Python Data Structures (lists, tuples, dictionaries, sets, indexing, and slicing)
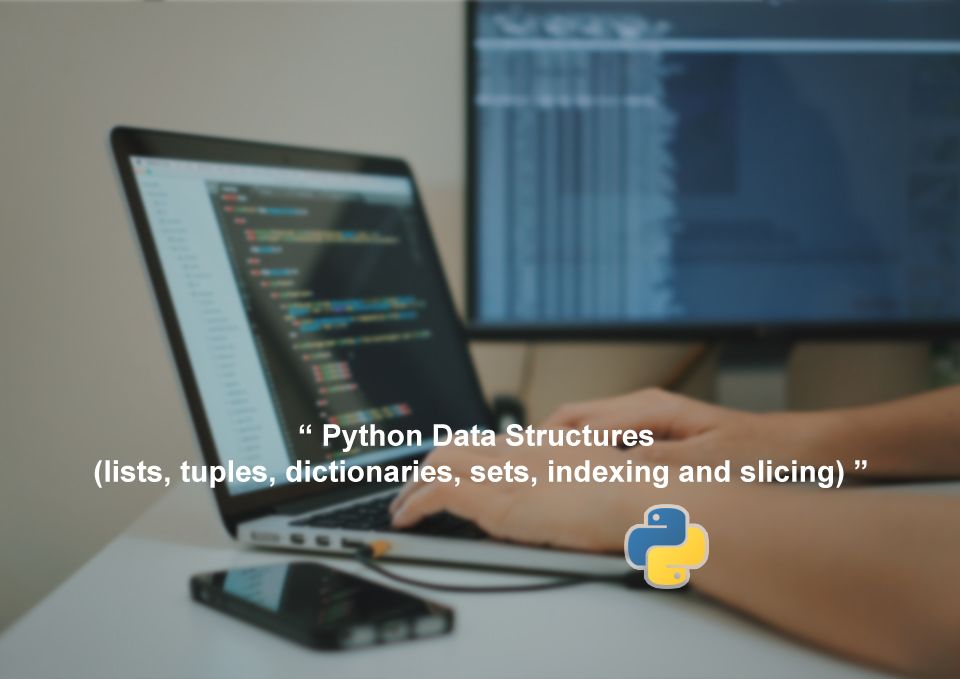
How to write effective and fast code in Python requires a basic understanding of data structures. To effectively access, manipulate, and arrange data, data structures are containers that store data in a specific way.
Lists, tuples, arrays, and sets are Python data structures most utilized. This essay will examine these structures and analyze how indexing and slicing can be used to manage them.
What is a list in data structure?
One of the most fundamental and adaptable data structures in Python is lists. They are used to store a variety of data objects, including strings, integers, and even other lists.
A list can be made by enclosing a collection of values in square brackets, which appear as follows:
my_list = [1, 2, 3, "four", 5.6, [7, 8, 9]]
Lists are mutable because they can have components added, removed, or changed after being made. for Specific elements of a list can be accessed by using a number, which is an integer that indicates the position of the element in the list.
The first element of a list has an index of zero because Python employs 0-based indexing. The last element in the list is denoted by the number -1, and negative indexing can be used to reach elements from the end of the list.
For example, to access the first element of the list above, you can use:
print(my_list[0]) # Output: 1
To modify an element in a list, you can simply assign a new value to the corresponding index:
my_list[1] = "two"
print(my_list) # Output: [1, 'two', 3, 'four', 5.6, [7, 8, 9]]
You can also add elements to the end of a list using the append()
method, or insert elements at a specific position using the insert()
method:
my_list.append("six")
print(my_list) # Output: [1, 'two', 3, 'four', 5.6, [7, 8, 9], 'six']
my_list.insert(2, "three")
print(my_list) # Output: [1, 'two', 'three', 3, 'four', 5.6, [7, 8, 9], 'six']
What is a tuple in Python with an example?
Lists and tuples share the ability to store a collection of data objects. However, since they are immutable, you cannot change, add, or remove components after they have been created.
A comma-separated list of numbers can be turned into a tuple by enclosing it in parentheses, as in the following example:
my_tuple = (1, 2, 3, "four", 5.6)
Like lists, you can access individual elements of a tuple by using an index. However, you cannot modify the elements of a tuple:
print(my_tuple[3]) # Output: four
my_tuple[3] = "FOUR" # Raises a TypeError!
Tuples are generally used to hold a fixed set of data items that should not be changed, such as the coordinates of a point in space or the RGB values of a color.
What is a structure of a dictionary?
Dictionaries are used to hold a collection of key-value pairs, where each key maps to a specific value. You can create a dictionary by enclosing a comma-separated sequence of key-value pairs in curly braces, like this:
my_dict = {"name": "Alice", "age": 30, "city": "New York"}
In this example, the keys are "name"
, "age"
, and "city"
, and the corresponding values are "Alice"
, 30, and "New York", respectively. You can access the value of a specific key in a dictionary by using the square bracket notation:
print(my_dict["name"]) # Output: Alice
If you try to access a key that does not exist in the dictionary, Python will raise a KeyError
. To avoid this, you can use the get()
method, which returns the value of the key if it exists in the dictionary, or a default value if it does not:
print(my_dict.get("gender", "unknown")) # Output: unknown
You can add new key-value pairs to a dictionary by using the square bracket notation or the update()
method:
my_dict["gender"] = "female"
print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York', 'gender': 'female'}
my_dict.update({"age": 31, "state": "California"})
print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'city': 'New York', 'gender': 'female', 'state': 'California'}
What is a set data structure?
Sets are used to hold a collection of unique values. You can create a set by enclosing a comma-separated sequence of values in curly braces, or by using the set()
constructor function:
my_set = {1, 2, 3, 4, 5}
another_set = set([3, 4, 5, 6, 7])
In this example, my_set
and another_set
contain the values 3, 4, and 5, but my_set
also contains 1 and 2, while another_set
also contains 6 and 7. Sets are mutable, which means that you can add and remove elements from them. You can add a new element to a set using the add()
method, or remove an element using the remove()
method:
my_set.add(6)
print(my_set) # Output: {1, 2, 3, 4, 5, 6}
my_set.remove(2)
print(my_set) # Output: {1, 3, 4, 5, 6}
Indexing and Slicing
Indexing and slicing are powerful tools for working with data structures in Python. Indexing allows you to access individual elements of a data structure, while slicing allows you to access a contiguous subset of elements. Both indexing and slicing use square bracket notation.
For example, to access the first three elements of a list, you can use:
my_list = [1, 2, 3, 4, 5]
print(my_list[0:3]) # Output: [1, 2, 3]
In this example, the slice notation 0:3
means "start at index 0 and stop at index 3 (exclusive)", so the resulting slice contains the elements with indices 0, 1, and 2.
Example web app
Here's an example web app that uses several data structures in Python, including lists, dictionaries, and sets:
from flask import Flask, render_template, request
app = Flask(__name__)
# Define a list of products
products = [
{"name": "T-Shirt", "price": 19.99, "sizes": ["S", "M", "L"], "colors": ["Red", "Blue", "Green"]},
{"name": "Hoodie", "price": 39.99, "sizes": ["S", "M", "L"], "colors": ["Black", "Gray", "Navy"]},
{"name": "Jeans", "price": 49.99, "sizes": ["S", "M", "L"], "colors": ["Blue", "Black", "Gray"]},
{"name": "Sneakers", "price": 69.99, "sizes": ["US 6", "US 7", "US 8"], "colors": ["White", "Black", "Red"]}
]
# Define a dictionary of user data
users = {
"user1": {"name": "John", "email": "john@example.com", "password": "password1"},
"user2": {"name": "Jane", "email": "jane@example.com", "password": "password2"}
}
@app.route('/')
def index():
return render_template('index.html', products=products)
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
if username in users and users[username]['password'] == password:
return render_template('dashboard.html', user=users[username])
else:
return render_template('login.html', message='Invalid username or password')
else:
return render_template('login.html')
@app.route('/logout')
def logout():
return render_template('logout.html')
if __name__ == '__main__':
app.run(debug=True)
This app defines a Flask web app with two data structures: a list of products and a dictionary of user data. The app also uses the render_template()
function to render HTML templates for the login page, dashboard page, and logout page.
The login page uses a POST
request to handle user login credentials. If the user enters valid credentials, the app renders the dashboard page and passes in the user's data. The dashboard page displays the user's name and a table of products, which can be filtered by size and color using HTML forms and Jinja2 syntax.
To run this app, you'll need to create several templates: index.html
, login.html
, dashboard.html
, and logout.html
. Here's an example template for the dashboard page:
<!DOCTYPE html>
<html>
<head>
<title>Dashboard</title>
</head>
<body>
<h1>Welcome, {{ user.name }}!</h1>
<h2>Products</h2>
<form method="get" action="">
<label for="size">Size:</label>
<select id="size" name="size">
<option value="">All</option>
{% for size in sizes %}
<option value="{{ size }}">{{ size }}</option>
{% endfor %}
</select>
<label for="color">Color:</label>
<select id="color" name="color">
<option value="">All</option>
{% for color in colors %}
<option value="{{ color }}">{{ color }}</option>
{% endfor %}
</select>
<button type="submit">Filter</button>
</form>
<table>
<thead>
<tr>
<th>Name</th>
<th>Price</th>
<th>Sizes</th>
<th>Colors</th>
</tr>
</thead>
<tbody>
{% for product in products %}
{% if size == '' or size in product.sizes %}
{% if color == '' or color in product.colors %}
<tr>
<td>{{ product.name }}</td>
<td>{{ product.price }}</td>
<td>{{ product.sizes }}</td>
<td>{{ product.colors }}</td>
</tr>
{% endif %}
{% endif %}
{% endfor %}
</tbody>
</table>
<a href="/logout">Logout</a>
</body>
</html>
This template uses Jinja2 syntax to iterate over the list of products and filter them by size and color using HTML forms. The sizes
and colors
variables are generated dynamically based on the available sizes and colors in the product list.
Overall, this web application demonstrates the power and flexibility of Python data structures in building dynamic and interactive web applications. By combining lists, dictionaries, sets, and other data structures with web frameworks like Flask, developers can create complex and highly customized web applications that meet a wide range of user needs.