Python Conditional Statements and Loops
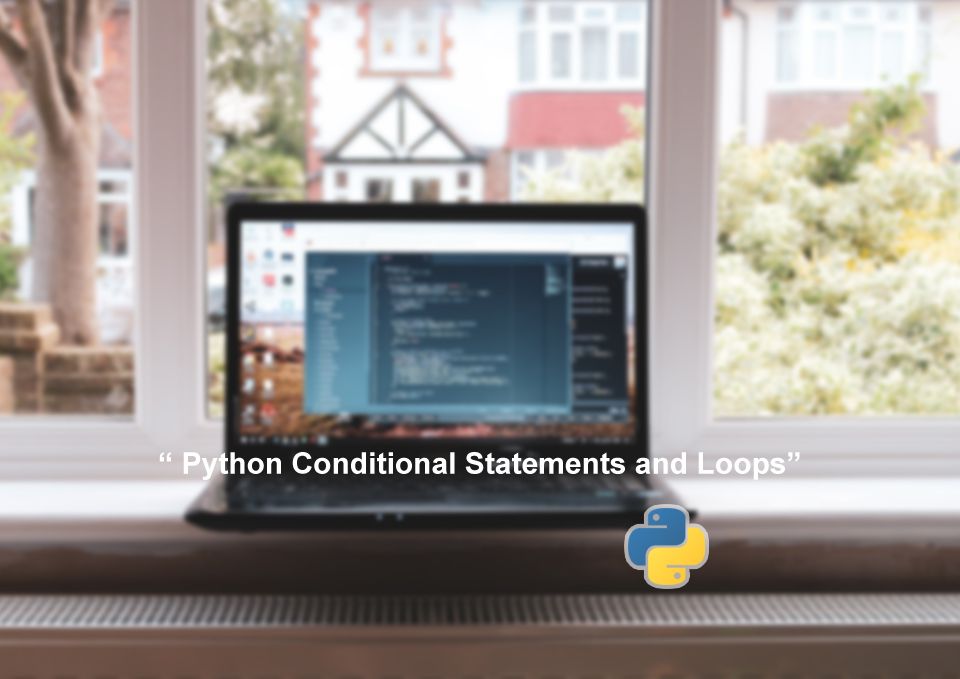
Conditional statements and loops are two important concepts in programming that help control the flow of code. Conditional statements allow you to execute different blocks of code based on certain conditions, while loops allow you to repeat a block of code until a certain condition is met. Together, they provide a powerful toolset for creating complex programs.
You can think of the importance of conditional statements and loops like a set of tools you might use to build a house. Each tool serves a different purpose, but together they allow you to construct a complex and functional structure. Conditional statements and loops are similar tools in web development. They allow you to build complex and dynamic websites and web applications that can respond to user input and provide a rich user experience.
This article will cover the basic syntax and functionality of if/else statements, while loops, for loops, the range function, and break/continue statements. It will also provide best practices and examples for using these tools effectively.
If/else statements
If/else statements are a type of conditional statement in programming that allow you to execute different blocks of code based on a certain condition. The "if" statement checks whether a certain condition is true, and if it is, executes a certain block of code. The "else" statement provides a fallback option in case the condition is not true, allowing you to execute a different block of code. Together, if/else statements provide a powerful toolset for controlling the flow of your program.
For example, you might say, "If you arrive before 6pm, come in through the front door. If you arrive after 6pm, come in through the back door." This is a lot like an if/else statement in programming. You're checking the time of day (the condition), and executing different instructions based on whether the condition is true or false.
Basic syntax of if/else statements
The basic syntax of an if/else statement in most programming languages is:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
The condition is typically a Boolean expression that evaluates to either true or false. If the condition is true, the code inside the if block is executed. If the condition is false, the code inside the else block is executed.
if/else statements in web development
To display different content to various users based on their login status, for instance, you could use an if/else statement. Depending on the user's device, you could use an if/else statement to run different code (e.g. mobile vs desktop). As an illustration, consider the following JavaScript if/else statement, which displays various contents depending on the user's login status:
if (userLoggedIn) {
// Display content for logged-in users
} else {
// Display content for non-logged-in users
}
Best practices if/else statements
Then using if/else statements in your code, it's important to follow best practices to ensure your code is readable and maintainable. Some best practices include:
- Use clear and descriptive variable names for your conditions
- Use proper indentation and formatting to make your code easy to read
- Avoid nesting too many if/else statements, as this can make your code hard to follow
- Use comments to explain the purpose of your if/else statements and any complex logic involved
By following these best practices, you can ensure your if/else statements are easy to understand and maintain, even as your codebase grows and changes over time.
While loops
While loops are a type of loop in programming that execute a block of code as long as a certain condition is true. The condition is checked at the beginning of each iteration of the loop, and if it's true, the loop body is executed. This process continues until the condition is false, at which point the loop exits.
In everyday life, you can think of while loops like a game of "Simon Says." The person leading the game gives a set of instructions, and the players must follow those instructions as long as they're preceded by the phrase "Simon says." If the instruction isn't preceded by that phrase, the players should not follow it. This is similar to how while loops work in programming. The condition is like the "Simon says" phrase, and the loop body is like the set of instructions that must be followed as long as the condition is true.
Basic syntax of while loops
The basic syntax of a while loop in most programming languages is:
while (condition) {
// Code to execute while the condition is true
}
The condition is typically a Boolean expression that evaluates to either true or false. The code inside the loop body will continue to execute as long as the condition is true.
While loops in web development
The use of while loops in web development is beneficial for many different tasks.
Use a while loop, for instance, to iterate through an array of items and carry out a specific action on each one. A while loop in JavaScript is demonstrated here, iterating through an array and writing each item to the console:
let items = ['apple', 'banana', 'orange'];
let i = 0;
while (i < items.length) {
console.log(items[i]);
i++;
}
This loop will continue to execute as long as the value of i
is less than the length of the items
array. On each iteration, it will log the current item to the console and increment the value of i
.
Best practices while loops
When using while loops in your code, it's important to follow best practices to ensure your code is efficient and bug-free. Some best practices include:
- Ensure that the condition will eventually become false, or else the loop will execute indefinitely (this is known as an infinite loop)
- Use clear and descriptive variable names for your loop counter and condition
- Avoid nesting too many while loops, as this can make your code hard to follow
- Use comments to explain the purpose of your loop and any complex logic involved
By following these best practices, you can ensure your while loops are easy to understand and maintain, even as your codebase grows and changes over time.
For loops
For loops are another type of loop in programming that allow you to execute a block of code a specific number of times. Unlike while loops, which continue to execute as long as a condition is true, for loops are ideal for iterating over a fixed range of values. This makes them useful for tasks like processing arrays or lists, or performing calculations a specific number of times.
In everyday life, you can think of for loops like counting. When you count from 1 to 10, you're essentially using a for loop. You start at 1, increment by 1 on each iteration, and stop when you reach 10. Similarly, a for loop in programming allows you to define a starting value, an ending value, and a step size, and then perform an action for each value in that range.
Basic syntax of for loops
The basic syntax of a for loop in most programming languages is:
for (initialization; condition; increment/decrement) {
// Code to execute for each value in the loop
}
The initialization
step is where you define the starting value for the loop. The condition
step is the condition that must be true for the loop to continue executing, and the increment/decrement
step is the action to take at the end of each iteration (e.g. adding 1 to a counter).
for loops in web development
For loops are commonly used in web development for tasks like iterating over arrays or creating tables of data. Here's an example of a for loop in JavaScript that iterates over an array of numbers and calculates their sum:
let numbers = [1, 2, 3, 4, 5];
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
console.log(sum); // Outputs 15
In this example, the for loop initializes a counter variable i
to 0, and continues to execute as long as i
is less than the length of the numbers
array. On each iteration, the current number in the array is added to the sum
variable, and the value of i
is incremented by 1.
Best practices for loops
When using for loops in your code, it's important to follow best practices to ensure your code is efficient and maintainable. Some best practices include:
- Use clear and descriptive variable names for your loop counter and other variables
- Avoid modifying the loop counter inside the loop body, as this can lead to unexpected behavior
- Use the
break
statement to exit the loop early if a certain condition is met - Use the
continue
statement to skip over certain iterations of the loop if a condition is met - Use comments to explain the purpose of your loop and any complex logic involved
By following these best practices, you can ensure your for loops are easy to understand and maintain, even as your codebase grows and changes over time.
Range function
The range function in Python is used to generate a sequence of numbers. It is a built-in function that can be used to create a list, tuple, or set of numbers. The range function takes in three arguments - start, stop, and step - and generates a sequence of numbers between the start and stop values, with an increment of the step value.
Basic syntax range function
The basic syntax of the range function is as follows:
range(start, stop, step)
Here, the start parameter specifies the starting value of the sequence, the stop parameter specifies the ending value of the sequence (exclusive), and the step parameter specifies the increment between each number in the sequence.
Examples range function in web development:
The range function can be used in a variety of ways in web development. For example, it can be used to iterate through a sequence of numbers and perform a certain action for each number. Here's an example of how the range function can be used in a simple web application to generate a list of even numbers:
<!DOCTYPE html>
<html>
<head>
<title>Even Numbers</title>
</head>
<body>
<h1>Even Numbers</h1>
<ul>
{% for num in range(2, 11, 2) %}
<li>{{ num }}</li>
{% endfor %}
</ul>
</body>
</html>
In this example, the range function is used to generate a sequence of even numbers between 2 and 10 (inclusive), and the for loop is used to iterate through each number in the sequence and display it as a list item.
Best practices range function
When using the range function, it's important to keep in mind a few best practices to ensure that your code is efficient and readable. Here are some tips:
- Use the range function when you need to generate a sequence of numbers. It is more efficient than creating a list of numbers using a for loop.
- Be mindful of the start, stop, and step parameters. Make sure that you specify the correct values to generate the desired sequence of numbers.
- Use the range function in combination with other Python statements such as for loops or list comprehensions to perform more complex operations.
- Avoid using the range function for generating large sequences of numbers. In such cases, it is better to use a generator expression, which will consume less memory.
Break and Continue Statements
In programming, it's common to encounter situations where we need to exit a loop prematurely or skip to the next iteration. This is where the break and continue statements come in handy.
The break statement is used to exit a loop prematurely when a certain condition is met. For example, if you're searching for an item in a list, once you find the item, you can exit the loop using the break statement.
The continue statement, on the other hand, is used to skip the current iteration of a loop and move on to the next iteration. This is useful when you want to skip certain values in a list that don't meet a specific condition.
Basic Syntax of Break and Continue Statements
The syntax for the break statement is simple. It's used within a loop and is usually placed within an if statement that checks for a condition to exit the loop. Here's an example:
for i in range(10):
if i == 5:
break
print(i)
In the above example, the loop starts at 0 and ends at 9. When the value of i is 5, the if statement is true and the break statement is executed, causing the loop to exit prematurely.
The syntax for the continue statement is also simple. It's used within a loop and is usually placed within an if statement that checks for a condition to skip the current iteration. Here's an example:
for i in range(10):
if i == 5:
continue
print(i)
In the above example, when the value of i is 5, the if statement is true and the continue statement is executed, causing the loop to skip the current iteration.
Examples of using Break and Continue Statements in Web Development
In web development, you may use break and continue statements to iterate through arrays or perform certain actions based on certain conditions. Here's an example of using the break statement to exit a loop when a certain condition is met:
fruits = ["apple", "banana", "cherry", "orange", "kiwi", "melon", "mango"]
for fruit in fruits:
if fruit == "orange":
break
print(fruit)
In the above example, the loop iterates through the fruits array. When it gets to the value "orange", the if statement is true and the break statement is executed, causing the loop to exit prematurely. The output will be:
apple
banana
cherry
Here's an example of using the continue statement to skip certain values in an array:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
for number in numbers:
if number % 2 == 0:
continue
print(number)
In the above example, the loop iterates through the numbers array. When it encounters an even number, the if statement is true and the continue statement is executed, causing the loop to skip the current iteration. The output will be:
1
3
5
7
9
Best Practices for using Break and Continue Statements
Here are some best practices for using break
and continue
statements in web development:
- Use them judiciously: While
break
andcontinue
statements can be useful in certain situations, it is important to use them judiciously. Overuse of these statements can make your code difficult to understand and maintain, especially for other developers who may be working on the same codebase. - Always provide a clear condition: When using
break
orcontinue
statements, it is important to provide a clear condition that triggers the statement. This will help to avoid introducing bugs or unexpected behavior in your code. - Keep it simple: When using
break
orcontinue
statements, it is important to keep the code as simple as possible. Avoid using these statements in complicated nested loops or in situations where the code is hard to understand. Simplicity will improve the readability of your code and make it easier to maintain. - Use descriptive variable names: When using
break
orcontinue
statements, it is important to use descriptive variable names. This will make it easier for other developers to understand the code and to make changes if necessary. - Test your code: Before deploying your code, it is important to thoroughly test it to make sure that the
break
andcontinue
statements are working as expected. This will help to avoid introducing bugs or unexpected behavior in your code.
Summary of key points
The article explains the four fundamental data types in Python, which are integer (int), floating-point (float), string (str), and boolean (bool). Variables are used to hold values and are declared using a specific syntax.
The article further discusses the rules for declaring variables in Python and changing their values. The article also explains type conversion and how the int data type can be used in Python for counting, indexing, arithmetic operations, and more. The proper understanding of Python data types and variables is essential for building complicated programs.