8 Step Fixing React Query Errors in React JS
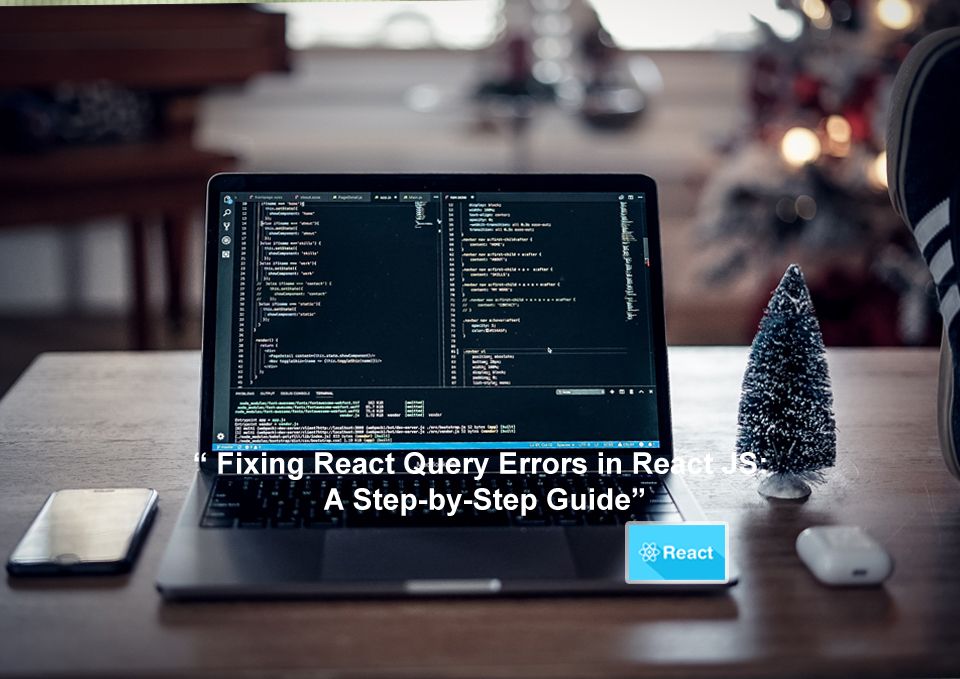
8 Step Fixing React Query Errors in React JS. Error handling is a key task in handling data fetching. React Query helps us take asynchronous data with maximum flexibility. It provides various hooks for creating read updates and deleting data from the database.
React Query is a great module that makes error handling really easy by returning an error prop.
This tutorial will teach you how to use the useQuery hook to make Get requests with React queries. The most important thing is for you to understand how to easily handle error responses after a get request to the server.
React Query provides an error object containing the error message; we'll use the Bootstrap alert UI component to display error messages in our React application.
How to display error messages in React JS using React Query
- Step 1: Create React Project
- Step 2: Install React Query and Axios Modules
- Step 3: Setting Up Bootstrap
- Step 4: Register React-Query in React
- Step 5: Build Function Component
- Step 6: Handle Error Response
- Step 7: Update the Global Component
- Step 8: View App on Browser
Create React Project
You need to run the command in the terminal to create a brand-new React project.
npx create-react-app react-blog-app
After creating the application, move it to the project folder.
cd react-blog-app
Install React Query and Axios Modules
This project needs to add Axios and react-query libraries to the React project.
Run the command with npm to install the module.
npm install @tanstack/react-query Axios
Setting Up Bootstrap
We will install and use the Bootstrap library to style our React application.
npm i bootstrap
This work is not done yet, you must register the bootstrap CSS in the src/App.js
file.
import "../node_modules/bootstrap/dist/css/bootstrap.min.css";
function App() {
return <div></div>;
}
export default App;
Register React-Query in React
React Query is installed, but to take full advantage of the library you must import the QueryClientProvider and the QueryClient module in your App.js
file.
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import { QueryClient, QueryClientProvider } from "@tanstack/react-query";
// Create a client
const queryClient = new QueryClient();
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<QueryClientProvider client={queryClient}>
<App />
</QueryClientProvider>
);
Build Function Component
Go to the src folder in the src directory, create a components/
folder and create a Users.js
file.
import React from 'react'
function Users() {
return (
<div>Users page</div>
)
}
export default Users
Handle Error Response
Now that you've created the Users functional component, we'll show you how to use the useQuery hook to make a get request while handling errors and other responses.
Make sure to add the following code to your components/Users.js
file.
import React from "react";
import axios from "axios";
import { useQuery } from "@tanstack/react-query";
async function fetchData() {
const { data } = await axios.get(
"https://jsonplaceholder.typicode.com/users1"
);
return data;
}
function Users() {
const { isLoading, isError, error, data } = useQuery(["users"], fetchData);
if (isLoading) {
return <div className="alert alert-warning">Retrieving Data ...</div>;
}
if (isError) {
return (
<div>
<div className="alert alert-danger">{error.message}</div>
</div>
);
}
return (
<ul className="list-group">
{data.map((res, index) => {
return (
<li className="list-group-item mb-3" key={index}>
<h3>{res.username}</h3>
<p>{res.email}</p>
</li>
);
})}
</ul>
);
}
export default Users;
Update Global Component
We are almost done with function components and React queries, the final task is to add the Users component to the App.js
file.import React from react;
import React from "react";
import "../node_modules/bootstrap/dist/css/bootstrap.min.css";
import Users from "./components/Users";
function App() {
return (
<div className="container mt-5">
<h2 className="mb-4">React Query Error Handling Example</h2>
<Users />
</div>
);
}
export default App;
View App on Browser
Our application is almost ready, the next step is to call the React development server with the following command.
npm start
You need to paste the given URL on your browser's address bar to view the application on your browser.
http://localhost:3000
Conclusion
In this tutorial, we learned how to fetch error messages, in contrast, to React query fetch requests. We saw how to use the isError and error properties returned by the useQuery hook.
The Error object provides an easy mechanism to handle errors in React applications driven by React queries. A response is always expected after a request to the server; it may be a success or an error.
If something goes wrong somehow. We can take advantage of the error property and display an error message to the user.