How to Play Mp3 Sound with ReactJS
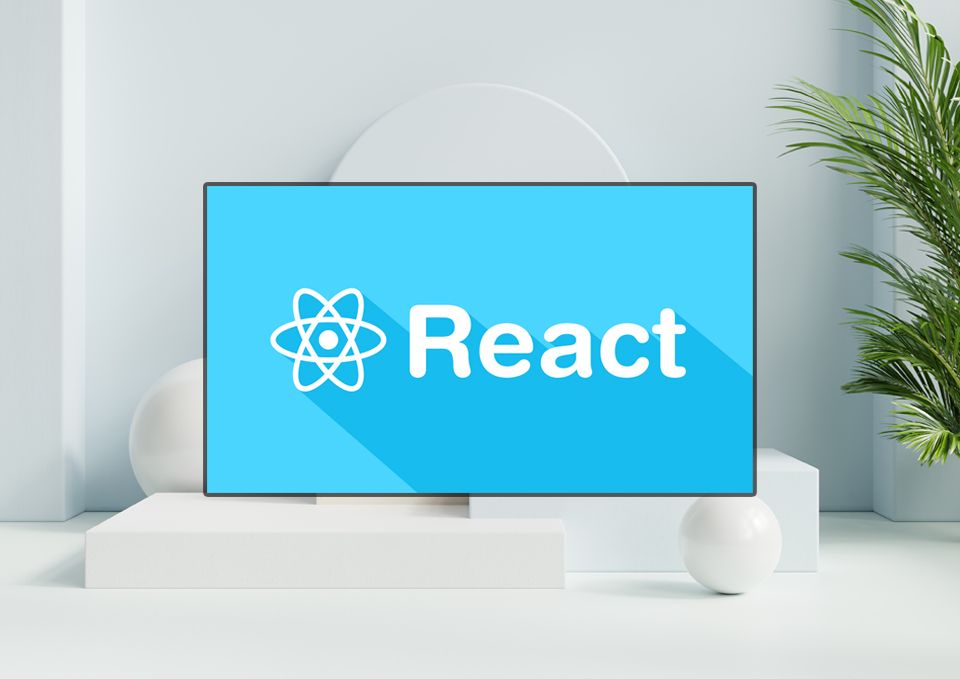
Learn how to create captivating audio experiences with ReactJs in this tutorial. We will explore the basics of React and how it can be used to play mp3 sounds in apps. From creating components, and managing state to utilizing props, you will gain a deep understanding of the fundamentals of React and how to use it to create engaging audio experiences.
Introduction to the React Sound Player.
We will begin by creating a sound player component using the React Sound Player library. This library allows us to load our mp3 audio file and play it in the app with ease. We will be using some of the useful features the library provides such as the ability to control playback speed, set a loop on or off, change volume, create multiple sound players, and more.
Example Import react sound player library and function play and stop
import React from 'react';
import Sound from 'react-sound';
class AudioPlayer extends React.Component {
constructor(props) {
super(props);
this.state = {
playStatus: Sound.status.STOPPED
}
this.handleToggle = this.handleToggle.bind(this);
}
handleToggle(status) {
if (status === Sound.status.PLAYING) {
this.setState({ playStatus: Sound.status.PAUSED });
} else {
this.setState({ playStatus: Sound.status.PLAYING });
}
}
render() {
return (
<div>
<Sound
url={this.props.url}
playStatus={this.state.playStatus}
onToggle={this.handleToggle}
/>
<button onClick={() => this.handleToggle(this.state.playStatus)}>
{this.state.playStatus === Sound.status.PLAYING ? 'Pause' : 'Play'}
</button>
</div>
);
}
}
export default AudioPlayer;
Setting up and Creating a Functional SoundPlayer Component.
To get started, we will create a functional SoundPlayer component with ReactJS. We need to install the React Sound Player library for this. Let’s begin by importing the useInSound hook from the library which allows us to access functions such as setPlaybackSpeed, setLoop and so on.
Then, we’ll create a ref which can be used to interact with our audio element dynamically. Lastly, we need to define our onPlay and onPause functions so that when triggered the sound in our app can play or pause respectively. code Creating a Functional SoundPlayer Component.
- Create a class called SoundPlayer that extends the Component class.
- Add a constructor to the SoundPlayer class that takes in a source URL for the sound file as an argument.
- Add a method to the SoundPlayer class called playSound() which will be used to play the sound file from the given source URL.
- Add a method to the Sound
Example point number 01:
public class SoundPlayer extends Component{
private String soundFile;
public SoundPlayer(String soundFile){
this.soundFile = soundFile;
}
public void play(){
System.out.println("Playing sound file: " + soundFile);
}
public void pause(){
System.out.println("Pausing sound file: " + soundFile);
}
public void stop(){
System.out.println("Stopping sound file: " + soundFile);
}
}
Example point number 02:
public class SoundPlayer {
private String sourceUrl;
public SoundPlayer(String sourceUrl) {
this.sourceUrl = sourceUrl;
}
// other methods
}
Example point number 03:
public class SoundPlayer {
private String sourceUrl;
public SoundPlayer(String sourceUrl) {
this.sourceUrl = sourceUrl;
}
public void playSound() {
try {
URL url = new URL(sourceUrl);
AudioInputStream audioIn = AudioSystem.getAudioInputStream(url);
Clip clip = AudioSystem.getClip();
clip.open(audioIn);
clip.start();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Example point number 04:
Sound.prototype.getSound = function() {
return this.sound;
}
Importing Audio Files into React Projects.
Once the necessary components have been created and functions defined, we can start to import audio files into our React project. To do this, we need to define a new variable called audioFile and use the React createRef() method to store the path of our mp3 file within it. With this ref in place, we can now attach it to the sound player by passing audioFile into props as shown below.
const audioFile = React.createRef();
class App extends React.Component {
render() {
return (
<div>
<SoundPlayer audioFile={audioFile} />
</div>
);
}
}
Adding Play, Stop, and Pause Controls for Your MP3s.
To make your sound player interactive and customizable, you can add play, pause and stop buttons to it. Start by creating a new function for each button in the imported app.js file paired with an appropriate event listener. Within the functions, define an if statement that will detect any changes in audioFile props and trigger the actions accordingly depending on whether it's playing or stopped. Finally, add three new buttons to the render() method with corresponding labels or icons.
//app.js
import React from 'react';
class App extends React.Component {
constructor(props){
super(props);
this.state = {
audioFile: null
};
}
// Define functions for play, pause and stop buttons
playButton = () => {
if(this.state.audioFile.paused) {
this.state.audioFile.play();
}
}
pauseButton = () => {
if(this.state.audioFile.played) {
this.state.audioFile.pause();
}
}
stopButton = () => {
if(this.state.audioFile.played) {
this.state.audioFile.pause();
this.state.audioFile.currentTime = 0;
}
}
render(){
return (
<div>
// Add play, pause and stop buttons
<button onClick={this.playButton}>Play</button>
<button onClick={this.pauseButton}>Pause</button>
<button onClick={this.stopButton}>Stop</button>
</div>
);
}
}
export default App;
Uploading Sounds from External Sources Using Data URIs.
If you would like to add sounds from external sources, such as audio streaming services, a better approach is using Data URIs. To do this, start by creating an XMLHttpRequest object that will allow you to access the sound file through its URL in JSON format. Then use the FileReader() method to convert the response into a Data URI which can be used with React's player component. Finally set the newly created Data URI in the source attribute of player and you'll be ready playback your sound.
import React, { useState } from 'react';
function App() {
const [dataURI, setDataURI] = useState(null);
const fetchSound = () => {
const url = "http://example.com/sound";
const xhr = new XMLHttpRequest();
xhr.responseType = 'json';
xhr.onreadystatechange = () => {
if (xhr.readyState === XMLHttpRequest.DONE) {
const reader = new FileReader();
reader.onload = (e) => {
setDataURI(e.target.result);
}
reader.readAsDataURL(xhr.response);
}
}
xhr.open('GET', url);
xhr.send();
}
return (
<div>
{dataURI ? (
<audio src={dataURI} controls />
) : (
<button onClick={fetchSound}>Fetch sound</button>
)}
</div>
);
}
export default App;
Finish.