Python Data Types and Variables
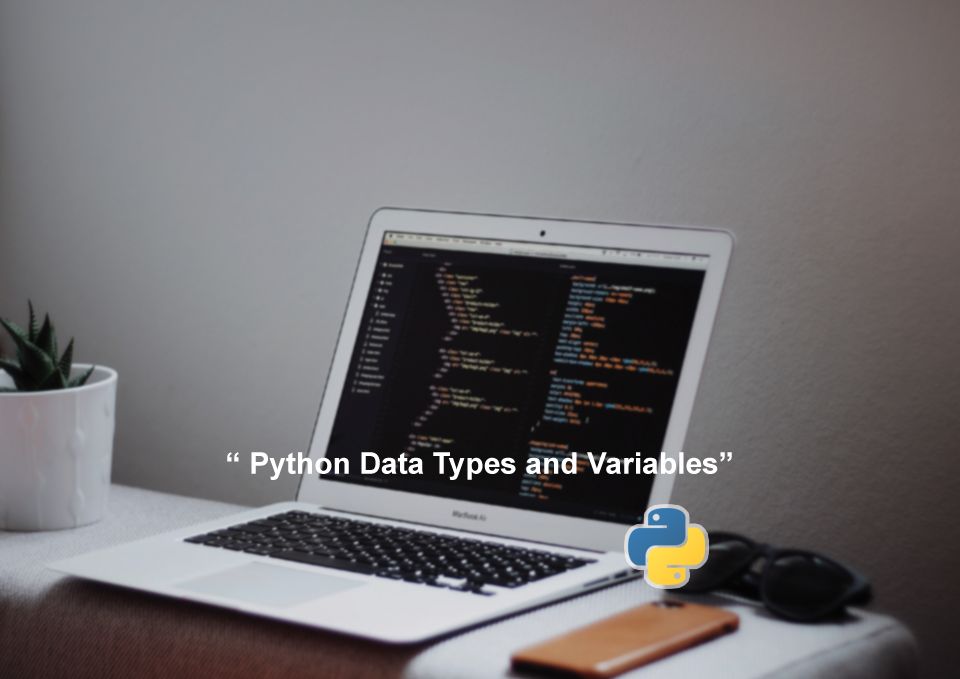
Variables and data types are important programming ideas, and Python offers a wide variety of data types to deal with. In Python, there are four fundamental data types: boolean values, strings, and floating-point numbers (int, float) (bool). Each of these data kinds has distinct characteristics and serves a variety of functions.
In Python, variables are used to hold values and are declared using a particular syntax. They can be used to carry out calculations, work with strings, and carry out other operations. They can store any of the data types mentioned above. Building complicated programs requires a thorough understanding of Python data types and variables, which serve as the foundation for all applications.
What are the 4 main data types in Python?
A. Integer (int) data type
A whole number that can be positive, negative, or zero is referred to as an integer in Python. Quantities like the amount of things in a shopping cart or the length of a text are represented by integers. Many mathematical operations, such as addition, subtraction, multiplication, and division, can be performed using integers.
Integers can be used to indicate the quantity of products and their prices, for instance, if you want to figure out the total cost of the things in a shopping cart. The final step is to compute the cost overall using mathematical techniques.
B. Floating-point (float) data type
Floating-point numbers, or floats, are used to represent decimal values. They can be positive, negative, or zero and are used in calculations that require precision, such as financial calculations or scientific calculations.
For instance, you can use floats to represent the temperature values while calculating the average temperature for a given month. The average temperature can then be determined by using mathematical processes.
C. String (str) data type
Text is represented by strings, which are collections of characters. Messages, addresses, and other text values are stored in strings. Concatenation, slicing, and formatting are just a few of the many operations that can be used to work with strings.
Using a string to store the user's name and concatenating it with a greeting message, for instance, can be used to create a greeting message for a user.
D. Boolean (bool) data type
A boolean value is a binary value that can be either true or false. Booleans are used to represent logical values, such as whether a condition is true or false. They are often used in control flow statements, such as if statements and while loops.
For example, if you want to check whether a user is logged in, you can use a boolean value to represent the user's authentication status.
Variables in Python
In Python, variables are used to hold values and are declared using a particular syntax. They can be used to carry out calculations, work with strings, and carry out other operations. They can store any of the data types mentioned above.
For instance, variables can be used to store the length and width of a rectangle so that the area of the rectangle can be calculated. The area can then be determined using mathematical operations.
Rules for declaring variables in Python
In Python, variables are declared using a specific syntax. The syntax for declaring a variable in Python is as follows:
variable_name = value
The variable name is the name that you give to the variable, and the value is the value that you want to store in the variable.
For example, if you want to store the value 10 in a variable called "x"
, you can use the following code:
x = 10
There are some rules that you need to follow when declaring variables in Python. These rules include the following:
- Variable names can contain letters, numbers, and underscores, but they cannot start with a number.
- Variable names are case-sensitive, so
"x"
and"X"
are two different variables. - Variable names should be descriptive and meaningful, to make the code easier to read and understand.
- Variable names should not be the same as Python keywords, such as
"if"
,"else"
, or"while"
.
Changing variable values
The ability to alter a variable's value at any time is one of Python's key features. This implies that you can carry out calculations, store user input, and carry out other operations using variables.
For instance, variables can be used to store the length and width of a rectangle so that the area of the rectangle can be calculated. The area of various rectangles can then be calculated by altering the values of the variables.
Type conversion
Using type conversion, you can change the data type of an object in Python. The process of changing one data type into another is known as type conversion. There are several built-in type conversion functions in Python, including int()
, float()
, str()
, and bool ()
.
For instance, you can use the int()
function to turn a string into an integer. Similarly, you can use the str()
function to change an integer into a string.
Integer (int) data type
Depending on the system architecture, Python represents integers using either 32 or 64 bits. This means that the range of integers that Python can represent is constrained by the number of bits that are required to do so.
For instance, on a 32-bit system, the range of integers that can be represented is from -2,147,483,648 to 2,147,483,647.
Examples of int data type
Here are some examples of how the int data type can be used in Python:
- Counting: Integers are commonly used to represent counts in Python. For example, you might use an integer variable to count the number of times a user clicks a button on a web page.
- Indexing: Integers are also commonly used to index into arrays and lists in Python. For example, you might use an integer variable to access a specific element in an array.
- Arithmetic: Integers are used in a wide variety of arithmetic operations in Python, such as addition, subtraction, multiplication, and division. For example, you might use integers to calculate the total cost of a purchase, or to calculate the average score of a group of students.
Here is an example of how the int data type can be used in a Python program to calculate the sum of two integers:
# Declare two integer variables
a = 5
b = 7
# Add the two integers and store the result in a third variable
c = a + b
# Print the result
print("The sum of", a, "and", b, "is", c)
In this example, we declare two integer variables (a and b), add them together using the + operator, and store the result in a third variable (c). We then use the print() function to display the result on the console.
Integers are a simple yet powerful data type in Python, and they are used extensively in both basic and advanced applications. Whether you are counting, indexing, or performing arithmetic operations, the int data type is an essential tool in your Python programming toolkit.
Floating-point (float) data type
For representing decimal numbers with a fractional component in Python, use the floating-point data type (float). In Python, floats are a fundamental data type that are used for a variety of purposes, including scientific computations, financial calculations, and geometric calculations.
The IEEE 754 standard, which depends on the system architecture, uses either 32 or 64 bits to represent a number when using floats in Python. Therefore, Python's ability to represent a wide range of floating-point numbers is constrained by the number of bits that can be assigned to each one. The range of floating-point numbers that can be represented, for instance, on a 32-bit system, is roughly between 1.2 x 10-38 and 3.4 x 1038.
Examples of float data type
- Scientific computations: Floats are commonly used to represent measurements in scientific computations. For example, you might use a float variable to represent the temperature in Celsius or the weight of an object in grams.
- Financial calculations: Floats are also commonly used in financial calculations, such as calculating interest or performing currency conversions. For example, you might use a float variable to calculate the total interest paid on a loan.
- Geometry: Floats are used in a wide variety of geometric calculations, such as calculating the area of a circle or the volume of a sphere. For example, you might use a float variable to calculate the area of a circle with a given radius.
Here is an example of how the float data type can be used in a Python program to calculate the total price of an item with sales tax:
# Declare a float variable for the price of the item
price = 9.99
# Declare a float variable for the sales tax rate
tax_rate = 0.07
# Calculate the total price with sales tax
total_price = price * (1 + tax_rate)
# Print the result
print("The total price with sales tax is", total_price)
Here, we declare two float variables (price and tax rate), use the * operator to calculate the total price with sales tax, and then store the result in a third variable (total price). The print() function is then employed to show the outcome on the console.
In Python, floats are a potent data type that are widely used in both simple and complex applications. The float data type is a crucial component of your Python programming toolbox whether you are working with financial calculations, scientific calculations, or geometric calculations.
String (str) data type
A string (str) is a group of characters in the Python programming language that can include letters, numbers, and symbols. In Python programs, text data is represented by strings, which can be altered in a variety of ways. A group of characters can be declared as a string by enclosing them in either single (' ') or double quotes (" ").
A string cannot be changed once it has been created because the string data type is immutable. However, you can alter an existing string to produce a new one, such as by joining two strings together or cutting it in half to obtain a substring.
Examples of str data type
Here are some examples of how the string data type can be used in Python:
- Text data: Strings are commonly used to represent text data in Python programs, such as the contents of a file, the text of a message, or the name of a person. For example, you might use a string variable to represent a user's name, as in the following code:
# Declare a string variable for the user's name
name = "John Smith"
# Print a message to greet the user
print("Hello, " + name + "!")
In this example, we declare a string variable (name) and use the concatenation operator (+) to combine it with a greeting message.
- Data validation: Strings can be used to validate input from a user or an external source, such as a file or a database. For example, you might use a regular expression to validate an email address, as in the following code:
import re
# Declare a string variable for the email address
email = "john@example.com"
# Validate the email address using a regular expression
if re.match(r"[^@]+@[^@]+\.[^@]+", email):
print("The email address is valid.")
else:
print("The email address is not valid.")
In this example, we use the re module to match the string variable (email) against a regular expression pattern that represents a valid email address.
- String manipulation: Strings can be manipulated in a wide variety of ways, such as by slicing, concatenating, or replacing substrings. For example, you might use the replace() method to replace a substring in a string, as in the following code:
# Declare a string variable for a message
message = "Hello, world!"
# Replace the word "world" with "Python"
new_message = message.replace("world", "Python")
# Print the new message
print(new_message)
In this example, we declare a string variable (message) and use the replace() method to create a new string (new_message) that replaces the word "world" with "Python".
Boolean (bool) data type
In Python, the Boolean data type is used to represent true/false values. The bool data type has only two possible values: True and False. Boolean values are important in programming because they help control the flow of a program.
The bool data type is a subclass of the integer data type. In Python, True is equal to 1 and False is equal to 0. This means that you can perform arithmetic operations on Boolean values, just like you can with integer values.
Examples of bool data type
Here are some examples of using Boolean values in Python:
# Example 1
x = 5
y = 3
print(x > y) # Output: True
# Example 2
a = True
b = False
print(a and b) # Output: False
# Example 3
is_raining = True
if is_raining:
print("Remember to bring an umbrella!") # Output: "Remember to bring an umbrella!"
In Example 1, we compare the values of x and y using the greater-than operator (>
). Since x is greater than y, the output is True.
In Example 2, we use the Boolean operators and
to combine two Boolean values. The and
operator returns True only if both operands are True. In this case, since one of the operands is False, the output is False.
In Example 3, we use a Boolean value as the condition in an if
statement. The code inside the if
block is executed only if the condition is True.
Overall, the Boolean data type is a fundamental part of programming in Python, and it's important to understand how to use it effectively in your code.
Summary of key points
I. Introduction
- Python is a popular programming language used for various purposes
- Data types and variables are fundamental concepts in Python programming
A. Integer (int) data type
- The int data type represents whole numbers
- Integers can be positive, negative, or zero
- Integers are immutable in Python
B. Floating-point (float) data type
- The float data type represents decimal numbers
- Floating-point numbers can be positive, negative, or zero
- Floating-point numbers can be represented with scientific notation
- Floating-point numbers are susceptible to rounding errors
C. String (str) data type
- The str data type represents text
- Strings are enclosed in quotes (single or double)
- Strings can be concatenated, sliced, and formatted
- Escape sequences are used to represent special characters
D. Boolean (bool) data type
- The bool data type represents true or false values
- Booleans are used in conditional statements and loops
- Booleans can be combined using logical operators (and, or, not)
A. Variables in Python
- Variables are used to store values in Python
- Variables must be declared before they can be used
- Variables can be reassigned to different values
B. Rules for declaring variables in Python
- Variable names can only contain letters, numbers, and underscores
- Variable names cannot start with a number
- Variable names are case-sensitive
- Variable names should be descriptive
C. Examples of variable declaration in Python
- Variables can be assigned values using the equals sign (=)
- Variables can be assigned values of different data types
- Multiple variables can be assigned values simultaneously
Overall, understanding data types and variables is crucial for anyone learning Python as they form the building blocks of most programming tasks. By having a clear grasp of these concepts, beginners can start creating simple programs and applications in no time.
- Python Data Types and Variables