Making an HTTP Request from Node.js Using the 'Request' Package
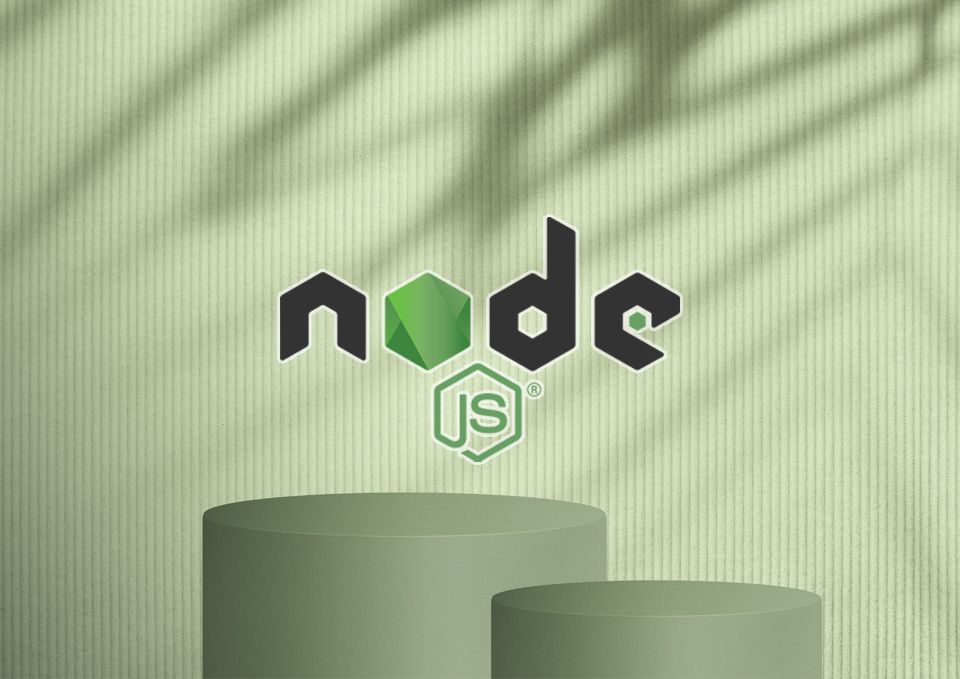
Have you ever struggled with making an HTTP request from Node.js? With the help of the 'Request' package, it's simpler than ever to make requests from your code. In this guide, we'll walk through four steps that show you how to use this package to make any type of request in Node.js.
Steps to Mastering HTTP Requests With Node.js
Install the 'Request' Package in Node.
The first step in making an HTTP request from Node.js is to install the 'Request' package. To do so, use the following command: npm install request --save This will install the 'Request' package and add it as a dependency in your project's package.json file. Once installed, you’re ready for the next step.
example Install the 'Request' Package in Node.js
To install the 'Request' package in Node.js
open a terminal window and type:
npm install request
Define the Request Options and Destination URL.
Now that you have the 'Request' package installed, you must define your request options. This will determine where in the web server your request is sent, as well as what type of request (GET, POST et,c.) it is. This can be done by creating an object with the appropriate values for each possible parameter and setting it to the options variable. Then set the destination URL for your request to a variable called URL.
Example To Define the Request Options and Destination URL.
Request Options:
- HTTP Method (e.g. GET, POST, PUT, DELETE)
- Headers (e.g. Content-Type, Authorization)
- Body (if applicable)
Destination URL:
- The full URL of the API endpoint being
Call the Request Function to Execute the HTTP Request from Node.
After you have successfully set your request options and the URL, you can call the request() function to execute the HTTP request. This is the final step in making a Node.js HTTP request, and it looks like this: request(options, function (error, response, body) { // your code here }); The first parameter of the request() function contains your previously defined options object. The second parameter is a callback function that will be called when the request is completed. Within this callback, you can program what should happen once you receive the response from server.
Example Call the Request Function to Execute the HTTP Request from Node.js
const request = require('request');
request('http://www.example.com', function (error, response, body) {
console.log('error:', error); // Print the error if one occurred
console.log('statusCode:', response && response.statusCode);
}
Handle Response from Server and Output Data Included in the Response Object.
After sending the request, you can use the callback function to determine how the response should be handled. The parameter of this callback contains all the necessary information concerning the received response: In case of an error, it will contain an error message that provides more details about what went wrong. If no errors occurred, data about the server’s response is included in informational fields such as statusCode and headers. Additionally, the body field will contain the server’s actual response if it was successful.
example Handle Response from Server and Output Data Included in the Response :
Object.const response = {
status: 200,
data: [
{name: 'John', age: 30},
{name: 'Jane', age: 25}
]
};
if (response.status === 200) {
console.log('Success!');
response.data.forEach(person => {
console.log(`${person.name} is ${person.age} years old`);
}
}
With this example, the response from the server is displayed on the console. The status code field holds the value 200, which indicates that the request was successful. The data array provided in the response contains two objects containing details about John and Jane's age.
Using a forEach loop it is possible to iterate through the array of persons and display a formatted string on the console containing each person's name and age.
Use Promises to Improve Code Readability and Write a More Scalable Codebase for complex use cases.
Promises enable developers to write cleaner and more concise code, while also helping them accomplish complex logic with ease. With promises, you don't have to wrap your callback function in multiple levels of nested if statements; instead, you can chain together the different operations that influence a request's lifecycle. Integrating Promises into an application gives it the flexibility to handle complex use cases like error handling, rejection of requests, and chaining of requests.
example code Use Promises to Improve Code Readability and Write a More Scalable Codebase for complex use cases.
example code for different method using node.js Using the 'Request' Package.
// Including the request package
var request = require("request");
// Making a request to a web page
request("http://www.example.com", function(error, response, body) {
// Checking for errors
if(error) {
console.log("Something went wrong!");
console.log(error);
} else {
// Checking the status code (200 is HTTP OK)
console.log("The status code was: " + response.statusCode);
// Printing the web page
console.log(body);
}
});
// Making a request to an API
request("https://some-api.com/users", function(error, response, body) {
// Parsing the data as JSON
var data = JSON.parse(body);
// Printing the data
console.log(data);
});
// Making a POST request
// Form data
var formData = {
name: "John Doe",
email: "john@doe.com"
};
// Making a POST request with form data
request.post({url:"http://some-api.com/users", formData: formData}, function(err, httpResponse, body) {
if (err) {
return console.error('upload failed:', err);
}
console.log('Upload successful! Server responded with:', body);
});
Steps to Mastering HTTP Requests With Node.js
Finish.