Python Functions: built-in functions
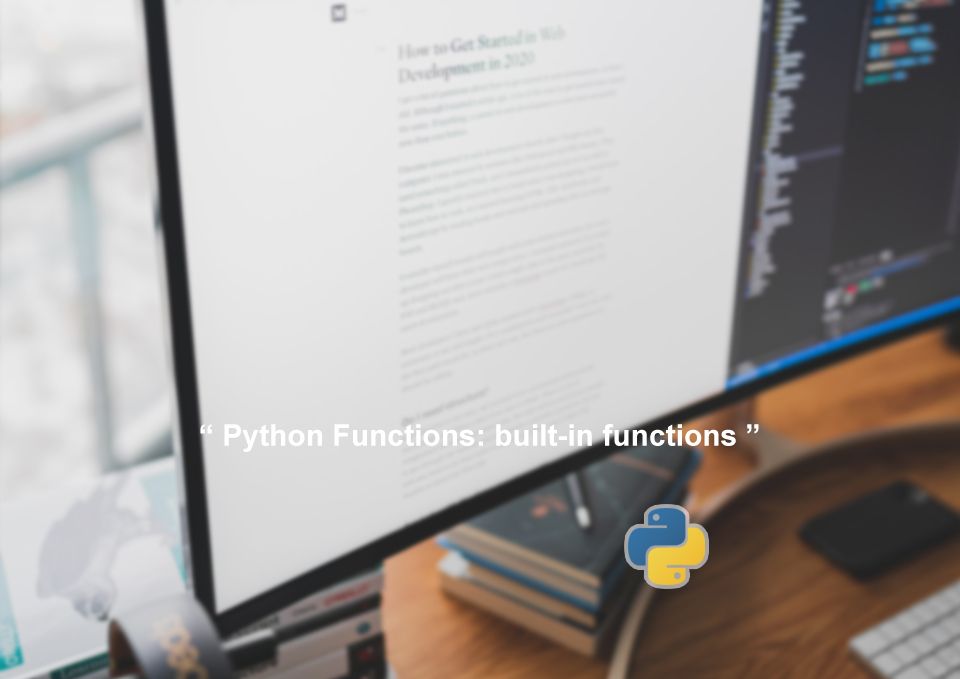
Python is a high-level programming language that is common in a variety of fields, including web development, machine learning, and data science. One of the main features of Python is its support for functions. You can reuse sections of code known as functions to complete various jobs.
In Python, there are a lot of built-in methods that can be used to complete a variety of jobs. In this article, we'll discuss some of Python's most well-liked built-in methods and demonstrate how to employ them to produce a simple web application.
Basic Built-in Functions
1. print()
The print() function is used to output values to the console. It takes one or more arguments and displays them on the screen.
For example, the following code will display the string "Hello, World!" on the console:
print("Hello, World!")
2. type()
The type() function is used to determine the type of a variable. It takes one argument and returns the type of the variable.
For example, the following code will return the type of the variable x:
x = 5
print(type(x))
Output:
<class 'int'>
3. len()
The len() function is used to determine the length of an object. It takes one argument and returns the length of the object.
For example, the following code will return the length of the string "Hello, World!":
str = "Hello, World!"
print(len(str))
Output:
13
4. range()
The range() function is used to generate a sequence of numbers. It takes one, two, or three arguments and returns a sequence of numbers.
For example, the following code will generate a sequence of numbers from 0 to 4:
for x in range(5):
print(x)
Output:
0
1
2
3
4
5. input()
The input() function is used to receive input from the user. It takes one argument, which is the prompt message to display to the user, and returns the user's input.
For example, the following code will prompt the user to enter their name and display it on the console:
name = input("Enter your name: ")
print("Hello, " + name + "!")
Mathematical Functions
1. abs()
The abs() function is used to return the absolute value of a number. It takes one argument and returns the absolute value of the number.
For example, the following code will return the absolute value of -4:
x = abs(-4)
print(x)
Output:
4
2. round()
The round() function is used to round a number to a specified number of digits. It takes one or two arguments and returns the rounded number.
For example, the following code will round the number 3.14159 to two decimal places:
x = round(3.14159, 2)
print(x)
Output:
3.14
3. max()
The max() function is used to find the maximum value in a sequence. It takes one or more arguments and returns the maximum value.
For example, the following code will find the maximum value in the list [3, 5, 2, 7, 1]:
x = max([3, 5, 2, 7, 1])
print(x)
Output:
7
4. min()
The min() function is used to find the minimum value in a sequence. It takes one or more arguments and returns the minimum value.
For example, the following code will find the minimum value in the list [3, 5, 2, 7, 1]:
x = min([3, 5, 2, 7, 1])
print(x)
Output:
1
String Functions
1. upper()
The upper() function is used to convert a string to uppercase. It takes no arguments and returns the uppercase string.
For example, the following code will convert the string "hello" to uppercase:
str = "hello"
print(str.upper())
Output:
HELLO
2. lower()
The lower() function is used to convert a string to a lowercase. It takes no arguments and returns the lowercase string.
For example, the following code will convert the string "HELLO" to lowercase:
str = "HELLO"
print(str.lower())
Output :
hello
3. strip()
The strip() function is used to remove whitespace from the beginning and end of a string. It takes no arguments and returns the stripped string.
For example, the following code will remove the whitespace from the string " hello ":
str = " hello "
print(str.strip())
Output:
hello
List Functions
1. append()
The append() function is used to add an item to the end of a list. It takes one argument, which is the item to be added to the list.
For example, the following code will add the number 4 to the end of the list [1, 2, 3]:
lst = [1, 2, 3]
lst.append(4)
print(lst)
Output:
[1, 2, 3, 4]
2. remove()
The remove() function is used to remove the first occurrence of an item from a list. It takes one argument, which is the item to be removed from the list.
For example, the following code will remove the number 2 from the list [1, 2, 3]:
lst = [1, 2, 3]
lst.remove(2)
print(lst)
Output :
[1, 3]
3. sort()
The sort() function is used to sort the items in a list in ascending order. It takes no arguments and returns the sorted list.
For example, the following code will sort the list [3, 1, 4, 2] in ascending order:
lst = [3, 1, 4, 2]
lst.sort()
print(lst)
Output:
[1, 2, 3, 4]
Building a Simple Web App
After going over some of Python's most popular built-in methods, let's look at how we can use them to create a straightforward web application.
We'll be utilizing the Flask web framework in this demonstration. Flask is a compact web framework that makes it simple and fast to create web applications.
First, we need to install Flask
Open a terminal and run the following command:
pip install Flask
Create a new file called app.py
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/calculate', methods=['POST'])
def calculate():
height = float(request.form['height'])
weight = float(request.form['weight'])
bmi = round(weight / (height ** 2), 2)
return render_template('result.html', bmi=bmi)
if name == 'main':
app.run(debug=True)
Let's break down what is happening in this code:
- We import the Flask module and two additional modules, render_template and request. render_template is used to render HTML templates, and request is used to handle incoming HTTP requests.
- We create a new instance of the Flask class and call it app.
- We define a new route for the root URL ('/'). When a user visits this URL, the index() function is called, which renders the index.html template.
- We define a new route for the '/calculate' URL. This route is only accessible via HTTP POST requests, which means that it can only be accessed when a user submits a form. The calculate() function is called when this route is accessed. The function gets the height and weight values from the form data using the request.form dictionary. We then calculate the user's BMI and round it to two decimal places using the round() function. Finally, we render the result.html template and pass the calculated BMI value as a variable.
- We start the Flask application using the app.run() method. We pass the debug=True argument to enable debugging mode, which allows us to see detailed error messages if there are any issues with our code.
let's create the HTML templates.
Create two new files in the same directory as app.py called index.html and result.html. Add the following code to index.html:
<!DOCTYPE html>
<html>
<head>
<title>BMI Calculator</title>
</head>
<body>
<h1>BMI Calculator</h1>
<form method="POST" action="/calculate">
<label for="height">Height (in meters):</label>
<input type="number" name="height" id="height" step="0.01" required>
<br>
<label for="weight">Weight (in kilograms):</label>
<input type="number" name="weight" id="weight" step="0.01" required>
<br>
<button type="submit">Calculate BMI</button>
</form>
</body>
</html>
This HTML template defines a simple form that allows the user to enter their height and weight values. The form data is submitted to the '/calculate' URL using the HTTP POST method.
Next, add the following code to result.html:
<!DOCTYPE html>
<html>
<head>
<title>BMI Calculator Result</title>
</head>
<body>
<h1>BMI Calculator Result</h1>
<p>Your BMI is: {{ bmi }}</p>
</body>
</html>
This HTML template displays the calculated BMI value that was passed as a variable from the Python code.
Run the web app
To run the web app, open a terminal, navigate to the directory where app.py is located, and run the following command:
python app.py
This will start the Flask application, and you should see output similar to the following:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
* Restarting with stat
* Debugger is active!
Open a web browser
Navigate to http://localhost:5000/.
You should see the BMI Calculator form. Enter your height and weight values and click the Calculate BMI button. You should be redirected to the BMI Calculator Result page, which displays your calculated BMI value.
key points for the article:
- Built-in functions are pre-defined functions in Python that can perform common operations on data.
- Examples of commonly used built-in functions include print(), len(), type(), and range().
- The print() function is used to display output to the console, while the len() function is used to calculate the length of a string, list, or tuple.
- The type() function is used to determine the data type of a variable, and the range() function is used to generate a sequence of numbers.
- Built-in functions can be combined with other Python features, such as loops and conditionals, to create more complex programs.
- We can use built-in functions to create simple web applications using the Flask web framework and HTML templates.
- Experimenting with built-in functions and exploring the Python documentation is an essential part of learning Python.
- Understanding built-in functions is a crucial step in becoming a proficient Python programmer.
- With time and practice, you can become comfortable using built-in functions and create powerful Python programs.