File Input and Output (Creating Simple Web APP)
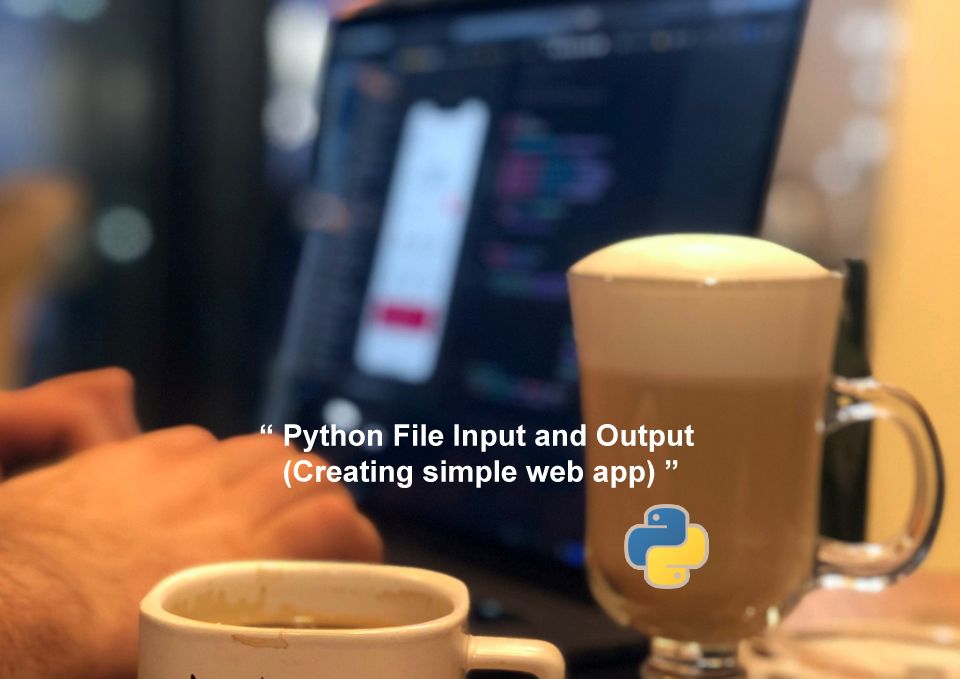
File Input and Output (Creating Simple Web APP)
The fundamentals of Python file input and output will be covered in this Article, including opening and closing files, reading and writing text files, utilizing the with statement, file modes, and managing exceptions.
Create a simple upload an image file
For this example, let's say we want to build a web application that allows users to upload an image file, and the application will then display the image back to the user.
To start, we will need to create a new Python file to contain our application code. We can call this file app.py
.
First
we will need to import the necessary modules for our application:
from flask import Flask, render_template, request, redirect, url_for
Flask
is a web framework for Python that allows us to build web applications.render_template
is a function that allows us to render HTML templates.request
is a module that allows us to access data submitted in a form.redirect
andurl_for
are modules that allow us to redirect the user to a different page.
Create a new instance of the Flask class
app = Flask(__name__)
This creates a new instance of the Flask class and assigns it to the variable app
.
Create a new route
let's create a new route for our application that will display a form for the user to upload their image:
@app.route('/')
def index():
return render_template('index.html')
This creates a new route for the root URL of our application (/
). When the user visits this URL, the index
function will be called, which will render the index.html
template.
Create template.
we need to create the index.html
template. We can create a new directory called templates
to store our HTML templates, and create a new file called index.html
inside this directory:
<!DOCTYPE html>
<html>
<head>
<title>Image Upload</title>
</head>
<body>
<h1>Upload an Image</h1>
<form method="POST" action="{{ url_for('upload') }}" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="Upload">
</form>
</body>
</html>
This HTML template contains a form that allows the user to upload an image file. When the user submits the form, the data will be sent to the upload
function, which we will create next.
Create route Upload
Let's create a new route for the upload
function:
@app.route('/upload', methods=['POST'])
def upload():
file = request.files['file']
file.save(file.filename)
return redirect(url_for('display', filename=file.filename))
This creates a new route for the /upload
URL, which will be called when the user submits the form in the index
template. The methods
parameter specifies that this route should only accept POST
requests.
Inside the upload
function, we use the request.files
object to access the uploaded file. We then save the file to the server's file system using the save
method, and redirect the user to the display
function.
Create route display
let's create a new route for the display
function:
@app.route('/display/<filename>')
def display(filename):
with open(filename, 'rb') as file:
data = file.read()
return data
This creates a new route for the /display
URL, which will be called when the user is redirected from the upload
function. The filename
parameter in the URL specifies the name of the uploaded file.
Inside the display
function, we open the file in binary mode using the with
statement and read its contents using the read
method. We then return the contents of the file to the user.
Update app.py
Finally, we need to add a few lines of code to the bottom of our app.py
file to run our application:
if __name__ == '__main__':
app.run(debug=True)
This checks if the current module is being run as the main program, and starts the Flask development server with debugging enabled.
Finish
Now that our application code is complete, we need to create a new directory called static
to store our uploaded image files. We can then run our application by running the following command in the terminal:
python app.py
This will start the Flask development server, and our application will be accessible at http://localhost:5000
.
o test our application, we can visit the root URL in our web browser (http://localhost:5000
) and upload an image file using the form. The application should then display the uploaded image back to the user.
Opening and Closing Files
In Python, opening a file with the open()
function is the first step in dealing with files. The file name and the opening option are this function's first and second arguments, respectively. The file can be opened in many ways, including read-only mode ('r')
, write-only mode ('w')
, and read-write mode ('r+')
.
For example
let's say we have a file called example.txt in our current directory, and we want to open it in read-only mode. We would use the following code:
file = open('example.txt', 'r')
Once we are done working with the file, we need to close it using the close()
method. This ensures that any changes we made to the file are saved and that the file is released from memory.
Reading and Writing Text Files:
After we learn to open a file, then we can also read the file or write to it. When reading a file, we use the read() method, which reads the entire contents of the file and returns it as a string.
For example
let's say our example.txt
file contains the following text:
Hello, world!
We could read this text into a variable using the following code:
file = open('example.txt', 'r')
text = file.read()
print(text)
This would output the following:
Hello, world!
When writing to a file, we use the write()
method, which writes a string to the file. If the file does not exist, it will be created. If it does exist, any existing contents will be overwritten.
For example, let's say we want to write the following text to a new file called newfile.txt
:
This is a new file.
We could write this text to the file using the following code:
file = open('newfile.txt', 'w')
file.write('This is a new file.')
file.close()
Using the with Statement
When working with files in Python, it is best to use the with statement, which automatically closes the file when we are finished with it. This helps to ensure that the file is properly closed, even if an exception occurs.
For example, we want to read from a file called example.txt
using the with the statement. We'd use the following code:
with open('example.txt', 'r') as file:
text = file.read()
print(text)
In this code, the with
statement ensures that the file is automatically closed when the block of code is finished executing, even if an exception occurs.
File Modes
As mentioned earlier, there are several modes in which a file can be opened in Python. The most common modes are:
- read-only mode (
'r'
) - write-only mode (
'w'
) - read-write mode (
'r+'
) - binary mode (
'b'
)
In addition, we can specify whether the file should be opened in text mode ('t'
) or binary mode ('b'
).
For example
if we want to open a file called image.jpg
in binary mode for reading, we would use the following code:
with open('image.jpg', 'rb') as file:
data = file.read()
print(data)
In this code, the 'rb'
mode specifies that the file should be opened in binary mode for reading.
Handling Exceptions
When working with files in Python, it is important to handle exceptions that may occur. Some common exceptions that may occur when working with files include:
FileNotFoundError
: This exception is raised when a file cannot be found.PermissionError
: This exception is raised when we do not have permission to access a file.IOError
: This exception is raised when an input/output error occurs.
We can use a try
/except
block to handle these exceptions and provide helpful error messages to the user.
For example
let's say we want to open a file called example.txt, but we are not sure if the file exists. We can use the following code to handle the FileNotFoundError exception:
try:
with open('example.txt', 'r') as file:
text = file.read()
print(text)
except FileNotFoundError:
print('The file could not be found.')
In this code, if the file cannot be found, the FileNotFoundError
exception will be raised, and the error message 'The file could not be found.'
will be printed.
Key Point :
- Python provides built-in functions and modules for working with files, such as the
open
function for opening files and theos
module for performing file system operations. - When opening files in Python, it's important to specify the file mode (e.g. "r" for reading, "w" for writing) and close the file when finished using it.
- Python's
with
statement is a useful way to ensure that files are automatically closed after they are opened. - When working with text files in Python, the
read
andwrite
methods can be used to read and write the contents of the file. - Exceptions can be used to handle errors that occur when working with files, such as when a file is not found or cannot be opened.
- File input and output can be used in web applications to allow users to upload and download files. The Flask web framework provides a convenient way to build web applications in Python.
- When building a file upload feature in a web application, it's important to ensure that uploaded files are validated and that appropriate security measures are taken to prevent malicious code from being uploaded.
- Overall, file input and output is an important topic in Python programming, and understanding how to work with files is a useful skill for a wide range of applications, including web development.