Easily Measure Your Internet Connection Speed with React JS
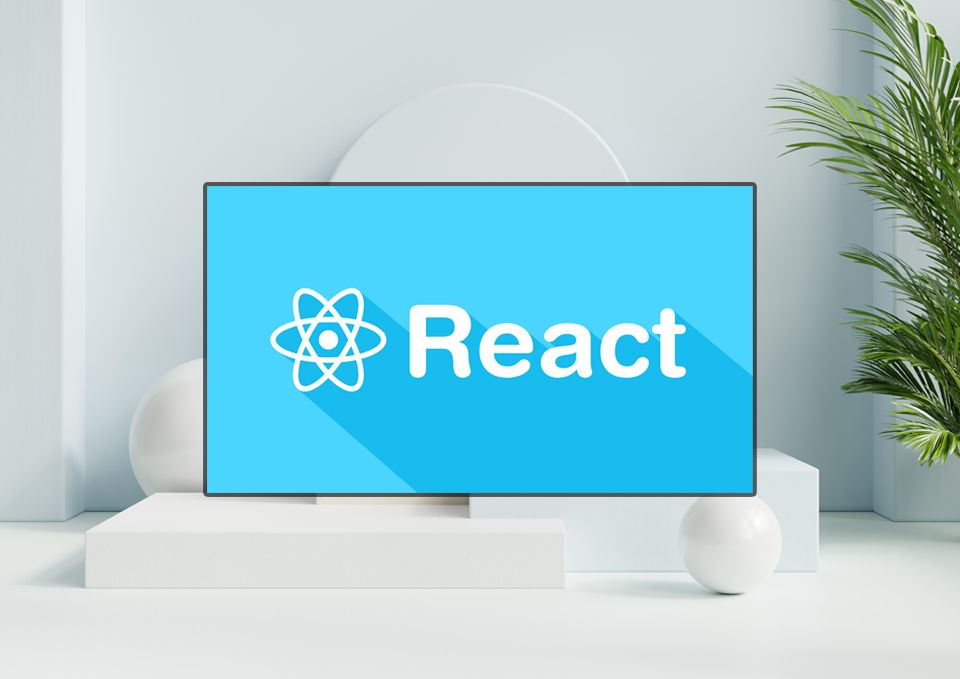
Are you finding it difficult to measure the speed of your internet connection? With this guide, you can learn how to quickly create a React JS component that can easily measure your internet connection's speed. Get all the tips and resources needed to start building now!
How to Create a New React App
Are you ready to create a new React App? If so, you'll need to first make sure that you have Node and npm installed. Once those are in place, you can run the following command to get started:
npx create-react-app my-app
This will generate the basic folder structure for your application and allow you to start building!.
next task is to move inside the app.
cd my-app
React.js: Building Functional Components
To create a functional component with React.js, the first step is to make the components/ folder. Then add a ConnectionSpeed.js file inside the folder which will contain the code for your new functional component. This code is just a regular JavaScript function that returns React elements to be used in the rest of your application.
import React from 'react'
function Profile() {
return (
<div>ConnectionSpeed page</div>
)
}
export default ConnectionSpeed
Integrating a React Internet Meter Model with Your Web Application
If you're looking to integrate a React Internet Meter Model with your web application, then you'll need to add a third-party library. The command for doing this is simple - just run the following code to add the React Internet Meter library within React.
npm install react-internet-meter
Using Bootstrap with React
Are you looking to make use of Bootstrap in a React project? Fortunately, the installation of a Bootstrap package is not a necessity to be able to accomplish this. By adding this module, we are able to structuralize our basic layout using React.
npm i bootstrap
To use Bootstrap with React, open the src/App.js component and import the Bootstrap CSS into the file.
import "../node_modules/bootstrap/dist/css/bootstrap.min.css";
function App() {
return <div></div>;
}
export default App;
Create Your Own Internet Speed Meter
To create your own internet speed meter in React, you will need to first import the ReactInternetSpeedMeter component from the “react-internet-meter” package. This generic component can be customised with various properties, allowing you to determine the internet speed. Finally, place the code into the components/ConnectionSpeed.js file.
import React from "react";
import "../../node_modules/react-internet-meter/dist/index.css";
import { ReactInternetSpeedMeter } from "react-internet-meter";
function ConnectionSpeed() {
const [checkSpeed, SetCheckSpeed] = React.useState(
"Finding internet speed."
);
return (
<div>
<h2 className="mb-3">React Find Internet Speed Example</h2>
<ReactInternetSpeedMeter
txtSubHeading="Internet connection is slow"
outputType="" // "alert"/"modal"/"empty"
customClassName={null}
pingInterval={2000} // milliseconds
txtMainHeading="Opps..."
thresholdUnit="megabyte" // "byte" , "kilobyte", "megabyte"
threshold={50}
imageUrl="https://i.postimg.cc/sft772VP/speedometer.png"
downloadSize="1561257" //bytes
callbackFunctionOnNetworkDown={(data) =>
console.log(`Internet speed : ${data}`)
}
callbackFunctionOnNetworkTest={(data) => SetCheckSpeed(data)}
/>
<div className="card-body mt-4">
<span className="display-1">{checkSpeed} MB/s</span>
</div>
</div>
);
}
export default ConnectionSpeed;
Creating and Registering a React Component
Now that the React component has been written, it's time to add it to your application! To do this, open up the App.js file and import the newly-created component into the file.
import ConnectionSpeed from "./components/ConnectionSpeed";
import "../node_modules/bootstrap/dist/css/bootstrap.min.css";
import "./App.css";
function App() {
return (
<div className="container">
<ConnectionSpeed />
</div>
);
}
export default App;
Start React Application
Building a React application starts with installing the necessary packages. Once you have set up your environment, you can use the command line to start an application. After running this command, your browser will take over and your application will be ready to go, provided that you are connected to the internet.
npm start
http://localhost:3000
Finish.