5 Steps to Mastering HTTP Requests With Node.js
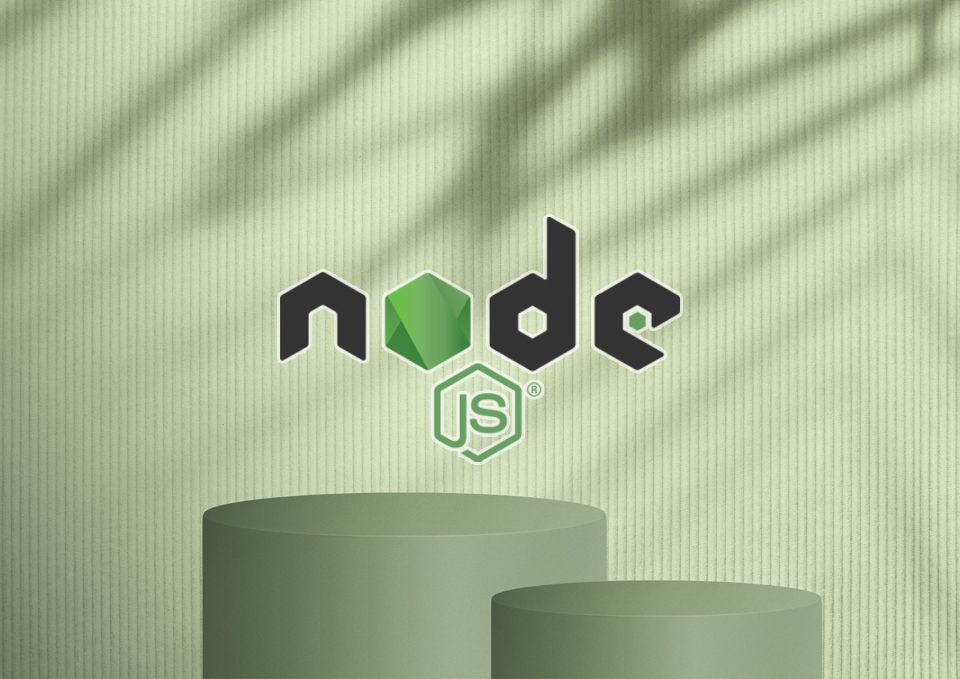
Node.js is a powerful tool for making HTTP requests over the internet. In this five-step tutorial, you'll learn how to use Node.js to make efficient and effective requests with code examples you can use in your own projects. Get ready to take your Node.js techniques to the next level!
Understand HTTP Fundamentals.
Before you dive into coding your HTTP request, make sure you understand the fundamentals of HTTP. This protocol underlies virtually every website and web application and is essential to making Node.js requests. Learn which headers and methods are used when requesting information from a server, as well as how to structure the URI or URL string with query parameters. Knowing these basics will help you create effective requests faster!
Step 1: Installing Node Packages for Making HTTP Requests
To get started making HTTP requests from Node.js, you'll need to install some packages first. Examples of http request node.js packages include :
Step 2: Learn the fundamentals of HTTP requests.
In order to be familiar with HTTP requests, it is important to know their basics. The structure of a request comprises of different components that should be taken into consideration. Moreover, grasping the various HTTP methods and status codes is equally essential.
Step 3: of developing a Node.js application involves understanding the Node.js request library.
Node.js provides a library that simplifies making HTTP requests. This library provides an easy-to-use interface for making requests and managing responses. The request library supports all major HTTP methods, including GET, POST, PUT, DELETE, HEAD, and OPTIONS. It also supports HTTPS and automatic redirects, as well as proxy support. This library is ideal for making simple HTTP requests in a Node.js application and it is popular among developers due to its ease of use.
Ready to practice making HTTP requests with Node.js?
Step 4 is all about honing your skills! You can do this using the sample APIs available or by creating your own. Once you feel comfortable, you'll be ready to move on to more complex operations.
Example POST, PUT, and DELETE HTTP requests using Node.js
Node.js provides an efficient way to make POST, PUT, and DELETE HTTP requests with simple code. Here’s how: For instance, sending a POST request is easily done with the Node.js request module command:
var request = require('request');
var options = {
url: 'http://www.example.com/some/endpoint',
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
some: 'data'
})
};
request(options, function (err, res, body) {
if (err) {
console.log(err);
} else {
console.log(body);
}
});
var request = require('request');
var options = {
url: 'http://www.example.com/some/endpoint',
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
some: 'data'
})
};
request(options, function (err, res, body) {
if (err) {
console.log(err);
} else {
console.log(body);
}
});
var request = require('request');
var options = {
url: 'http://www.example.com/some/endpoint',
method: 'DELETE',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({
some: 'data'
})
};
request(options, function (err, res, body) {
if (err) {
console.log(err);
} else {
console.log(body);
}
});
Step 5: of using Node.js is to utilize the request library for more complicated requests.
The library allows you to make POST, PUT, DELETE, and HEAD requests easily and quickly, so you can tailor your code for the specific needs of your project. With some practice, you'll be able to apply these methods with confidence!
finished