Python Functions: defining and calling functions
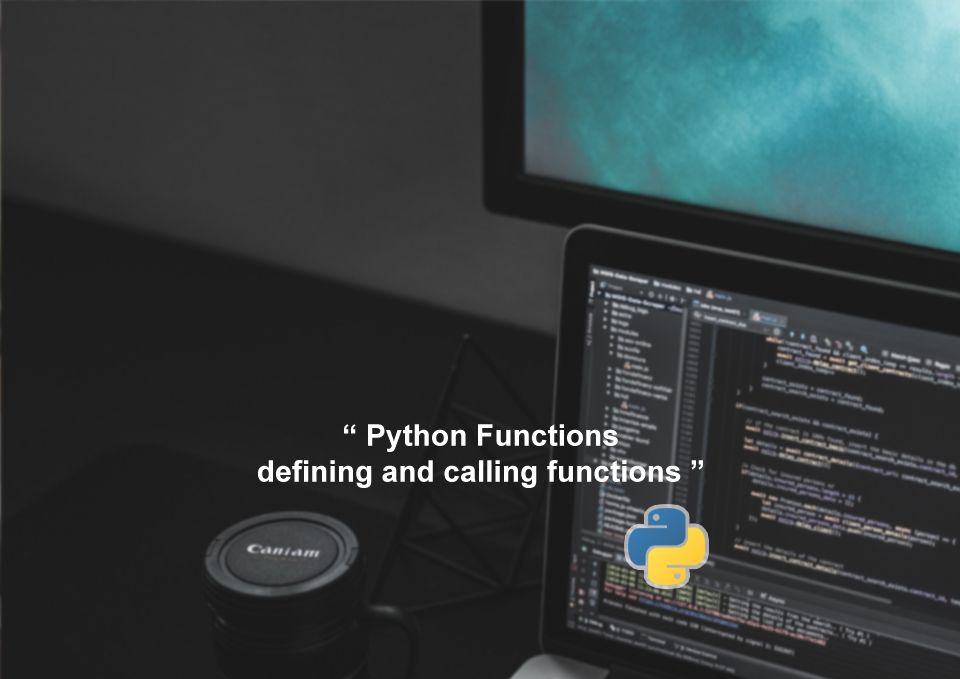
A crucial component of the Python programming language are functions. You can use them to create reusable code that can be used in other areas of your software. In Python, you use the def keyword, the function name, and a pair of parentheses to define a function. You can specify one or more parameters that the function will accept between the parentheses.
Here is an example of a simple function that accepts two parameters and returns their sum:
def add_numbers(x, y):
return x + y
To call this function, you simply need to provide the required arguments in the same order as the parameters. Here is an example of how to call the add_numbers
function:
result = add_numbers(3, 4)
print(result) # Output: 7
In this example, we pass the arguments 3 and 4 to the add_numbers
function, which returns their sum. We then assign the result to a variable called result
, and finally print the result to the console.
Analogies in Everyday Life
To help you better understand the concepts of defining and calling functions, let's look at some analogies from everyday life.
1. Defining a Recipe
Consider yourself a chef who wants to develop a new recipe for a delectable cake. To accomplish this, you must define a set of directions that another person can use to make the cake. These guidelines may include a list of the ingredients to be used, the order in which they should be combined, and the baking temperature and time.
A function in Python is comparable to a recipe. When you create a function, you are producing a set of guidelines that another person can follow to carry out a certain activity. Input parameters, variable assignments, and a return value could all be part of these instructions.
2. Calling a Mechanic
Imagine that you need to take your car to a mechanic to have it fixed because it is producing an odd noise. As soon as you get to the mechanic's shop, you describe the issue to the technician, and they examine your car. The mechanic identifies the problem, explains what has to be corrected, and provides a pricing estimate.
Calling a function in Python is like taking your automobile to the shop. A function is called when you want it to carry out a certain action and pass it any input parameters it requires. Following the function's code execution, it returns a result that you can utilize in your program.
Defining Functions
In Python, the def
keyword must be used before the function name and parentheses to declare a function. You can specify any necessary parameters for the function inside the parenthesis. When a function is called, these parameters are supplied to it as variables. The syntax for defining a straightforward function that writes a message to the console, for instance, is as follows:
def say_hello(name):
print("Hello, " + name + "!")
In this example, the function is called say_hello
, and it accepts a single parameter, name
. The function simply prints a message to the console, which includes the name parameter passed in.
Default Values Parameters
You can also specify default values for parameters in your function. This means that if the function is called without providing a value for the parameter, the default value will be used instead. Here's an example :
def greet(name="World"):
print("Hello, " + name + "!")
In this example, the function is called greet
, and it accepts a single parameter, name
, with a default value of "World". If no parameter is provided when the function is called, it will default to "World".
Return Keyword value
Finally, you can use the return
keyword to specify a value that the function should return. For example, here is a function that calculates the area of a rectangle and returns the result:
def calculate_area(width, height):
area = width * height
return area
In this example, the function is called calculate_area
, and it accepts two parameters, width
and height
. The function calculates the area of the rectangle by multiplying the width and height and assigns the result to the area
variable. The return
keyword specifies that the function should return the value of the area
variable.
Calling Functions
With Python, you merely use the function name enclosed in parentheses to invoke the function. You must provide any parameters the function requires inside the parenthesis. Here is an example of how to use the earlier say hello function:
say_hello("Alice")
You can also assign the result of a function to a variable. For example, here is how you would call the calculate_area
function from earlier and assign the result to a variable:
rectangle_area = calculate_area(10, 5)
In this example, the function is called with the parameters 10
and 5
, and the result (50)
is assigned to the rectangle_area
variable.
functions calling other functions
It is crucial to remember that functions can call other functions. This enables you to divide difficult jobs into smaller, easier to handle chunks. This is a straightforward program that utilizes two functions to determine the area of a circle as an illustration:
import math
def calculate_area(radius):
area = math.pi * radius ** 2
return area
def print_area(area):
print("This area,"+ calculate_area(5))
The calculate_area
function accepts the radius of the circle as a parameter and uses the math.pi
constant to calculate the area. The result is then returned to the calling function. The print_area
function accepts the area of the circle as a parameter and simply prints it to the console.
The main program calls the calculate_area
function with a radius of 5, and then passes the result to the print_area
function to print the result to the console.
Applying Code in Making a Web App
Now that we have a basic understanding of how to define and call functions in Python, let's look at some examples of how to apply this knowledge to making a web app.
User Authentication Function
User authentication is among the most popular features of an online application. We can develop a straight forward function that takes a username and password
as parameters and verifies their validity against a user database.
def authenticate_user(username, password):
# Check database for user info
if username in users.keys() and password == users[username]:
return True
else:
return False
In this example, the function is called authenticate_user
, and it accepts two parameters, username
and password
. The function checks the users
dictionary for the provided username and password. If the credentials are valid, the function returns True
. Otherwise, it returns False
.
We can then call this function whenever a user attempts to log in to the web app. If the function returns True
, we can grant the user access to the app. If it returns False
, we can display an error message to the user and prevent access to the app.
Best Practices for Writing Functions
When writing functions in Python, there are several best practices that you should follow to ensure that your code is clear, maintainable, and efficient. Here are a few of the most important best practices:
- Use clear and concise function names that accurately describe what the function does.
- Limit the function's responsibility to a single task, and avoid creating functions that are too long or too complex.
- Avoid using global variables, as they can make it difficult to reason about the behavior of your code.
- Handle errors using try-except statements to ensure that your code does not crash or produce unexpected behavior.
Conclusion
The fundamentals of defining and invoking functions in Python have been covered in this guide. To aid in your understanding of these ideas, we have offered examples and analogies from everyday life as well as some writing function best practices. You can write functions that are understandable, succinct, and useful, which improves the efficiency and maintainability of your code by doing so.
It is advised that you practice writing your own functions and investigate the extensive selection of libraries and modules offered for Python in order to further your grasp of the language's functions. You will acquire the abilities necessary to produce clear, effective, and efficient code as well as to fully exploit Python's function-oriented programming model with practice and experience.
Summary
In summary, Python functions are a fundamental aspect of the language and a key tool for organizing and reusing code. By learning how to define and call functions, and by following best practices for writing clear, maintainable, and efficient code, you can become a more effective and proficient Python programmer.
- Python Data Types and Variables
- Python Functions: defining and calling functions