Best Practices for Caching in React JS with React Query
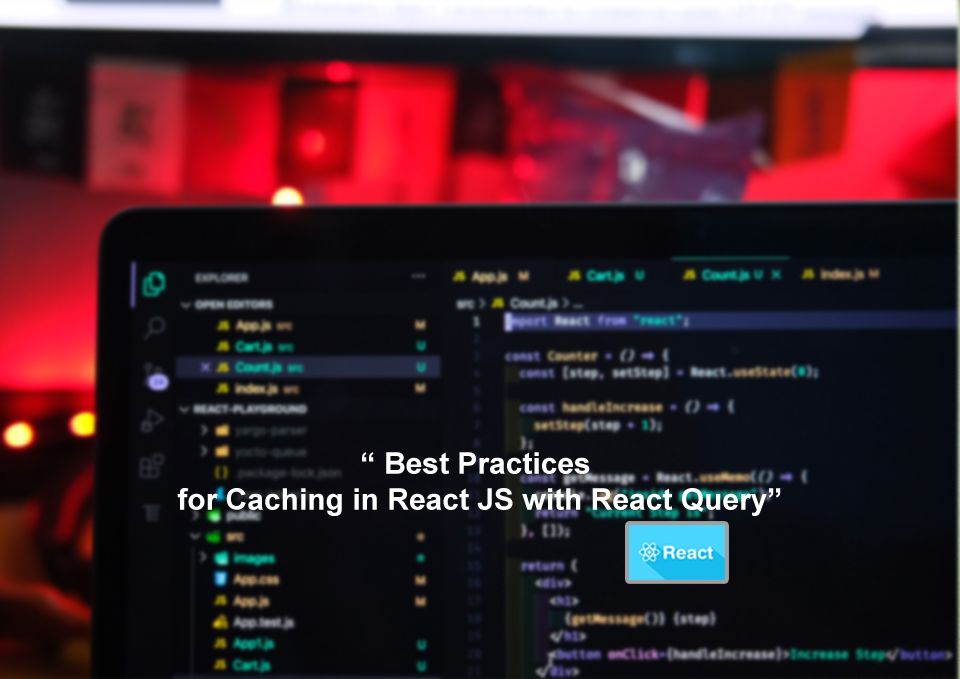
Caching in React JS with React Query. In this tutorial, we will learn how to create a basic React application and communicate with a server to fetch and cache data using React Query.
React is an excellent framework. However, it also has some disadvantages. React applications don't provide an opinionated way of getting or updating data from components; therefore, developers end up generating their own methods of getting data.
Ideally, this means piecing together component-based state and effects using React hooks or using a more general state management library to store and serve data asynchronously throughout the application.
There are tons of good state management libraries out there. However, they are not up to the mark when it comes to managing async or server state.
In general, managing the server state is cumbersome. Once you get your hands on the server state, some challengers will automatically appear:
- Data Caching
- Getting to know when data gets old
- Updating old data in the background
- Updating the latest changes in the data
- Shared memory query results through structures
- Manage memory with server state garbage pool
- Performance optimization for data paging and lazy loading
- Deduplication of multiple requests for the same data with a single request
We have discussed the advantages and benefits of using React Query with React.
Now let's look at the useQuery hook:
React Query is a server state package for managing asynchronous operations between server and client. The useQuery hook is available through the react-query library.
The useQuery hook is a simple yet powerful feature primarily for implementing data fetching code in contrast to the React query module.
How to fetch and cache data with React Query in React using the useQuery Hook
- Build React Project
- Install Axios and React queries
- Set Up Bootstrap
- Configure React-Query in React
- Create Function Component
- Get data using useQuery Hook
- Update App JS Component
- Test App on Browser
Build React Project
Let's start by building a new React project with the given commands.
npx create-react-app react-blog-app
Moved to the application folder as soon as the application is built.
cd react-blog-app
Install Axios & React Query
To handle fetch requests and fetch request management install Axios and react-query libraries.
npm install @tanstack/react-query axios
Set Up Bootstrap
We will install the bootstrap CSS library in our project using the command.
npm i bootstrap
To make bootstrap fully work in React Add the module CSS in the src/App.js
file.
import "../node_modules/bootstrap/dist/css/bootstrap.min.css";
function App() {
return <div></div>;
}
export default App;
Configure React-Query in React
We will add React-Query to our React application. You can do this by wrapping the App.js
component with QueryClientProvider and QueryClient from the react-query package.
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import { QueryClient, QueryClientProvider } from "@tanstack/react-query";
// Create a client
const queryClient = new QueryClient();
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<QueryClientProvider client={queryClient}>
<App />
</QueryClientProvider>
);
It will grant us access to all hooks in the react-query module.
Create Function Component
We now need to create the components/
folder and the Users.js
file.
import React from 'react'
function Users() {
return (
<div>Users page</div>
)
}
export default Users
Fetch Data with useQuery Hook
To manage the fetch data request state, put the following code in the components/Users.js
file.
import React from "react";
import axios from "axios";
import { useQuery } from "@tanstack/react-query";
async function fetchData() {
const { data } = await axios.get(
"https://jsonplaceholder.typicode.com/users"
);
return data;
}
function Users() {
const { isLoading, isError, error, data } = useQuery(["users"], fetchData);
if (isLoading) {
return <div>Data is loading...</div>;
}
if (isError) {
return <div>Error! {error.message}</div>;
}
return (
<ul className="list-group">
{data.map((res, i) => {
return (
<li className="list-group-item mb-3" key={i}>
<h3>{res.username}</h3>
<p>{res.email}</p>
</li>
);
})}
</ul>
);
}
export default Users;
Update App JS Component
The next step is to add the Users component to the App.js
file; this will make the Users component available in our React application.
import React from "react";
import "../node_modules/bootstrap/dist/css/bootstrap.min.css";
import Users from "./components/Users";
function App() {
return (
<div className="container mt-5">
<h2>React Query Fetch State Management Example</h2>
<Users />
</div>
);
}
export default App;
Test App on Browser
We finally reached the last step of this post; just open the console and execute the given command.
npm start
Once the server is running, test the application on a browser using the URL below.
http://localhost:3000
Conclusion
React Query is a great tool, but that doesn't mean you shouldn't try other options. Redux is also another powerful and convenient option.
This is the ultimate panacea for web developers; in order to master in managing the global state, you must try React Query and Redux together.
In this article, we saw how to set up React queries in a React application. How to use the useQuery hook to make an asynchronous call to the server to fetch data.
Not only that but also how to effortlessly manage useQuery hook responses that include loading isError error data and success.