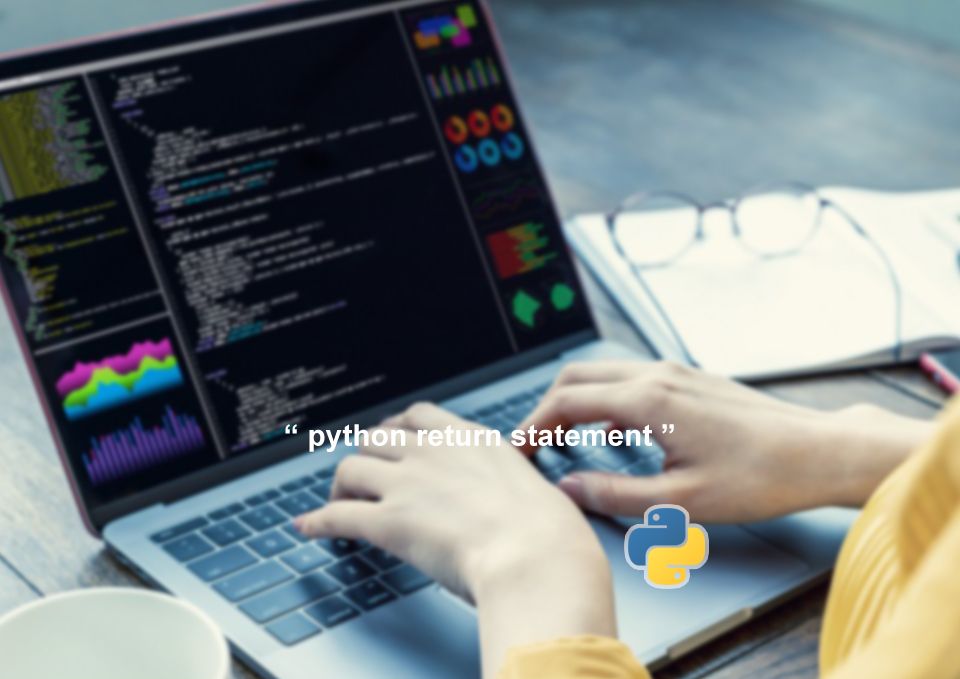
Python functions are one of the most important concepts in Python programming. They are blocks of code that perform a specific task and can be called from anywhere in the program. A return statement is a statement that is used to return a value or values from a function.
Understanding the concept of the return statements is essential in Python programming as it helps in building more complex programs. This article will explore the concept of return statements in Python functions and how it can be applied in making a web app.
Understanding Python Functions
Code can be condensed into a single block that can be utilized numerous times by using functions. They are declared by using the def keyword, the function name, and parentheses around the arguments.
When the function is called, the code section is then indented and run.
Here's an example of a function that takes two arguments and returns their sum:
def add_numbers(num1, num2):
sum = num1 + num2
return sum
In the above code, we defined a function add_numbers
that takes two arguments num1
and num2
. The function then adds the two numbers and returns their sum using the return
keyword.
Importance of Return Statement
As it enables the development of more complex programs, the return statement is a crucial idea in Python programming. It enables us to apply a function's output to another area of the software.
Types of Return Statements
There are three types of return statements in Python:
- Return statement with a value
- Return statement without a value
- Return statement with multiple values
1. Return statement with a value
A return statement with a value is used to return a single value from a function. The value can be of any data type, such as a string, integer, or list.
def get_full_name(first_name, last_name):
full_name = f"{first_name} {last_name}"
return full_name
In the above example, we defined a function get_full_name
that takes two arguments first_name
and last_name
. The function then concatenates the two arguments to form the full name and returns it using the return
keyword.
2. Return statement without a value
A return statement without a value is used to exit a function and return control to the caller. It does not return any value.
def print_message(message):
if message:
print(message)
else:
return
In the above example, we defined a function print_message
that takes a single argument message
. The function then checks if the message is not empty and prints it. If the message is empty, the function exits using the return
keyword without returning any value.
3. Return statement with multiple values
A return statement with multiple values is used to return more than one value from a function. The values are separated by commas and enclosed in parentheses.
def get_name_and_age():
name = "John"
age = 25
return name, age
In the above example, we defined a function get_name_and_age
that does not take any argument. The function then sets two variables name
and age
and returns them as a tuple using the return
keyword.
How to use return statement in Flask
Flask provides a return
statement that is used to return a response to the client. The return
statement can be used to return a string, JSON data, or HTML content.
Returning a string
To return a string as a response, we can use the return
statement followed by the string.
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
In the above example, we defined a route /
that returns the string "Hello, World!" as a response using the return
statement.
Returning JSON data
To return JSON data as a response, we can use the jsonify
function provided by Flask.
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/person')
def get_person():
person = {"name": "John", "age": 25, "city": "New York"}
return jsonify(person)
In the above example, we defined a route /person
that returns a dictionary person
as JSON data using the jsonify
the function provided by Flask.
Returning HTML content
To return HTML content as a response, we can use templates provided by Flask. Templates are files that contain HTML code with placeholders for dynamic content.
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/profile/<name>')
def get_profile(name):
return render_template('profile.html', name=name)
In the above example, we defined a route /profile/<name>
that returns an HTML template profile.html
with the dynamic content name
using the render_template
the function provided by Flask.
Conclusion
In conclusion, the return statement is an essential concept in Python programming that allows us to return values from a function. It can be used to return a single value or multiple values, or exit a function without returning any value.
In web app development, Flask provides a return statement that is used to return a response to the client. We can use the return statement to return a string, JSON data, or HTML content. Understanding the concept of return statements is crucial in building more complex programs and making web apps.