Python Function: parameters and arguments
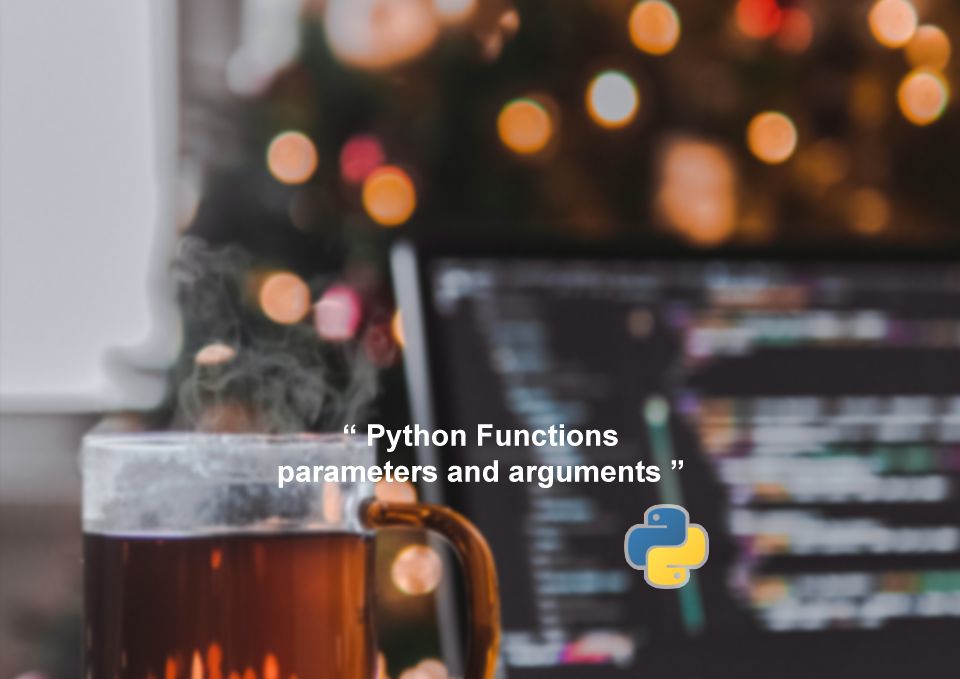
Python functions are a crucial piece of programming equipment. They make it possible for programmers to create reusable code that can be called repeatedly from various areas of the application.
Developers must grasp parameters and arguments in order to write efficient and effective Python functions since they enable data passing to the function.
We will examine the idea of parameters and arguments in Python functions in this article, offering a beginner-friendly tutorial and practical examples to help you comprehend how they operate.
Parameters
Parameters in Python functions are placeholders for values that are passed to the function. They define what types of data the function expects to receive and how many.
When a function is called, the values that are passed to the function are called arguments
5 types of parameters in Python functions:
1. Positional Parameters
These are the most common type of parameters, where the function expects the arguments to be passed in a specific order.The arguments are assigned to the parameters in the order they are passed to the function.
For example, let's consider a function to calculate the sum of two numbers:
def sum_numbers(num1, num2):
return num1 + num2
In this function, num1
and num2
are positional parameters. If we call this function with sum_numbers(5, 6)
, num1
will be assigned the value 5
, and num2
will be assigned the value 6
.
2. Keyword Parameters
These parameters allow the caller to specify which parameter each argument should be assigned to.
They are defined by specifying the parameter name, followed by the equals sign (=)
, and then the value
.
For example:
def divide_numbers(num1, num2):
return num1 / num2
We can call this function with divide_numbers(num2=5, num1=10)
, and the values of num1
and num2
will be assigned correctly.
3. Default Parameters
These are parameters that have default values assigned to them. If a value is not passed to the function for that parameter, the default value will be used.
For example:
def greet(name="John"):
return f"Hello, {name}"
We can call this function with greet()
and it will return "Hello, John"
. If we call greet("Alice")
, it will return "Hello, Alice"
.
4. Variable-Length Parameters
These are parameters that allow an indefinite number of arguments to be passed to the function.
They are defined by adding an asterisk (*)
before the parameter name.
For example:
def sum_numbers(*args):
total = 0
for num in args:
total += num
return total
We can call this function with sum_numbers(1, 2, 3, 4)
and it will return the sum
of all the arguments.
5. Keyword Variable-Length Parameters
These are parameters that allow an indefinite number of keyword arguments to be passed to the function.
They are defined by adding two asterisks (**)
before the parameter name.
For example:
def print_person(**kwargs):
print(f"Name: {kwargs.get('name')}")
print(f"Age: {kwargs.get('age')}")
print(f"City: {kwargs.get('city')}")
We can call this function with print_person(name="John", age=30, city="London")
and it will print
the details of the person.
Parameters in a web app
Let's consider an example of a web app that takes a user's name and age as input and returns a personalized greeting.
Here's the function that we can use:
def greet_user(name, age):
if age < 18:
return f"Hi, {name}! You must be accompanied by an adult to access this site."
else:
return f"Hello, {name}! Welcome to our site."
We can call this function with greet_user("Alice", 16)
and it will return
"Hi, Alice! You must be accompanied by an adult to access this site." If we call greet_user("Bob", 25)
, it will return
"Hello, Bob! Welcome to our site."
Arguments
Arguments are the values that are passed to the function when it is called.
3 types of arguments in Python:
1. Positional Arguments
These are the most common type of arguments, where the function expects the arguments to be passed in a specific order.
The order of the arguments must match the order of the parameters in the function definition.
2. Keyword Arguments
These arguments allow the caller to specify which parameter each argument should be assigned to.
They are defined by specifying the parameter name, followed by the equals sign (=)
, and then the value.
3. Unpacking Arguments
These arguments allow the caller to pass a list or tuple of values as separate arguments to the function.
They are defined by adding an asterisk (*)
before the list or tuple.
For example:
def multiply_numbers(num1, num2, num3):
return num1 * num2 * num3
numbers = [2, 4, 6]
result = multiply_numbers(*numbers)
In this example, we define a list of three numbers and pass it to the function using the asterisk (*)
operator. The function will receive the values 2, 4, and 6
as separate arguments.
Arguments in a web app
# Using positional arguments
greet_user("Alice", 16)
# Using keyword arguments
greet_user(name="Bob", age=25)
Let's consider the same example of a web app that takes a user's name and age as input and returns a personalized greeting.
We can call the greet_user()
function using both positional and keyword arguments.
Positional vs Keyword Arguments
Positional and keyword arguments have their advantages and disadvantages, depending on the use case.
Positional arguments
are easy to use and read, but the order of the arguments must be remembered.
Keyword arguments
can be used to specify which argument goes where, making the code more readable, but they require more typing.
Best practices for using positional and keyword arguments :
- Use positional arguments when the order of the arguments is important.
- Use keyword arguments when the order of the arguments is not important, and when the function has many parameters.
- Use default values for parameters whenever possible.
Positional and keyword arguments in a web app
Let's consider an example of a web app that takes a user's name, age, and city as input and returns a personalized greeting. Here's the function that we can use:
def greet_user(name, age, city="London"):
if age < 18:
return f"Hi, {name}! You must be accompanied by an adult to access this site in {city}."
else:
return f"Hello, {name}! Welcome to our site in {city}."
# Using positional arguments
greet_user("Alice", 16, "New York")
# Using keyword arguments
greet_user(name="Bob", age=25, city="Paris")
# Using default parameter value
greet_user("Charlie", 17)
All of these calls will return the correct personalized greeting, using either positional, keyword, or default parameter values.
Combining Parameters and Arguments
It is possible to combine parameters and arguments in various ways to make functions more flexible and easier to use.
One common technique is to use a variable number of arguments, which allows the function to accept any number of arguments.
Variable Number of Arguments
Python allows a function to accept a variable number of arguments using the *args
parameter. The *args
parameter is used to specify that the function can accept any number of positional arguments.
Here's an example:
def add_numbers(*args):
result = 0
for number in args:
result += number
return result
result = add_numbers(1, 2, 3, 4)
In this example, we define a function called add_numbers()
that takes a variable number of arguments using the *args
parameter.
The function then uses a loop to add all of the arguments together and return the result. We can call this function with any number of arguments, and it will return the sum of all the numbers.
Keyword Arguments with **kwargs
We can also use the **kwargs
parameter to accept any number of keyword arguments. The **kwargs
parameter is used to specify that the function can accept any number of keyword arguments.
Here's an example:
def greet_user(name, age, **kwargs):
city = kwargs.get("city", "London")
country = kwargs.get("country", "UK")
if age < 18:
return f"Hi, {name}! You must be accompanied by an adult to access this site in {city}, {country}."
else:
return f"Hello, {name}! Welcome to our site in {city}, {country}."
result = greet_user("Alice", 16, city="New York", country="USA")
In this example, we define a function called greet_user()
that takes a name, age, and any number of keyword arguments using the **kwargs
parameter.
The function then uses the get()
method to retrieve the values of the city and country parameters, with default values of "London" and "UK"
, respectively.
We can call this function with any number of keyword arguments, and it will return a personalized greeting.
Combining Arguments and Parameters in a Web App
def greet_user(name, age, *interests):
if age < 18:
return f"Hi, {name}! You must be accompanied by an adult to access this site."
message = f"Hello, {name}! "
if interests:
message += "I see you're interested in "
message += ", ".join(interests)
message += ". "
message += "Welcome to our site!"
return message
result = greet_user("Alice", 16, "reading", "swimming")
In this example, we define a function called greet_user()
that takes a name, age, and any number of interests using the *interests
parameter.
The function then uses a conditional statement to check if the user is under 18 years old. If so, it returns a message asking the user to be accompanied by an adult.
If not, it constructs a personalized greeting that includes the user's name and interests, if any. We can call this function with any number of interests, and it will return a personalized greeting that includes the user's interests, if any.
Key Points
- A function is a block of code that performs a specific task.
- Functions are defined using the
def
keyword, followed by the function name and the parameters in parentheses. - Parameters are variables that are used to pass data to a function, and arguments are the values that are passed to the function when it is called.
- Functions can be made more flexible by using variable arguments and keyword arguments.
- In Python, functions are first-class objects, which means they can be assigned to variables, passed as arguments to other functions, and returned as values from functions.
- When teaching Python functions to beginners, it's important to use analogies and practical examples to help them understand the concepts.
- Practical examples of using functions and parameters include performing calculations, manipulating data, interacting with external systems, and building web apps.
- By breaking down the concepts into manageable parts and providing clear, concise explanations, beginners can become confident in their ability to write and use functions in their own projects.