How to setup page 404 in React Using React Router
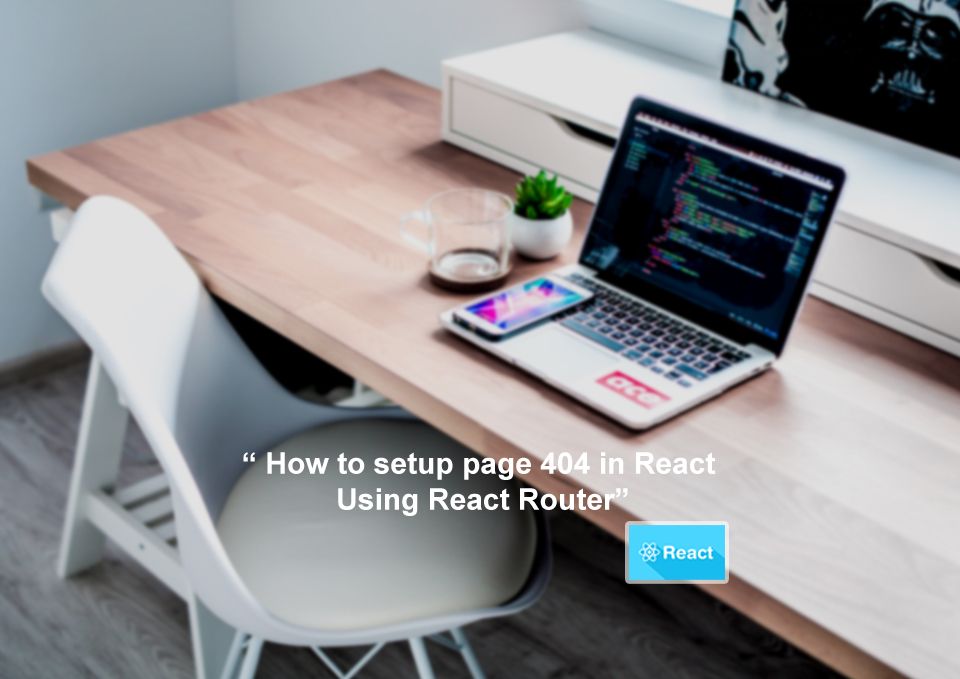
404 is the status code that appears when accessing a web page that does not exist. Ideally, the user is redirected to a custom 404 page that notifies that the requested page is no longer available.
If you want to set a custom 404-page template in React. Then this guide is for you.
In this tutorial, you will learn how to use the React Router DOM v6 package to build a custom 404-page template in a React JS application.
This comprehensive guide will help you analyze and evaluate: How to properly install and set up the router dom library in React. How to create a React router dom 404 page template in React. How to resolve 404 errors in React using the router dom API. How to make a 404 route component and redirect to Use the Astrix * symbol of returning a 404 page when the requested page does not exist.
Create React Environment
The first step is to install or add node and npm to your development machine.
We can use the packages above to create react applications; this is the command that needs to be invoked.
npx create-react-app react-blog
//next
cd react-blog
Set Up Router DOM in React
You need to install the react-router library to enable navigation routing in your React application without any hassle.
npm i react-router-dom
Go to the src/index.js
file; you need to import and wrap your application components with the BrowserRouter module.import react from react; import ReactDOM from react-dom/client.
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App";
import "./index.css";
import { BrowserRouter } from "react-router-dom";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<BrowserRouter>
<App />
</BrowserRouter>
</React.StrictMode>
);
Create Function Components
After completing the previous step, we need a few functional components to create the no-page found route.
First create a components/
folder and a Home.js
the file inside the folder.
import React from 'react'
function Home() {
return (
<div>Home page</div>
)
}
export default Home
Next create a components/ folder and a Contact.js file in the directory.
import React from 'react'
function Contact() {
return (
<div>Contact page</div>
)
}
export default Contact
Finally build the components/
directory and Template404.js
file with the given code.
import React from 'react'
function Template404() {
return (
<div>Template404 page</div>
)
}
export default Template404
Create React 404 Template Component
You will need the Routes Route and Link modules to set up routing in React.
You also need to import the components that help us create our functionality.
Use the Link component to set up routing, and when accessing a broken url, the * sign redirects to the page and does not find the component.
Update the code into the src/App.js
file:
import { Routes, Route, Link } from "react-router-dom";
import Template404 from "./components/Template404";
import Contact from "./components/Contact";
import Home from "./components/Home";
function App() {
return (
<div>
<h2>React Custom 404 Template Page Example</h2>
<div>
<Link to="/">Home</Link>
</div>
<div>
<Link to="/contact">Contact</Link>
</div>
<div>
<Link to="/page-not-found">Obselete Route</Link>
</div>
<Routes>
<Route path="/" element={<Home />} />
<Route path="/profile" element={<Contact />} />
<Route path="*" element={<Template404 />} />
</Routes>
</div>
);
}
export default App;
Test React Application
The npm start command instructs the React application server to invoke and open the application on the browser.
npm start
http://localhost:3000